Introduction to Alarm Clock Circuits
An alarm clock circuit is an electronic device designed to alert the user at a pre-set time. It consists of various components that work together to keep accurate time and trigger an alarm when the set time is reached. In this article, we will explore the design and working principle of alarm clock circuits, discussing their key components, functionality, and practical applications.
Key Components of an Alarm Clock Circuit
The main components of a typical alarm clock circuit include:
- Microcontroller or Integrated Circuit (IC)
- Real-Time Clock (RTC) module
- Display (LCD or LED)
- Keypad or buttons for user input
- Buzzer or speaker for the alarm
- Power supply
Microcontroller or Integrated Circuit (IC)
The microcontroller or IC is the brain of the alarm clock circuit. It processes user input, controls the display, and triggers the alarm when the set time matches the current time. Some popular microcontrollers used in alarm clock circuits include:
Microcontroller | Description |
---|---|
Arduino Uno | An open-source microcontroller board based on the ATmega328P chip |
PIC16F877A | A versatile microcontroller from Microchip Technology |
ATmega328P | A low-power, high-performance 8-bit microcontroller from Atmel |
Real-Time Clock (RTC) Module
The RTC module is responsible for keeping accurate time, even when the main power is off. It typically uses a separate battery to maintain the time and date information. Some common RTC modules include:
RTC Module | Description |
---|---|
DS1307 | A low-power, full binary-coded decimal (BCD) clock/calendar with 56 bytes of non-volatile SRAM |
DS3231 | A highly accurate, temperature-compensated RTC with an integrated crystal oscillator |
PCF8563 | A low-power RTC with a programmable clock output and alarm function |
Display (LCD or LED)
The display shows the current time and allows the user to view and set the alarm time. Two common display types used in alarm clock circuits are:
- Liquid Crystal Display (LCD): LCDs offer a clear, readable display and are available in various sizes, such as 16×2 or 20×4 characters.
- Light-Emitting Diode (LED) Display: LED displays are bright, energy-efficient, and available in different colors and sizes, such as 7-segment or 8×8 matrix displays.
Keypad or Buttons for User Input
A keypad or buttons allow the user to set the current time, alarm time, and interact with other functions of the alarm clock circuit. The number of buttons required depends on the complexity of the circuit and the desired features.
Buzzer or Speaker for the Alarm
The buzzer or speaker produces the sound when the alarm is triggered. Piezo buzzers are commonly used due to their compact size, low power consumption, and simple integration with microcontrollers.
Power Supply
The power supply provides the necessary voltage and current to the components of the alarm clock circuit. It can be a battery, AC adapter, or a combination of both, depending on the design requirements.
Working Principle of an Alarm Clock Circuit
The working principle of an alarm clock circuit can be summarized in the following steps:
- The user sets the current time and alarm time using the keypad or buttons.
- The microcontroller stores the user input and continuously compares the current time from the RTC module with the set alarm time.
- The display shows the current time, which is updated by the microcontroller based on the information from the RTC module.
- When the current time matches the set alarm time, the microcontroller triggers the buzzer or speaker to produce the alarm sound.
- The user can stop the alarm by pressing a designated button, such as a snooze or dismiss button.
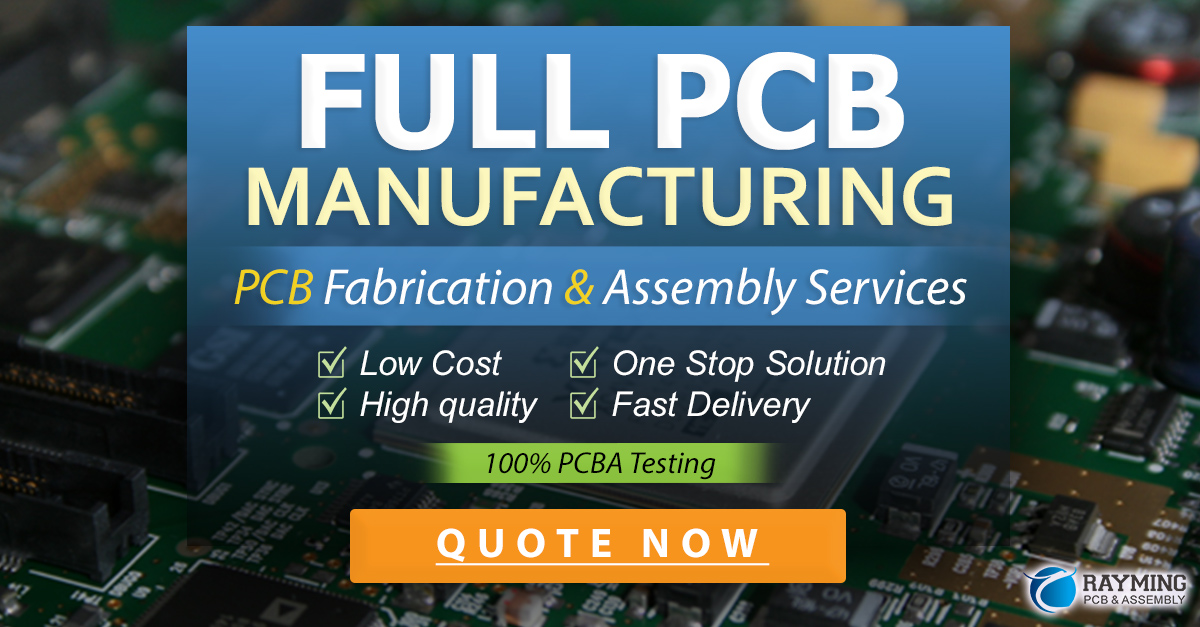
Designing an Alarm Clock Circuit
Designing an alarm clock circuit involves the following steps:
- Choose the appropriate components based on the desired features and complexity of the circuit.
- Create a schematic diagram that shows the connections between the components.
- Write the necessary code for the microcontroller to control the RTC module, display, and alarm functionality.
- Assemble the components on a breadboard or PCB, following the schematic diagram.
- Test the circuit to ensure proper functionality and make any necessary adjustments.
Here’s an example schematic diagram for a basic alarm clock circuit using an Arduino Uno, DS1307 RTC module, 16×2 LCD, and a piezo buzzer:
+5V
|
+--------+--------+
| | |
| DS1307 |
| | |
+--------+--------+
|
| +5V
| |
+--------+-----+--------+
| | |
| Arduino Uno |
| | |
+--------+-----+--------+
| |
| | +5V
| | |
+--------+-----+-----+--------+
| | | |
| 16x2 LCD | Piezo Buzzer
| | | |
+--------+-----------+--------+
Programming the Alarm Clock Circuit
Programming the alarm clock circuit involves writing code for the microcontroller to perform the following tasks:
- Initialize the RTC module and set the current time, if necessary.
- Read the user input from the keypad or buttons to set the alarm time.
- Continuously read the current time from the RTC module and update the display.
- Compare the current time with the set alarm time and trigger the buzzer when they match.
- Implement additional features, such as snooze functionality or multiple alarms, if desired.
Here’s a simple example code for an Arduino-based alarm clock circuit:
#include <Wire.h>
#include <DS1307RTC.h>
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
const int buzzerPin = 9;
const int setButton = 6;
const int upButton = 7;
const int downButton = 8;
int alarmHour = 0;
int alarmMinute = 0;
void setup() {
lcd.begin(16, 2);
pinMode(buzzerPin, OUTPUT);
pinMode(setButton, INPUT_PULLUP);
pinMode(upButton, INPUT_PULLUP);
pinMode(downButton, INPUT_PULLUP);
}
void loop() {
tmElements_t tm;
if (RTC.read(tm)) {
lcd.setCursor(0, 0);
lcd.print("Time: ");
printDigits(tm.Hour);
lcd.print(":");
printDigits(tm.Minute);
lcd.print(":");
printDigits(tm.Second);
if (tm.Hour == alarmHour && tm.Minute == alarmMinute) {
tone(buzzerPin, 1000, 5000);
}
} else {
lcd.setCursor(0, 0);
lcd.print("RTC Error!");
}
if (digitalRead(setButton) == LOW) {
setAlarmTime();
}
}
void printDigits(int digits) {
if (digits < 10) {
lcd.print('0');
}
lcd.print(digits);
}
void setAlarmTime() {
while (digitalRead(setButton) == HIGH) {
if (digitalRead(upButton) == LOW) {
alarmHour = (alarmHour + 1) % 24;
}
if (digitalRead(downButton) == LOW) {
alarmMinute = (alarmMinute + 1) % 60;
}
lcd.setCursor(0, 1);
lcd.print("Alarm: ");
printDigits(alarmHour);
lcd.print(":");
printDigits(alarmMinute);
delay(200);
}
}
Practical Applications of Alarm Clock Circuits
Alarm clock circuits find applications in various devices and systems, including:
- Bedside alarm clocks
- Wall-mounted or desk clocks with alarm functionality
- Wearable devices, such as smartwatches or fitness trackers with wake-up alarms
- Home automation systems that control lights or appliances based on set times
- Industrial control systems that require time-based triggering of events or processes
Troubleshooting Common Issues in Alarm Clock Circuits
When working with alarm clock circuits, you may encounter some common issues. Here are a few troubleshooting tips:
- Incorrect time displayed: Check the connections between the microcontroller and the RTC module, and ensure that the code is correctly initializing and reading the RTC.
- Alarm not triggering: Verify that the alarm time is set correctly and that the code is properly comparing the current time with the set alarm time. Check the buzzer connections and make sure the buzzer is functional.
- Buttons not responding: Ensure that the buttons are correctly connected to the microcontroller and that the code is properly detecting button presses. Use pull-up or pull-down resistors if necessary.
- Display issues: Check the connections between the microcontroller and the display, and verify that the code is sending the correct data to the display. Adjust the contrast if the display is hard to read.
Future Developments in Alarm Clock Circuits
As technology advances, alarm clock circuits continue to evolve and incorporate new features. Some potential future developments include:
- Integration with smart home systems, allowing users to control their alarm clocks through voice assistants or mobile apps.
- Adaptive alarms that adjust the wake-up time based on the user’s sleep patterns and daily schedule.
- Incorporation of biometric sensors to monitor sleep quality and provide personalized recommendations for better sleep habits.
- Wireless charging and battery management for portable alarm clock devices.
- Integration with multimedia systems, allowing users to wake up to their favorite music, podcasts, or videos.
FAQ
Q1. Can I use a different microcontroller or RTC module in my alarm clock circuit?
A1. Yes, you can use any compatible microcontroller or RTC module that meets your requirements. Just make sure to adjust the code and connections accordingly.
Q2. How can I add a snooze function to my alarm clock circuit?
A2. To add a snooze function, you can modify the code to introduce a delay when the snooze button is pressed. During this delay, the alarm will be temporarily silenced, and it will sound again after the specified snooze duration.
Q3. Can I control my alarm clock circuit remotely?
A3. Yes, you can add remote control functionality to your alarm clock circuit by incorporating wireless communication modules, such as Bluetooth or Wi-Fi, and developing a companion mobile app or web interface.
Q4. How do I set multiple alarms on my alarm clock circuit?
A4. To set multiple alarms, you can modify the code to store an array of alarm times and compare the current time with each alarm time in the array. When an alarm time matches the current time, trigger the corresponding alarm action.
Q5. Can I power my alarm clock circuit using a rechargeable battery?
A5. Yes, you can power your alarm clock circuit using a rechargeable battery, such as a lithium-ion battery. Include a battery management system to monitor the battery level and ensure safe charging and discharging. You can also incorporate a charging module to recharge the battery when necessary.
Conclusion
Alarm clock circuits are essential devices that help us stay on schedule and manage our time effectively. By understanding the design and working principle of these circuits, you can create your own custom alarm clock or troubleshoot issues in existing devices. With the rapid advancements in technology, alarm clock circuits are becoming more sophisticated, offering a wide range of features and integrations to enhance the user experience. As you explore the world of alarm clock circuits, remember to experiment, innovate, and adapt to the ever-changing landscape of electronic design.
Leave a Reply