Introduction to Raspberry Pi Voice Recognition
The Raspberry Pi is a versatile single-board computer that has revolutionized the world of DIY projects and hobbyist electronics. One of the many exciting applications of the Raspberry Pi is voice recognition, which allows users to interact with their devices using spoken commands. In this article, we will explore the basics of Raspberry Pi voice recognition and guide you through an easy voice recognition project that you can build at home.
What is Voice Recognition?
Voice recognition, also known as speech recognition, is the process of converting spoken words into text or commands that a computer can understand and execute. It involves the use of algorithms and machine learning techniques to analyze the sound waves of human speech and match them to predefined patterns or templates.
Benefits of Voice Recognition
Voice recognition technology offers several benefits, including:
- Hands-free interaction: Voice recognition allows users to interact with their devices without the need for physical input, such as typing or clicking.
- Accessibility: Voice recognition can be particularly useful for people with disabilities who may have difficulty using traditional input methods.
- Efficiency: Speaking commands can often be faster and more convenient than typing or navigating menus.
- Natural interaction: Voice recognition provides a more natural and intuitive way of interacting with technology, making it easier for users to adapt to new devices and applications.
Components Required for Raspberry Pi Voice Recognition Project
To build a Raspberry Pi voice recognition project, you will need the following components:
Component | Description |
---|---|
Raspberry Pi | A single-board computer that will serve as the brain of your voice recognition project. Any model of Raspberry Pi can be used, but we recommend using a Raspberry Pi 3 or newer for optimal performance. |
Microphone | A USB microphone or a compatible audio input device that will capture your voice commands. |
Speaker | A speaker or headphones that will provide audio output for your Raspberry Pi. |
Power Supply | A power supply unit (PSU) that will provide power to your Raspberry Pi. You can use the official Raspberry Pi PSU or any compatible 5V power supply. |
microSD Card | A microSD card with at least 8GB of storage capacity to install the Raspberry Pi operating system and store your project files. |
Optional Components
- USB Keyboard and Mouse: If you plan to interact with your Raspberry Pi directly, you may need a USB keyboard and mouse for initial setup and configuration.
- HDMI Cable: If you want to connect your Raspberry Pi to a monitor or TV for visual output, you will need an HDMI cable.
Setting Up Your Raspberry Pi for Voice Recognition
Step 1: Install Raspberry Pi OS
- Download the latest version of Raspberry Pi OS from the official Raspberry Pi website: https://www.raspberrypi.org/downloads/
- Write the downloaded image to your microSD card using a tool like Etcher or Win32 Disk Imager.
- Insert the microSD card into your Raspberry Pi and power it on.
Step 2: Configure Audio Input and Output
- Connect your USB microphone and speaker to your Raspberry Pi.
- Open the Raspberry Pi configuration tool by running the following command in the terminal:
sudo raspi-config
- Navigate to “Advanced Options” and select “Audio.”
- Choose the appropriate audio input and output devices for your microphone and speaker.
Step 3: Install Required Software
- Update your Raspberry Pi’s package list and upgrade any installed packages by running the following commands:
sudo apt update
sudo apt upgrade
- Install the required software packages for voice recognition by running the following command:
sudo apt install python3-pip python3-pyaudio
- Install the SpeechRecognition library using pip:
pip3 install SpeechRecognition
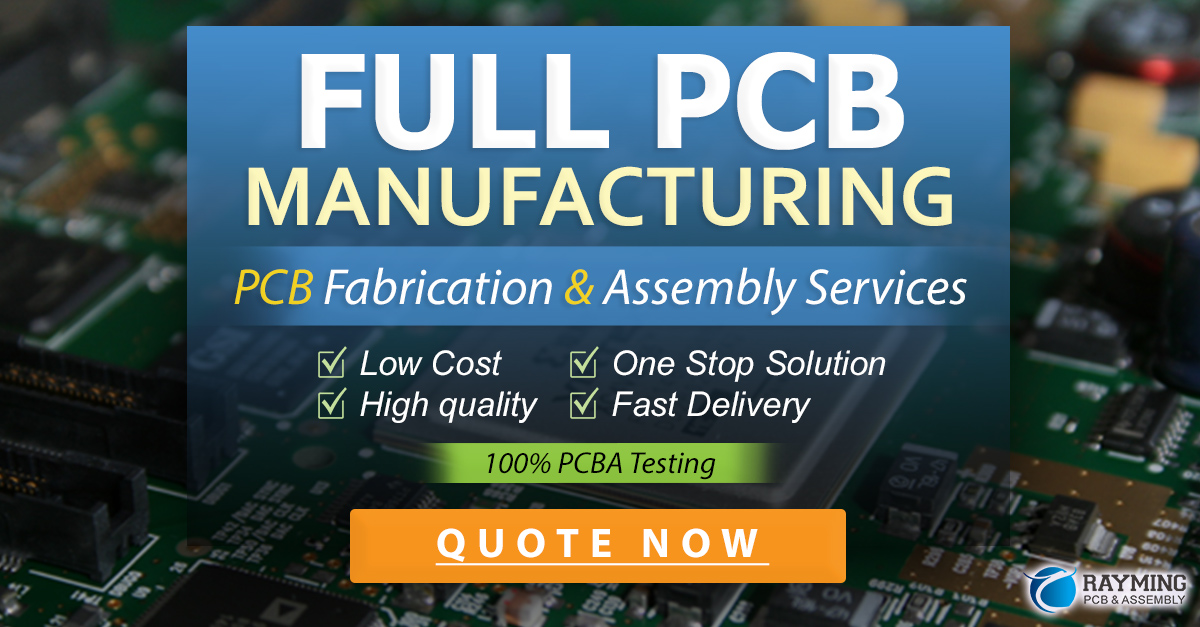
Building a Simple Voice Recognition Project
Now that your Raspberry Pi is set up for voice recognition, let’s build a simple project that demonstrates the basic functionality of voice recognition.
Step 1: Create a new Python file
- Open a new terminal window on your Raspberry Pi.
- Navigate to the directory where you want to store your project files.
- Create a new Python file named
voice_recognition.py
using the following command:
nano voice_recognition.py
Step 2: Write the Python code
Copy and paste the following code into the voice_recognition.py
file:
import speech_recognition as sr
def main():
r = sr.Recognizer()
with sr.Microphone() as source:
print("Say something!")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print(f"You said: {text}")
except sr.UnknownValueError:
print("Sorry, I could not understand what you said.")
except sr.RequestError as e:
print(f"Could not request results from Google Speech Recognition service; {e}")
if __name__ == "__main__":
main()
Step 3: Run the program
Save the voice_recognition.py
file and exit the nano editor. Run the program using the following command:
python3 voice_recognition.py
The program will prompt you to say something. Speak clearly into your microphone, and the program will attempt to recognize your speech using the Google Speech Recognition service. If successful, it will print the recognized text. If there are any errors or if the speech is not recognized, appropriate error messages will be displayed.
Enhancing Your Voice Recognition Project
Adding Voice Commands
You can extend your voice recognition project to execute specific actions based on the recognized voice commands. Here’s an example of how you can modify the voice_recognition.py
file to handle different commands:
import speech_recognition as sr
import subprocess
def execute_command(command):
if command == "open browser":
subprocess.Popen(["chromium-browser"])
elif command == "play music":
subprocess.Popen(["vlc", "path/to/music/file.mp3"])
else:
print(f"Unknown command: {command}")
def main():
r = sr.Recognizer()
with sr.Microphone() as source:
print("Say a command!")
audio = r.listen(source)
try:
command = r.recognize_google(audio)
print(f"You said: {command}")
execute_command(command)
except sr.UnknownValueError:
print("Sorry, I could not understand the command.")
except sr.RequestError as e:
print(f"Could not request results from Google Speech Recognition service; {e}")
if __name__ == "__main__":
main()
In this modified version, the execute_command()
function is added to handle specific voice commands. When a recognized command matches a predefined action, the corresponding subprocess is executed. You can customize the commands and their associated actions based on your project requirements.
Integrating with Other Services
You can further enhance your Raspberry Pi voice recognition project by integrating it with other services and APIs. Some popular integrations include:
- Home Automation: Control smart home devices using voice commands by integrating with platforms like Home Assistant or OpenHAB.
- Weather Updates: Retrieve weather information based on voice queries by integrating with weather APIs such as OpenWeatherMap or Weather Underground.
- Music Playback: Control music playback on your Raspberry Pi using voice commands by integrating with music players like VLC or MPD.
- Personal Assistant: Build your own personal assistant by integrating with natural language processing libraries like spaCy or NLTK.
Frequently Asked Questions (FAQ)
-
Q: Can I use any microphone with my Raspberry Pi for voice recognition?
A: While most USB microphones should work with the Raspberry Pi, it’s recommended to use a microphone specifically designed for voice recognition or one that has been tested and confirmed to work well with the Raspberry Pi. -
Q: Is an internet connection required for voice recognition on the Raspberry Pi?
A: If you are using online speech recognition services like Google Speech Recognition, an internet connection is required. However, there are offline speech recognition libraries available, such as CMU Sphinx or Kaldi, that can be used without an internet connection. -
Q: Can I use languages other than English for voice recognition on the Raspberry Pi?
A: Yes, most speech recognition libraries and services support multiple languages. You can specify the language you want to use when initializing the recognizer or making API requests. -
Q: How can I improve the accuracy of voice recognition on the Raspberry Pi?
A: To improve the accuracy of voice recognition, ensure that you are using a high-quality microphone and that the Raspberry Pi is placed in a quiet environment. You can also try adjusting the microphone sensitivity and speaking clearly and distinctly. -
Q: Are there any limitations to voice recognition on the Raspberry Pi?
A: The performance of voice recognition on the Raspberry Pi may be limited by the available processing power and memory. Complex voice recognition tasks or continuous speech recognition may require more powerful hardware or optimized software implementations.
Conclusion
Voice recognition on the Raspberry Pi is an exciting and accessible way to build interactive projects that respond to spoken commands. By following the steps outlined in this article, you can set up your Raspberry Pi for voice recognition and create a simple project that demonstrates the basic functionality.
As you gain more experience with voice recognition on the Raspberry Pi, you can explore more advanced techniques and integrations to create sophisticated voice-controlled applications. With the power of the Raspberry Pi and the ever-evolving field of voice recognition technology, the possibilities are endless.
So, grab your Raspberry Pi, connect a microphone, and start building your own voice recognition projects today!
Leave a Reply