What is an IoT Button?
An IoT button is a simple device that can trigger actions or events through the internet. It consists of a physical button connected to a microcontroller, such as the ESP8266, which is programmed to send a signal to a web service or application when the button is pressed. This signal can then be used to initiate a wide range of actions, from sending notifications to controlling smart home devices.
Types of IoT Buttons
There are several types of IoT buttons available, each with its own unique features and capabilities:
Type | Description |
---|---|
Simple Button | A basic button that sends a single signal when pressed |
Multi-Function Button | A button that can send different signals based on the number of presses or the duration of the press |
Wireless Button | A button that communicates wirelessly with other devices or services |
Programmable Button | A button that can be programmed to perform specific actions or trigger custom events |
Why Use an ESP8266 for an IoT Button?
The ESP8266 is an ideal choice for creating an IoT button due to its many advantages:
- Low Cost: The ESP8266 is incredibly affordable, with prices starting at just a few dollars.
- Small Size: The ESP8266 is tiny, measuring just 5mm x 5mm, making it easy to integrate into small devices like buttons.
- Wi-Fi Connectivity: The ESP8266 has built-in Wi-Fi, allowing it to connect to the internet and communicate with web services and applications.
- Low Power Consumption: The ESP8266 is designed for low power consumption, making it suitable for battery-powered devices.
- Easy to Program: The ESP8266 can be programmed using the Arduino IDE, which is familiar to many makers and hobbyists.
Comparison of ESP8266 with Other Microcontrollers
While the ESP8266 is a popular choice for IoT projects, there are other microcontrollers available that may be suitable depending on your specific needs:
Microcontroller | Advantages | Disadvantages |
---|---|---|
ESP8266 | Low cost, small size, Wi-Fi connectivity, low power consumption, easy to program | Limited processing power and memory compared to more powerful microcontrollers |
Arduino | Wide range of boards available, extensive library support, large community | Requires additional components for Wi-Fi connectivity, higher power consumption than ESP8266 |
Raspberry Pi | High processing power, runs full Linux operating system, extensive library support | Higher cost than ESP8266, larger size, higher power consumption |
Creating an ESP8266 IFTTT Button
Now that we understand the basics of IoT buttons and the advantages of using an ESP8266, let’s dive into the process of creating an ESP8266 IFTTT button.
Required Components
To create an ESP8266 IFTTT button, you will need the following components:
- ESP8266 microcontroller
- Breadboard
- Jumper wires
- Tactile button
- USB cable for programming
- Computer with Arduino IDE installed
Circuit diagram
Here is a simple circuit diagram for an ESP8266 IFTTT button:
+-----+
| |
| +--+--+
| | |
| | B |
| | |
| +--+--+
| |
+----+ +--+--+ |
| | | | |
| U | | E | |
| S | | S | |
| B +-----+ P | |
| | | 8 | |
+----+ | 2 | |
| 6 | |
| 6 | |
+--+--+ |
| |
| |
+-----+
In this diagram, the tactile button is connected to one of the GPIO pins on the ESP8266, and the other end is connected to ground. When the button is pressed, it will pull the GPIO pin low, which can be detected in the code.
Programming the ESP8266
To program the ESP8266 to function as an IFTTT button, you will need to use the Arduino IDE and install the necessary libraries for the ESP8266. Here is a step-by-step guide:
- Install the Arduino IDE on your computer.
- Add the ESP8266 board manager to the Arduino IDE by going to File > Preferences and adding the following URL to the “Additional Boards Manager URLs” field:
http://arduino.esp8266.com/stable/package_esp8266com_index.json
- Go to Tools > Board > Boards Manager and search for “esp8266”. Install the latest version of the ESP8266 package.
- Select the appropriate ESP8266 board from the Tools > Board menu.
- Connect the ESP8266 to your computer using a USB cable.
- Open a new sketch in the Arduino IDE and copy the following code:
#include <ESP8266WiFi.h>
#include <WiFiClientSecure.h>
const char* ssid = "your_wifi_ssid";
const char* password = "your_wifi_password";
const char* host = "maker.ifttt.com";
const char* apiKey = "your_ifttt_api_key";
const int buttonPin = 2;
void setup() {
Serial.begin(115200);
pinMode(buttonPin, INPUT_PULLUP);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
}
void loop() {
if (digitalRead(buttonPin) == LOW) {
WiFiClientSecure client;
client.setInsecure();
if (!client.connect(host, 443)) {
Serial.println("connection failed");
return;
}
String url = "/trigger/button_pressed/with/key/";
url += apiKey;
client.print(String("GET ") + url + " HTTP/1.1\r\n" +
"Host: " + host + "\r\n" +
"Connection: close\r\n\r\n");
while (client.connected()) {
String line = client.readStringUntil('\n');
if (line == "\r") {
Serial.println("IFTTT request sent");
break;
}
}
client.stop();
delay(5000);
}
}
- Replace
your_wifi_ssid
,your_wifi_password
, andyour_ifttt_api_key
with your actual Wi-Fi credentials and IFTTT API key. - Upload the sketch to the ESP8266 by clicking the “Upload” button in the Arduino IDE.
Setting Up IFTTT
To complete the setup of your ESP8266 IFTTT button, you will need to create an IFTTT applet that will be triggered when the button is pressed. Here’s how to do it:
- Sign up for an IFTTT account if you don’t already have one.
- Click on “Create” to create a new applet.
- Click on “Add” next to “If This” and search for “Webhooks”. Select the “Receive a web request” trigger.
- Enter an event name, such as “button_pressed”, and click “Create trigger”.
- Click on “Add” next to “Then That” and choose the action service you want to use, such as sending an email, posting a tweet, or controlling a smart home device.
- Configure the action as desired and click “Create action”.
- Review your applet and click “Finish”.
Your ESP8266 IFTTT button is now set up and ready to use!
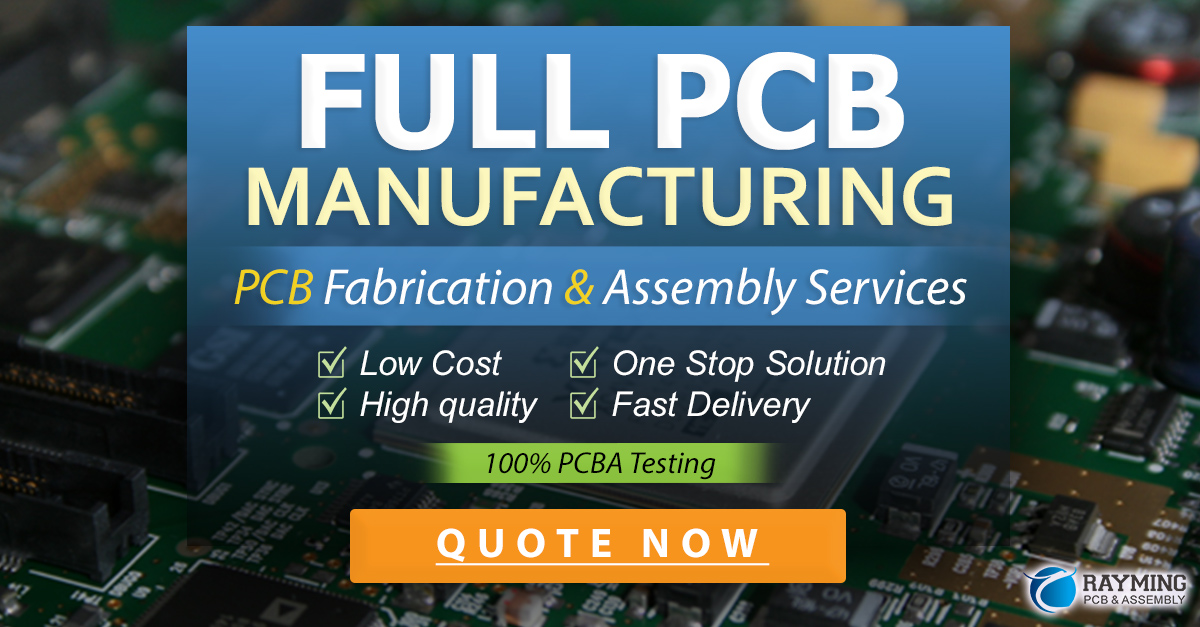
Applications of an ESP8266 IFTTT Button
An ESP8266 IFTTT button can be used for a wide range of applications, limited only by your imagination. Here are a few examples:
Smart Home Control
An ESP8266 IFTTT button can be used to control various smart home devices, such as lights, thermostats, and appliances. For example, you could create an IFTTT applet that turns on your smart lights when the button is pressed.
Notifications
You can use an ESP8266 IFTTT button to send notifications to your phone or email when certain events occur. For example, you could set up a button in your mailbox that sends you a notification when mail is delivered.
Automation
An ESP8266 IFTTT button can be used to automate repetitive tasks. For example, you could create a button that automatically orders your favorite pizza when pressed.
Emergency Alerts
In case of emergencies, an ESP8266 IFTTT button can be used to send alerts to family members or emergency services. For example, you could create a button that sends a text message to your family members when pressed.
FAQ
-
Q: Can I use other microcontrollers besides the ESP8266 to create an IFTTT button?
A: Yes, you can use other microcontrollers that have Wi-Fi connectivity, such as the Arduino MKR1000 or the Particle Photon. -
Q: How many actions can I trigger with one button press?
A: With IFTTT, you can create multiple applets that are triggered by the same event, so you can trigger as many actions as you want with a single button press. -
Q: Can I use the ESP8266 IFTTT button without an internet connection?
A: No, the ESP8266 IFTTT button requires an internet connection to communicate with the IFTTT service. -
Q: How long does the battery last on an ESP8266 IFTTT button?
A: The battery life depends on factors such as the size of the battery, the frequency of button presses, and the power consumption of the ESP8266. With proper power management techniques, an ESP8266 IFTTT button can last for several months on a single battery charge. -
Q: Can I use the ESP8266 IFTTT button outdoors?
A: Yes, but you will need to ensure that the button is properly protected from the elements, such as by using a waterproof enclosure.
Conclusion
The ESP8266 IFTTT button is a simple yet powerful device that can be used to trigger a wide range of actions and events through the internet. By combining the low-cost and ease of use of the ESP8266 with the versatility of IFTTT, makers and hobbyists can create custom IoT Solutions that are tailored to their specific needs.
Whether you want to control your smart home devices, send notifications, automate tasks, or create emergency alerts, an ESP8266 IFTTT button can help you achieve your goals. With a little creativity and programming knowledge, the possibilities are endless.
Leave a Reply