Introduction to Raspberry Pi Robotics
Robotics has become an increasingly popular hobby and educational tool in recent years, and the Raspberry Pi has played a significant role in making it accessible to beginners and enthusiasts alike. The Raspberry Pi is a credit card-sized single-board computer that offers a cost-effective and versatile platform for building robots. With its powerful processing capabilities, extensive GPIO (General Purpose Input/Output) pins, and compatibility with a wide range of sensors and actuators, the Raspberry Pi has become a go-to choice for robotics projects.
In this article, we will dive into the world of Raspberry Pi robotics and guide you through the process of building your very own Raspberry Pi robot. We will cover the essential components, software setup, and provide step-by-step instructions to help you create a functional and interactive robot.
Why Choose Raspberry Pi for Robotics?
Before we delve into the details of building a Raspberry Pi robot, let’s explore the reasons why the Raspberry Pi is an excellent choice for robotics projects:
-
Affordability: The Raspberry Pi is an inexpensive single-board computer, making it accessible to a wide range of users, from beginners to experienced hobbyists.
-
Versatility: With its powerful processor, GPIO pins, and support for various programming languages, the Raspberry Pi can be used for a wide range of robotics applications, from simple wheeled robots to more complex humanoid robots.
-
Large Community: The Raspberry Pi has a vast and active community of developers, enthusiasts, and educators who share their knowledge, projects, and resources, making it easier for beginners to get started and find support.
-
Educational Value: Building a Raspberry Pi robot is an excellent way to learn about programming, electronics, and robotics concepts, making it a valuable educational tool for students and self-learners.
Components Required for Building a Raspberry Pi Robot
To build a Raspberry Pi robot, you will need the following components:
Component | Description |
---|---|
Raspberry Pi Board | The brain of your robot, responsible for processing and controlling all the components. |
Motor Controller | Allows the Raspberry Pi to control the motors and drive the robot’s movements. |
DC Motors | Provide the locomotion for your robot, enabling it to move and navigate. |
Wheels | Attached to the motors, allowing the robot to move on various surfaces. |
Battery Pack | Powers the Raspberry Pi and other components, ensuring the robot can operate untethered. |
Ultrasonic Sensor | Enables the robot to detect obstacles and measure distances. |
Jumper Wires | Used to connect the various components to the Raspberry Pi’s GPIO pins. |
Breadboard | Provides a prototyping platform for connecting components without soldering. |
Chassis or Robot Platform | Serves as the physical structure and base for mounting all the components. |
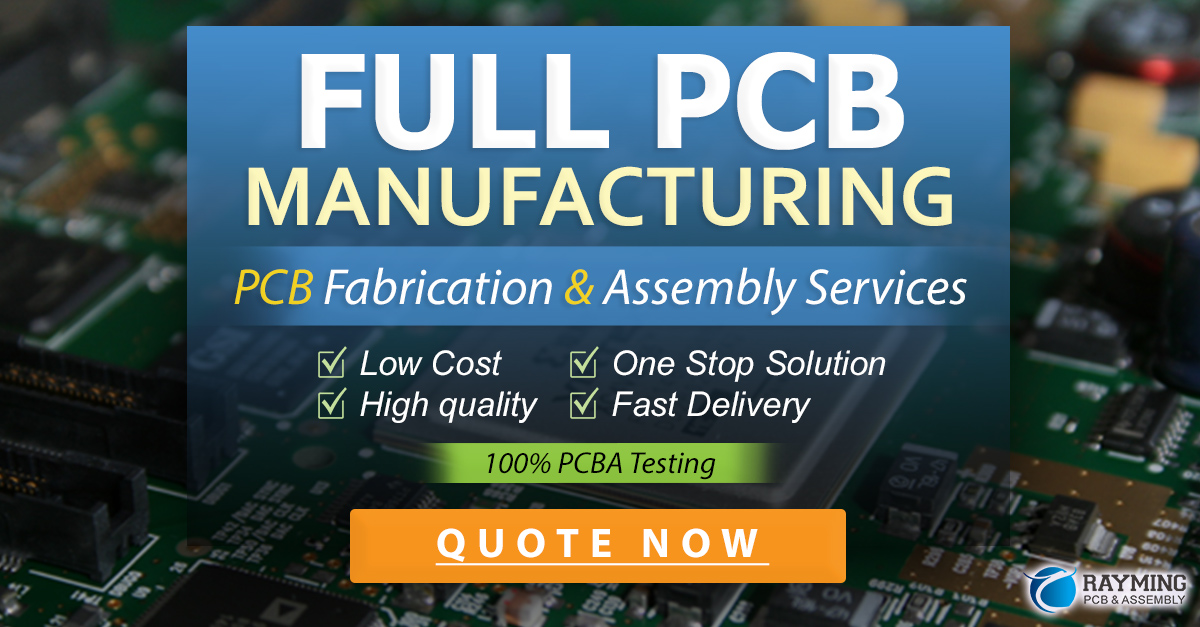
Step 1: Setting Up the Raspberry Pi
Before you start building your robot, you need to set up your Raspberry Pi with the necessary software and configuration.
1.1 Installing the Operating System
- Download the latest version of the Raspberry Pi operating system (e.g., Raspbian) from the official Raspberry Pi website.
- Use a tool like Etcher to write the operating system image to a microSD card.
- Insert the microSD card into your Raspberry Pi.
1.2 Initial Configuration
- Connect a keyboard, mouse, and monitor to your Raspberry Pi.
- Power on the Raspberry Pi and follow the initial setup wizard to configure basic settings like language, time zone, and Wi-Fi connection.
- Update the system by running the following commands in the terminal:
sudo apt update
sudo apt upgrade
1.3 Enabling SSH and Remote Access (Optional)
If you want to control your Raspberry Pi remotely or access it via SSH, you need to enable SSH:
- Open the terminal and run the following command:
sudo raspi-config
- Navigate to “Interfacing Options” and enable SSH.
- Reboot your Raspberry Pi for the changes to take effect.
Step 2: Assembling the Robot Chassis
The next step is to assemble the physical structure of your robot using the chassis or robot platform.
2.1 Mounting the Motors
- Attach the DC motors to the designated slots or mount points on the chassis, ensuring they are securely fastened.
- Connect the wheels to the motor shafts, making sure they are tightly fitted.
2.2 Placing the Raspberry Pi and Other Components
- Find a suitable location on the chassis to mount the Raspberry Pi board, ensuring it is stable and accessible.
- Place the battery pack and secure it to the chassis, keeping in mind the weight distribution and balance of the robot.
- Position the breadboard on the chassis, making sure it is easily accessible for connecting components.
Step 3: Wiring the Components
With the chassis assembled, it’s time to connect the various components to the Raspberry Pi using jumper wires and the breadboard.
3.1 Connecting the Motor Controller
- Connect the motor controller to the Raspberry Pi’s GPIO pins according to the manufacturer’s instructions.
- Typically, you will need to connect the motor controller’s power and ground pins to the Raspberry Pi’s 5V and GND pins, respectively.
- Connect the control pins of the motor controller to the appropriate GPIO pins on the Raspberry Pi.
3.2 Wiring the DC Motors
- Connect the positive and negative terminals of each DC motor to the corresponding output pins on the motor controller.
- Ensure the polarity of the connections is correct to avoid damaging the motors or causing unexpected behavior.
3.3 Connecting the Ultrasonic Sensor
- Identify the VCC, GND, Trigger, and Echo pins on the ultrasonic sensor.
- Connect the VCC and GND pins to the Raspberry Pi’s 5V and GND pins, respectively.
- Connect the Trigger and Echo pins to the designated GPIO pins on the Raspberry Pi.
Step 4: Programming the Robot
With the hardware assembly complete, it’s time to bring your robot to life by programming it using the Raspberry Pi.
4.1 Installing Required Libraries
- Open the terminal on your Raspberry Pi and install the necessary libraries for controlling the motor controller and ultrasonic sensor.
- For example, if you are using the L298N motor controller and the HC-SR04 ultrasonic sensor, you can install the RPi.GPIO library by running the following command:
sudo apt install python3-rpi.gpio
4.2 Writing the Robot Control Code
- Create a new Python file and import the required libraries.
- Initialize the GPIO pins for the motor controller and ultrasonic sensor.
- Write functions to control the robot’s movements, such as moving forward, backward, turning left, and turning right.
- Implement a function to read the distance measurements from the ultrasonic sensor.
- Create a main loop that continuously reads sensor data and makes decisions based on the measured distances to navigate the robot autonomously.
Here’s a simplified example of a basic robot control code:
import RPi.GPIO as GPIO
import time
# Motor controller pins
IN1 = 18
IN2 = 23
IN3 = 24
IN4 = 25
# Ultrasonic sensor pins
TRIGGER = 17
ECHO = 27
# Set up GPIO pins
GPIO.setmode(GPIO.BCM)
GPIO.setup(IN1, GPIO.OUT)
GPIO.setup(IN2, GPIO.OUT)
GPIO.setup(IN3, GPIO.OUT)
GPIO.setup(IN4, GPIO.OUT)
GPIO.setup(TRIGGER, GPIO.OUT)
GPIO.setup(ECHO, GPIO.IN)
def move_forward():
GPIO.output(IN1, GPIO.HIGH)
GPIO.output(IN2, GPIO.LOW)
GPIO.output(IN3, GPIO.HIGH)
GPIO.output(IN4, GPIO.LOW)
def move_backward():
GPIO.output(IN1, GPIO.LOW)
GPIO.output(IN2, GPIO.HIGH)
GPIO.output(IN3, GPIO.LOW)
GPIO.output(IN4, GPIO.HIGH)
def turn_left():
GPIO.output(IN1, GPIO.LOW)
GPIO.output(IN2, GPIO.HIGH)
GPIO.output(IN3, GPIO.HIGH)
GPIO.output(IN4, GPIO.LOW)
def turn_right():
GPIO.output(IN1, GPIO.HIGH)
GPIO.output(IN2, GPIO.LOW)
GPIO.output(IN3, GPIO.LOW)
GPIO.output(IN4, GPIO.HIGH)
def stop():
GPIO.output(IN1, GPIO.LOW)
GPIO.output(IN2, GPIO.LOW)
GPIO.output(IN3, GPIO.LOW)
GPIO.output(IN4, GPIO.LOW)
def measure_distance():
GPIO.output(TRIGGER, GPIO.HIGH)
time.sleep(0.00001)
GPIO.output(TRIGGER, GPIO.LOW)
while GPIO.input(ECHO) == 0:
pulse_start = time.time()
while GPIO.input(ECHO) == 1:
pulse_end = time.time()
pulse_duration = pulse_end - pulse_start
distance = pulse_duration * 17150
distance = round(distance, 2)
return distance
try:
while True:
distance = measure_distance()
print(f"Distance: {distance} cm")
if distance > 20:
move_forward()
else:
stop()
time.sleep(1)
turn_left()
time.sleep(1)
except KeyboardInterrupt:
GPIO.cleanup()
This code sets up the GPIO pins for the motor controller and ultrasonic sensor, defines functions for controlling the robot’s movements and measuring distances, and implements a simple autonomous navigation logic based on the measured distances.
4.3 Testing and Refinement
- Run the robot control code on your Raspberry Pi and observe the robot’s behavior.
- Test the robot in different environments and scenarios to assess its performance and reliability.
- Refine the code and make necessary adjustments based on your observations and desired functionality.
Step 5: Enhancing Your Raspberry Pi Robot
With the basic functionality of your Raspberry Pi robot in place, you can explore various ways to enhance and expand its capabilities. Here are a few ideas:
-
Adding Sensors: Incorporate additional sensors, such as infrared sensors, line following sensors, or a camera module, to enable more advanced navigation and object detection capabilities.
-
Wireless Control: Implement wireless control functionality using technologies like Bluetooth or Wi-Fi, allowing you to control your robot remotely using a mobile app or web interface.
-
Computer Vision: Integrate computer vision techniques using libraries like OpenCV to enable your robot to recognize and track objects, faces, or specific patterns.
-
Machine Learning: Explore machine learning algorithms to make your robot more intelligent and adaptable, enabling it to learn from its environment and make autonomous decisions.
-
Robotic Arm: Attach a robotic arm or gripper to your Raspberry Pi robot to enable manipulation and interaction with objects.
-
Voice Control: Implement voice recognition and synthesis capabilities to control your robot using voice commands and enable it to provide spoken feedback.
Conclusion
Building a Raspberry Pi robot is an exciting and rewarding project that allows you to explore the world of robotics and develop valuable skills in programming, electronics, and problem-solving. By following the steps outlined in this article, you can create a functional and interactive robot using the Raspberry Pi as the central processing unit.
Remember to start with a basic setup and gradually enhance your robot’s capabilities as you gain more experience and confidence. The Raspberry Pi robotics community is vast and supportive, so don’t hesitate to seek guidance, inspiration, and collaboration from fellow enthusiasts and experts.
With dedication, creativity, and a willingness to learn, you can unleash the potential of your Raspberry Pi robot and embark on a journey of innovation and discovery in the fascinating field of robotics.
Frequently Asked Questions (FAQ)
-
What programming language is commonly used for Raspberry Pi robotics?
Python is the most popular programming language for Raspberry Pi robotics due to its simplicity, versatility, and extensive library support. Other languages like C++ and Java can also be used, depending on the specific requirements of your project. -
Can I use other single-board computers instead of Raspberry Pi for building robots?
Yes, there are several other single-board computers available, such as Arduino, BeagleBone, and NVIDIA Jetson, that can be used for robotics projects. However, the Raspberry Pi has gained significant popularity due to its affordability, extensive community support, and wide range of compatible sensors and actuators. -
How can I power my Raspberry Pi robot?
You can power your Raspberry Pi robot using a battery pack or a power bank. It’s essential to choose a power source that provides sufficient capacity and voltage to power the Raspberry Pi and other components. Commonly used options include lithium-ion batteries, NiMH batteries, or USB power banks with a 5V output. -
What are some common challenges faced when building a Raspberry Pi robot?
Some common challenges include wiring and connection issues, compatibility problems between components, power management, and software configuration. It’s important to follow wiring diagrams and documentation carefully, ensure proper power supply, and troubleshoot any issues step by step. Seeking help from the community and referring to online resources can also help overcome challenges. -
Are there any ready-made Raspberry Pi robot kits available?
Yes, there are several Raspberry Pi robot kits available in the market that provide all the necessary components, instructions, and sometimes even pre-assembled chassis. These kits can be a great starting point for beginners who want to quickly get started with Raspberry Pi robotics without the need for extensive hardware knowledge or sourcing individual components.
Leave a Reply