What are Arduino Data Types?
Data types in Arduino define the type of data that a variable can hold. They specify the size and format of the data stored in a variable. Arduino provides several built-in data types that you can use to store and manipulate different kinds of data in your programs.
Primitive Data Types
Primitive data types are the most basic data types available in Arduino. They include:
Integer Data Types
Integer data types are used to store whole numbers, both positive and negative. Arduino provides several integer data types with different ranges and sizes:
Data Type | Size (bytes) | Range |
---|---|---|
int | 2 | -32,768 to 32,767 |
unsigned int | 2 | 0 to 65,535 |
long | 4 | -2,147,483,648 to 2,147,483,647 |
unsigned long | 4 | 0 to 4,294,967,295 |
short | 2 | -32,768 to 32,767 |
byte | 1 | 0 to 255 |
Here’s an example of declaring and using an integer variable:
int myNumber = 42;
Serial.println(myNumber); // Output: 42
Floating-Point Data Types
Floating-point data types are used to store numbers with decimal points. Arduino provides two floating-point data types:
Data Type | Size (bytes) | Range |
---|---|---|
float | 4 | -3.4028235E+38 to 3.4028235E+38 |
double | 4 | -3.4028235E+38 to 3.4028235E+38 |
Note that double
has the same size and range as float
in Arduino.
Here’s an example of declaring and using a floating-point variable:
float myFloat = 3.14;
Serial.println(myFloat); // Output: 3.14
Character Data Type
The character data type (char
) is used to store single characters. It occupies 1 byte of memory and can store ASCII characters.
Here’s an example of declaring and using a character variable:
char myChar = 'A';
Serial.println(myChar); // Output: A
Boolean Data Type
The boolean data type (bool
) is used to store logical values of either true
or false
. It occupies 1 byte of memory.
Here’s an example of declaring and using a boolean variable:
bool myBoolean = true;
Serial.println(myBoolean); // Output: 1 (true)
Compound Data Types
Compound data types are more complex data types that are composed of primitive data types. Arduino provides two main compound data types:
Arrays
Arrays allow you to store multiple values of the same data type in a single variable. To declare an array, you specify the data type followed by the array name and the number of elements in square brackets.
Here’s an example of declaring and using an integer array:
int myArray[5] = {1, 2, 3, 4, 5};
Serial.println(myArray[2]); // Output: 3
Strings
Strings are used to store sequences of characters. Arduino provides a built-in String
class that allows you to manipulate strings easily.
Here’s an example of declaring and using a string variable:
String myString = "Hello, Arduino!";
Serial.println(myString); // Output: Hello, Arduino!
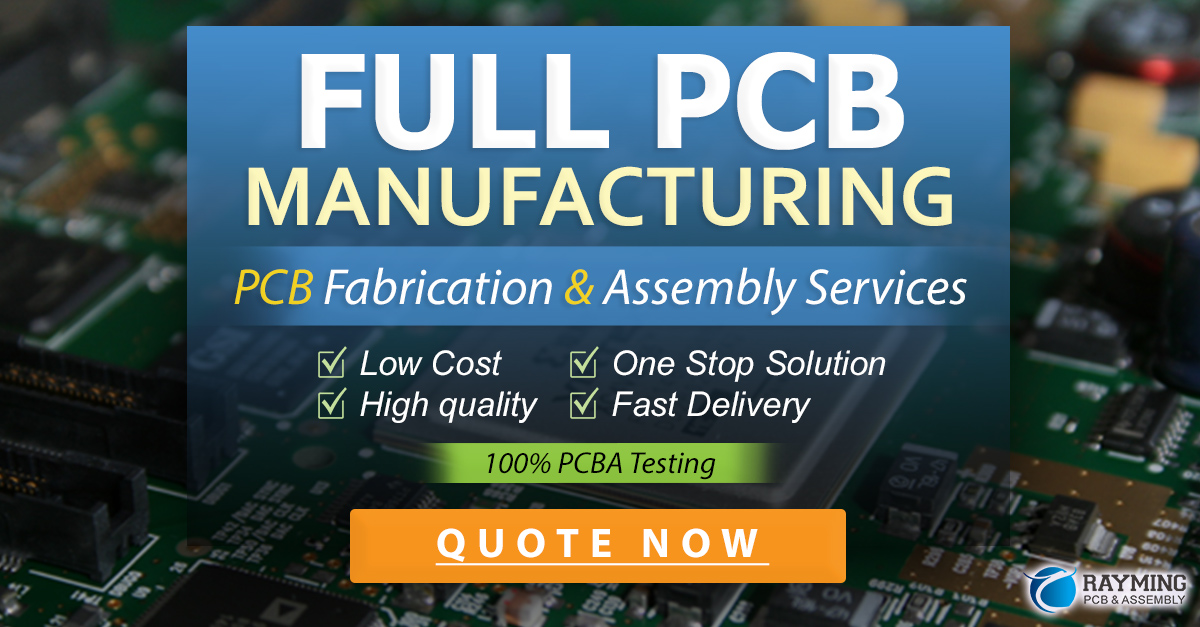
Data Type Conversion
Arduino allows you to convert between different data types using type casting. Type casting is the process of explicitly converting one data type to another.
Here’s an example of converting an integer to a floating-point number:
int myInt = 10;
float myFloat = (float)myInt;
Serial.println(myFloat); // Output: 10.00
You can also use functions like int()
, float()
, char()
, etc., to perform type conversion.
Choosing the Right Data Type
When selecting a data type for your variables, consider the following factors:
- Range: Ensure that the data type can accommodate the range of values you expect to store.
- Precision: For floating-point numbers, consider the precision required for your calculations.
- Memory usage: Choose data types that optimize memory usage, especially when dealing with large arrays or limited memory.
- Compatibility: Make sure the data types are compatible with the functions and libraries you plan to use.
FAQ
-
Q: What is the difference between
int
andlong
data types in Arduino?
A: Theint
data type is 2 bytes in size and can store values from -32,768 to 32,767, while thelong
data type is 4 bytes in size and can store values from -2,147,483,648 to 2,147,483,647. -
Q: Can I use the
double
data type instead offloat
for better precision?
A: In Arduino, thedouble
data type has the same size and precision as thefloat
data type. Therefore, usingdouble
will not provide any additional precision. -
Q: How do I declare a variable as a constant in Arduino?
A: To declare a variable as a constant in Arduino, you can use theconst
keyword before the data type. For example:const int MY_CONSTANT = 42;
. -
Q: What is the purpose of the
byte
data type in Arduino?
A: Thebyte
data type is used to store values from 0 to 255. It is commonly used when working with raw binary data or when you need to optimize memory usage for large arrays. -
Q: How can I convert a string to an integer in Arduino?
A: Arduino provides thetoInt()
function to convert a string to an integer. For example:int myInt = myString.toInt();
.
In conclusion, understanding Arduino data types is crucial for writing efficient and effective code. By choosing the appropriate data types for your variables and leveraging type conversion when necessary, you can create robust and optimized Arduino programs. Remember to consider factors like range, precision, memory usage, and compatibility when selecting data types for your projects.
Leave a Reply