Introduction to Beaglebone Black
The Beaglebone Black is a low-cost, community-supported development platform for developers and hobbyists. This credit-card-sized Linux computer is produced by Texas Instruments in association with Digi-Key and Newark element14. It is a powerful device that can be used in various applications, from robotics to home automation.
Key Features of Beaglebone Black
- AM335x 1GHz ARM® Cortex-A8 processor
- 512MB DDR3 RAM
- 4GB 8-bit eMMC on-board flash storage
- 3D graphics accelerator
- NEON floating-point accelerator
- 2x PRU 32-bit microcontrollers
- USB client for power & communications
- USB host
- Ethernet
- HDMI
- 2x 46 pin headers
Beaglebone Black Pinout
The Beaglebone Black has two 46-pin headers, P8 and P9, that provide access to the various interfaces and peripherals of the board. These headers allow users to connect various sensors, actuators, and other devices to the board.
P8 Header Pinout
Pin | Function | Pin | Function |
---|---|---|---|
1 | GND | 2 | GND |
3 | GPIO1_6 | 4 | GPIO1_7 |
5 | GPIO1_2 | 6 | GPIO1_3 |
7 | TIMER4 | 8 | TIMER7 |
9 | TIMER5 | 10 | TIMER6 |
11 | GPIO1_13 | 12 | GPIO1_12 |
13 | EHRPWM2B | 14 | GPIO0_26 |
15 | GPIO1_15 | 16 | GPIO1_14 |
17 | GPIO0_27 | 18 | GPIO2_1 |
19 | EHRPWM2A | 20 | GPIO1_31 |
21 | GPIO1_30 | 22 | GPIO1_5 |
23 | GPIO1_4 | 24 | GPIO1_1 |
25 | GPIO1_0 | 26 | GPIO1_29 |
27 | GPIO2_22 | 28 | GPIO2_24 |
29 | GPIO2_23 | 30 | GPIO2_25 |
31 | UART5_CTSN | 32 | UART5_RTSN |
33 | UART4_RTSN | 34 | UART3_RTSN |
35 | UART4_CTSN | 36 | UART3_CTSN |
37 | UART5_TXD | 38 | UART5_RXD |
39 | GPIO2_12 | 40 | GPIO2_13 |
41 | GPIO2_10 | 42 | GPIO2_11 |
43 | GPIO2_8 | 44 | GPIO2_9 |
45 | GPIO2_6 | 46 | GPIO2_7 |
P9 Header Pinout
Pin | Function | Pin | Function |
---|---|---|---|
1 | GND | 2 | GND |
3 | DC_3.3V | 4 | DC_3.3V |
5 | VDD_5V | 6 | VDD_5V |
7 | SYS_5V | 8 | SYS_5V |
9 | PWR_BUT | 10 | SYS_RESETn |
11 | UART4_RXD | 12 | GPIO1_28 |
13 | UART4_TXD | 14 | EHRPWM1A |
15 | GPIO1_16 | 16 | EHRPWM1B |
17 | I2C1_SCL | 18 | I2C1_SDA |
19 | I2C2_SCL | 20 | I2C2_SDA |
21 | UART2_TXD | 22 | UART2_RXD |
23 | GPIO1_17 | 24 | UART1_TXD |
25 | GPIO3_21 | 26 | UART1_RXD |
27 | GPIO3_19 | 28 | SPI1_CS0 |
29 | SPI1_D0 | 30 | SPI1_D1 |
31 | SPI1_SCLK | 32 | VDD_ADC |
33 | AIN4 | 34 | GNDA_ADC |
35 | AIN6 | 36 | AIN5 |
37 | AIN2 | 38 | AIN3 |
39 | AIN0 | 40 | AIN1 |
41 | CLKOUT2 | 42 | GPIO0_7 |
43 | GND | 44 | GND |
45 | GND | 46 | GND |
Beaglebone Black Pin Configuration
The Beaglebone Black uses a pin multiplexing system, which allows each pin to have multiple functions. The specific function of each pin is determined by the pin multiplexing configuration.
GPIO (General Purpose Input/Output)
GPIO pins can be configured as either inputs or outputs. They are commonly used to interface with various sensors, buttons, LEDs, and other devices.
Example code for configuring a GPIO pin as an output and toggling its state:
import Adafruit_BBIO.GPIO as GPIO
import time
LED_PIN = "P8_7"
GPIO.setup(LED_PIN, GPIO.OUT)
while True:
GPIO.output(LED_PIN, GPIO.HIGH)
time.sleep(1)
GPIO.output(LED_PIN, GPIO.LOW)
time.sleep(1)
PWM (Pulse Width Modulation)
PWM pins can generate a pulse width modulated signal, which is useful for controlling the brightness of LEDs, the speed of motors, or the position of servos.
Example code for generating a PWM signal:
import Adafruit_BBIO.PWM as PWM
PWM_PIN = "P9_14"
PWM.start(PWM_PIN, 50, 1000)
while True:
duty_cycle = input("Enter duty cycle (0-100): ")
PWM.set_duty_cycle(PWM_PIN, float(duty_cycle))
I2C (Inter-Integrated Circuit)
I2C is a serial communication protocol that allows multiple devices to communicate with each other using just two wires: SCL (Serial Clock) and SDA (Serial Data).
Example code for reading data from an I2C device:
import Adafruit_BBIO.I2C as I2C
DEVICE_ADDR = 0x68
i2c = I2C.I2C(2)
i2c.write8(DEVICE_ADDR, 0x00)
data = i2c.readU8(DEVICE_ADDR, 0x01)
print("Data:", data)
SPI (Serial Peripheral Interface)
SPI is another serial communication protocol that uses four wires: SCLK (Serial Clock), MOSI (Master Output Slave Input), MISO (Master Input Slave Output), and CS (Chip Select).
Example code for reading data from an SPI device:
import Adafruit_BBIO.SPI as SPI
spi = SPI.SPI(0, 0)
spi.mode = 0
spi.msh = 1000000
data = spi.xfer2([0x01, 0x02, 0x03])
print("Data:", data)
UART (Universal Asynchronous Receiver-Transmitter)
UART is a serial communication protocol that is commonly used for communication between the Beaglebone Black and other devices, such as GPS modules or Bluetooth modules.
Example code for reading data from a UART device:
import Adafruit_BBIO.UART as UART
import serial
UART.setup("UART1")
ser = serial.Serial(port="/dev/ttyO1", baudrate=9600)
data = ser.read(10)
print("Data:", data)
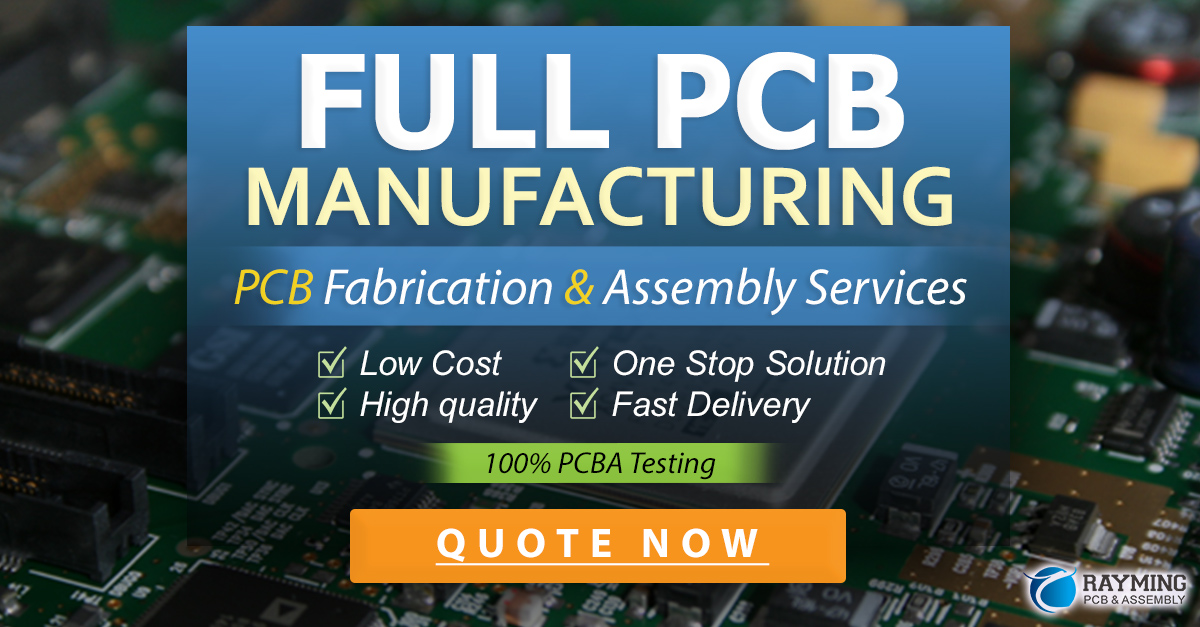
Beaglebone Black Pin Specifications
The Beaglebone Black’s pins have specific electrical characteristics that must be considered when interfacing with external devices.
Voltage Levels
- GPIO pins: 3.3V
- VDD_5V pins: 5V
- SYS_5V pins: 5V
- DC_3.3V pins: 3.3V
- VDD_ADC pin: 1.8V
Current Limits
- GPIO pins: 4-6mA
- VDD_5V and SYS_5V pins: 250mA (combined)
- DC_3.3V pins: 250mA (combined)
ADC (Analog-to-Digital Converter)
The Beaglebone Black has a 12-bit ADC with 7 channels (AIN0 to AIN6). The ADC has a voltage range of 0-1.8V.
Example code for reading an analog value from an ADC pin:
import Adafruit_BBIO.ADC as ADC
import time
ADC.setup()
ANALOG_PIN = "P9_40"
while True:
value = ADC.read(ANALOG_PIN) * 1.8
print("Voltage:", value)
time.sleep(0.1)
Beaglebone Black Pin Applications
The versatility of the Beaglebone Black’s pins allows for a wide range of applications, from simple projects to complex systems.
Sensor Interfacing
The Beaglebone Black can interface with various sensors using its GPIO, I2C, SPI, and ADC pins. Some common sensors include:
- Temperature sensors (e.g., LM35, DS18B20)
- Humidity sensors (e.g., DHT11, DHT22)
- Pressure sensors (e.g., BMP180, MPL3115A2)
- Accelerometers and gyroscopes (e.g., MPU6050, LSM9DS1)
Motor Control
PWM pins can be used to control the speed and direction of DC motors and servos. The Beaglebone Black can also interface with motor drivers (e.g., L293D, TB6612FNG) to control larger motors.
Display and User Interface
The Beaglebone Black can interface with various displays and user interface components using its GPIO and I2C pins:
- LCD and OLED displays (e.g., SSD1306, ST7735)
- Touchscreens (e.g., TSC2046)
- Buttons and switches
- Rotary encoders
Communication and Networking
The Beaglebone Black’s UART pins can be used to communicate with other devices, such as GPS modules, Bluetooth modules, or other microcontrollers. The board also has built-in Ethernet and USB host capabilities for networking and communication with other devices.
Frequently Asked Questions (FAQ)
-
What is the maximum current that can be drawn from the Beaglebone Black’s GPIO pins?
The maximum current that can be safely drawn from a single GPIO pin is 4-6mA. If more current is required, an external driver circuit should be used. -
Can I use the Beaglebone Black’s ADC pins to measure voltages higher than 1.8V?
No, the ADC pins have a maximum input voltage of 1.8V. To measure higher voltages, you must use a voltage divider circuit to scale the voltage down to the appropriate range. -
How can I control the brightness of an LED using the Beaglebone Black?
To control the brightness of an LED, you can use one of the PWM pins. By varying the duty cycle of the PWM signal, you can adjust the brightness of the LED. -
Can I use the Beaglebone Black to control a 5V device using the GPIO pins?
No, the GPIO pins operate at 3.3V and cannot directly control 5V devices. To interface with 5V devices, you must use a level shifter or an appropriate driver circuit. -
How do I communicate with I2C devices using the Beaglebone Black?
To communicate with I2C devices, you can use the Adafruit_BBIO library in Python. First, set up the I2C bus using the I2C.setup() function, then use the I2C.read() and I2C.write() functions to read from and write to the device.
Conclusion
The Beaglebone Black is a powerful and versatile single-board computer that offers a wide range of possibilities for developers and hobbyists. With its extensive pin headers and various interfaces, the Beaglebone Black can be used in a multitude of applications, from simple projects to complex systems.
By understanding the Beaglebone Black’s pinout, pin configuration, and specifications, users can effectively utilize the board’s capabilities and create innovative projects. The provided code examples and explanations serve as a starting point for working with the different interfaces and peripherals available on the Beaglebone Black.
As the Beaglebone Black community continues to grow, there is an ever-increasing number of resources, libraries, and projects available to help users make the most of this powerful device. Whether you are a beginner or an experienced developer, the Beaglebone Black offers an excellent platform for learning, experimentation, and innovation.
Leave a Reply