Introduction to Embedded Linux
Embedded Linux is a popular choice for developers working on embedded systems due to its flexibility, robustness, and open-source nature. This tutorial aims to provide a comprehensive guide to embedded Linux, covering everything from the basics to advanced topics.
What is Embedded Linux?
Embedded Linux refers to the use of the Linux operating system in embedded systems, such as smartphones, tablets, routers, and other dedicated devices. It is a stripped-down version of the Linux kernel, tailored to the specific requirements of the embedded device.
Advantages of Using Embedded Linux
- Open-source: Embedded Linux is open-source, which means that the source code is freely available for modification and redistribution.
- Customizable: Embedded Linux can be customized to fit the specific needs of the embedded device, allowing developers to optimize performance and reduce resource usage.
- Cost-effective: Since Embedded Linux is open-source, there are no licensing fees, making it a cost-effective solution for embedded systems.
- Large community support: Embedded Linux has a large and active community of developers who contribute to its development and provide support.
Getting Started with Embedded Linux
Hardware Requirements
To get started with Embedded Linux, you will need the following hardware:
- A development board (e.g., Raspberry Pi, BeagleBone, etc.)
- A host computer (Linux, macOS, or Windows)
- A serial cable or USB-to-serial converter
- An Ethernet cable or Wi-Fi adapter
Software Requirements
You will also need the following software:
- A Linux distribution for your host computer (e.g., Ubuntu, Fedora, etc.)
- A cross-compiler toolchain for your target architecture
- A bootloader (e.g., U-Boot)
- A build system (e.g., Buildroot, Yocto Project)
Setting Up the Development Environment
- Install a Linux distribution on your host computer.
- Install the necessary packages for embedded development (e.g., build-essential, git, etc.).
- Download and install the cross-compiler toolchain for your target architecture.
- Download and install the bootloader and build system of your choice.
Building an Embedded Linux System
Configuring the Kernel
The Linux kernel is the core of the embedded Linux system. To configure the kernel for your specific needs, you can use the menuconfig tool:
make menuconfig
This will open a graphical interface where you can select the desired options for your kernel.
Compiling the Kernel
Once you have configured the kernel, you can compile it using the following command:
make zImage
This will generate a compressed kernel image (zImage) that can be loaded by the bootloader.
Creating a Root Filesystem
The root filesystem contains the necessary files and directories for the embedded Linux system to function. You can create a root filesystem using a build system like Buildroot or Yocto Project.
For example, to create a root filesystem using Buildroot:
make menuconfig
make
This will generate a root filesystem image that can be loaded by the bootloader.
Configuring the Bootloader
The bootloader is responsible for initializing the hardware and loading the kernel and root filesystem. To configure the bootloader, you can use the menuconfig tool:
make menuconfig
This will open a graphical interface where you can select the desired options for your bootloader.
Flashing the Images
Once you have generated the kernel and root filesystem images, you need to flash them onto the embedded device. The specific steps will depend on your development board and bootloader.
For example, to flash the images using U-Boot on a Raspberry Pi:
- Copy the kernel and root filesystem images to the SD card.
- Insert the SD card into the Raspberry Pi.
- Power on the Raspberry Pi and interrupt the boot process by pressing a key.
- Load the kernel image:
load mmc 0:1 ${kernel_addr_r} zImage
- Load the root filesystem image:
load mmc 0:2 ${fdt_addr_r} rootfs.img
- Boot the system:
bootz ${kernel_addr_r} ${fdt_addr_r}
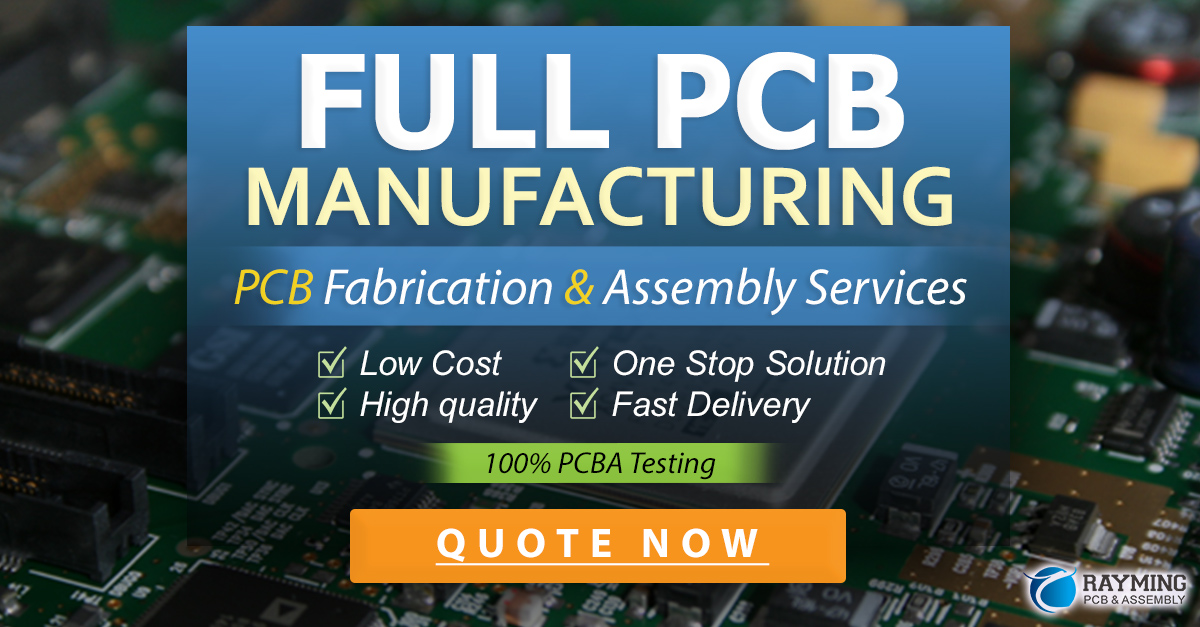
Debugging Embedded Linux Systems
Debugging is an essential part of embedded Linux development. There are several tools and techniques you can use to debug your embedded Linux system.
Kernel Debugging
To debug the Linux kernel, you can use the printk function to output messages to the console:
printk(KERN_INFO "Hello, world!\n");
You can also use the gdb debugger to debug the kernel:
gdb vmlinux
This will open the gdb debugger, allowing you to set breakpoints, inspect variables, and step through the kernel code.
Application Debugging
To debug user-space applications, you can use the gdb debugger:
gdb app
This will open the gdb debugger, allowing you to set breakpoints, inspect variables, and step through the application code.
You can also use the strace tool to trace system calls made by an application:
strace app
This will output a trace of all system calls made by the application, which can be useful for debugging.
Remote Debugging
To debug an embedded Linux system remotely, you can use the gdbserver tool:
gdbserver :2345 app
This will start the gdbserver on the embedded device, allowing you to connect to it from your host computer using the gdb debugger:
gdb app
target remote 192.168.1.100:2345
This will connect the gdb debugger to the gdbserver running on the embedded device, allowing you to debug the application remotely.
Advanced Topics
Real-Time Linux
Real-time Linux is a variant of the Linux kernel that provides deterministic performance for real-time applications. It is commonly used in industrial control systems, robotics, and other applications that require precise timing.
To use real-time Linux, you need to patch the Linux kernel with the PREEMPT_RT patch and configure it for real-time operation.
Device Drivers
Device drivers are kernel modules that provide an interface between the hardware and the operating system. To develop a device driver for embedded Linux, you need to have a good understanding of the Linux device model and the kernel API.
Some key concepts to understand when developing device drivers include:
- Device registration and discovery
- Interrupt handling
- Memory mapping
- Direct memory access (DMA)
- Synchronization primitives (e.g., spinlocks, mutexes)
System Optimization
Embedded Linux systems often have limited resources, so it is important to optimize the system for performance and resource usage. Some techniques for optimizing embedded Linux systems include:
- Reducing the size of the kernel and root filesystem
- Optimizing the application code for performance
- Using a real-time operating system (RTOS) for time-critical tasks
- Offloading tasks to dedicated hardware (e.g., DSP, FPGA)
- Using power management techniques to reduce power consumption
Frequently Asked Questions (FAQ)
-
What is the difference between embedded Linux and desktop Linux?
Embedded Linux is a stripped-down version of the Linux kernel, tailored to the specific requirements of embedded devices. It is optimized for resource-constrained environments and often lacks the graphical user interface and other features found in desktop Linux distributions. -
Can I use embedded Linux on any hardware?
Embedded Linux can be used on a wide range of hardware platforms, from small microcontrollers to powerful system-on-chips (SoCs). However, the specific hardware requirements will depend on the application and the desired features. -
What programming languages can I use with embedded Linux?
You can use any programming language that can be compiled for the target architecture, including C, C++, Python, and Java. However, C and C++ are the most commonly used languages for embedded Linux development due to their performance and low-level access to hardware. -
How do I choose a build system for embedded Linux?
The choice of build system will depend on your specific requirements and preferences. Some popular build systems for embedded Linux include Buildroot, Yocto Project, and OpenEmbedded. Each build system has its own strengths and weaknesses, so it is important to evaluate them based on your needs. -
What are some common challenges in embedded Linux development?
Some common challenges in embedded Linux development include: - Limited resources (e.g., memory, storage, processing power)
- Diverse hardware platforms and architectures
- Real-time performance requirements
- Security and reliability concerns
- Long-term maintenance and support
Conclusion
Embedded Linux is a powerful and flexible platform for developing embedded systems. This tutorial has covered the basics of embedded Linux, including hardware and software requirements, building an embedded Linux system, debugging techniques, and advanced topics like real-time Linux and device drivers.
By following the steps outlined in this tutorial, you should be able to get started with embedded Linux development and create custom embedded systems tailored to your specific needs.
Topic | Description |
---|---|
Introduction | Overview of embedded Linux and its advantages |
Getting Started | Hardware and software requirements, setting up the development environment |
Building the System | Configuring and compiling the kernel, creating a root filesystem, configuring the bootloader, flashing the images |
Debugging | Kernel debugging, application debugging, remote debugging |
Advanced Topics | Real-time Linux, device drivers, system optimization |
Frequently Asked Questions | Common questions and answers about embedded Linux development |
Remember, embedded Linux development is a complex and ongoing process. It requires a deep understanding of the Linux kernel, hardware platforms, and software development techniques. However, with the right tools, knowledge, and perseverance, you can create powerful and efficient embedded systems using Linux.
Leave a Reply