Introduction to Atmega2560
The Atmega2560 is a powerful 8-bit microcontroller developed by Microchip Technology (formerly Atmel). It is part of the AVR family of microcontrollers and is widely used in various applications, including robotics, automation, and embedded systems. This manual provides a comprehensive guide to understanding and working with the Atmega2560 microcontroller.
Key Features of Atmega2560
The Atmega2560 offers a wide range of features that make it suitable for diverse projects. Some of its key features include:
- 256 KB of In-System Programmable Flash memory
- 8 KB of SRAM
- 4 KB of EEPROM
- 86 General Purpose I/O pins
- 16 PWM channels
- 16 Analog-to-Digital Converter (ADC) channels
- 4 UARTs (Universal Asynchronous Receiver/Transmitter)
- 2 USARTs (Universal Synchronous/Asynchronous Receiver/Transmitter)
- 1 SPI (Serial Peripheral Interface)
- 1 TWI (Two-Wire Interface)
- Real-time Counter (RTC)
- 6 Timer/Counters with compare modes
Atmega2560 Pin Configuration
The Atmega2560 comes in a 100-pin TQFP (Thin Quad Flat Pack) package. The following table provides an overview of the pin configuration:
Pin Range | Description |
---|---|
1-22 | Port A (PA0-PA7), Port B (PB0-PB7), Port C (PC0-PC7) |
23-53 | Port D (PD0-PD7), Port E (PE0-PE7), Port F (PF0-PF7), Port G (PG0-PG5) |
54-61 | Port H (PH0-PH7) |
62-69 | Port J (PJ0-PJ7) |
70-77 | Port K (PK0-PK7) |
78-85 | Port L (PL0-PL7) |
86-93 | ADC Inputs (ADC0-ADC15) |
94-100 | Power and Ground |
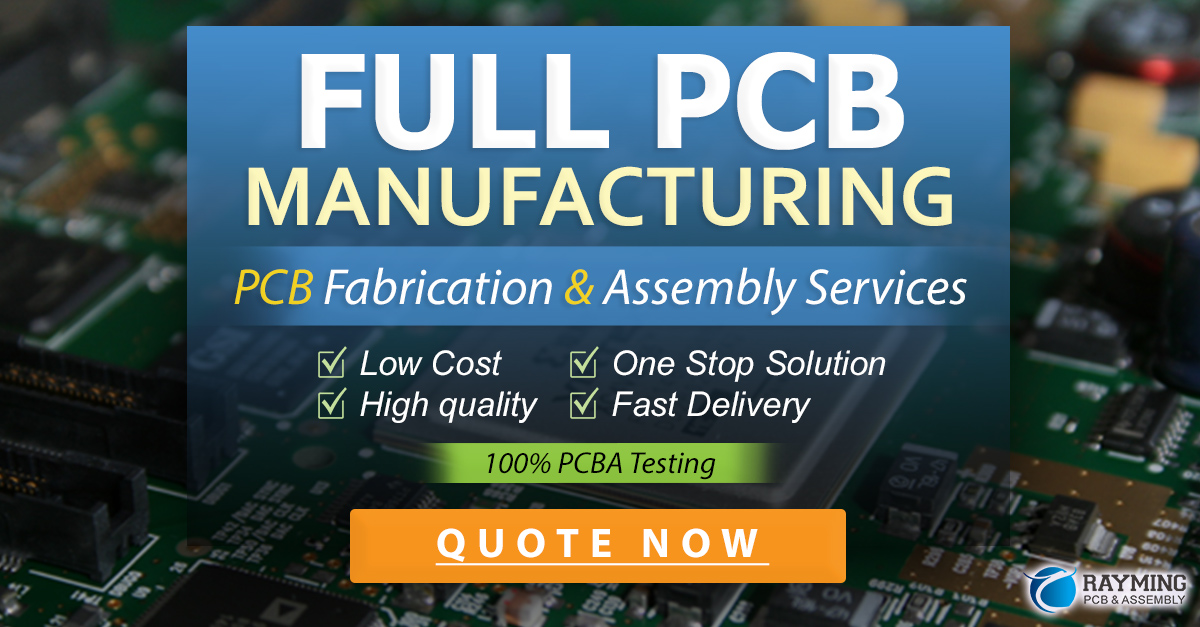
Setting Up the Development Environment
To start working with the Atmega2560, you need to set up a development environment. Here are the steps to follow:
-
Install Atmel Studio: Atmel Studio is an Integrated Development Environment (IDE) provided by Microchip for developing AVR and SAM microcontroller applications. Download and install the latest version of Atmel Studio from the official website.
-
Connect the Atmega2560 to your computer: You can use a development board like Arduino Mega 2560 or a standalone Atmega2560 chip with a programming interface such as ISP (In-System Programming) or JTAG (Joint Test Action Group).
-
Configure the programmer: In Atmel Studio, go to “Tools” > “Device Programming” and select the appropriate programmer based on your setup (e.g., “Atmel-ICE” for ISP or “JTAG” for JTAG programming).
-
Create a new project: Go to “File” > “New” > “Project” and select “GCC C Executable Project” for AVR. Choose the Atmega2560 as the target device and specify the project name and location.
Programming the Atmega2560
The Atmega2560 can be programmed using the C programming language. Atmel Studio provides a comprehensive environment for writing, compiling, and uploading code to the microcontroller. Here’s a basic example of an LED blinking program:
#include <avr/io.h>
#include <util/delay.h>
#define LED_PIN PB7
int main(void)
{
DDRB |= (1 << LED_PIN); // Set LED_PIN as output
while (1)
{
PORTB ^= (1 << LED_PIN); // Toggle LED_PIN
_delay_ms(1000); // Delay for 1 second
}
return 0;
}
To compile and upload the code:
- Open the project in Atmel Studio.
- Click on the “Build” button or go to “Build” > “Build Solution” to compile the code.
- If there are no errors, click on the “Debug” button or go to “Debug” > “Start Debugging and Break” to upload the code to the Atmega2560.
Atmega2560 Peripheral Interfaces
The Atmega2560 provides various peripheral interfaces for communication and interaction with external devices. Some of the commonly used interfaces include:
UART (Universal Asynchronous Receiver/Transmitter)
UART is used for serial communication between the Atmega2560 and other devices. It allows asynchronous data transmission and reception. The Atmega2560 has four UART modules: UART0, UART1, UART2, and UART3.
To use UART, you need to initialize the UART module with the desired baud rate, data bits, stop bits, and parity. Here’s an example of UART initialization:
#define BAUD 9600
#define F_CPU 16000000UL
void uart_init(void)
{
UBRR0H = (F_CPU / (16UL * BAUD)) >> 8;
UBRR0L = (F_CPU / (16UL * BAUD)) & 0xFF;
UCSR0B = (1 << RXEN0) | (1 << TXEN0);
UCSR0C = (1 << UCSZ01) | (1 << UCSZ00);
}
To transmit data over UART, you can use the uart_putchar
function:
void uart_putchar(char data)
{
while (!(UCSR0A & (1 << UDRE0)));
UDR0 = data;
}
To receive data, you can use the uart_getchar
function:
char uart_getchar(void)
{
while (!(UCSR0A & (1 << RXC0)));
return UDR0;
}
I2C (Inter-Integrated Circuit)
I2C, also known as TWI (Two-Wire Interface), is a synchronous serial communication protocol used for communication between the Atmega2560 and other I2C-compatible devices. It uses two lines: SCL (Serial Clock) and SDA (Serial Data).
To use I2C, you need to initialize the TWI module and set the SCL frequency. Here’s an example of TWI initialization:
#define F_CPU 16000000UL
#define TWI_FREQ 100000UL
void twi_init(void)
{
TWBR = ((F_CPU / TWI_FREQ) - 16) / 2;
TWSR = 0;
}
To transmit data over I2C, you can use the twi_start
, twi_write
, and twi_stop
functions:
void twi_start(uint8_t address)
{
TWCR = (1 << TWINT) | (1 << TWSTA) | (1 << TWEN);
while (!(TWCR & (1 << TWINT)));
TWDR = address;
TWCR = (1 << TWINT) | (1 << TWEN);
while (!(TWCR & (1 << TWINT)));
}
void twi_write(uint8_t data)
{
TWDR = data;
TWCR = (1 << TWINT) | (1 << TWEN);
while (!(TWCR & (1 << TWINT)));
}
void twi_stop(void)
{
TWCR = (1 << TWINT) | (1 << TWSTO) | (1 << TWEN);
}
To receive data, you can use the twi_read
function:
uint8_t twi_read(uint8_t ack)
{
TWCR = (1 << TWINT) | (1 << TWEN) | (ack ? (1 << TWEA) : 0);
while (!(TWCR & (1 << TWINT)));
return TWDR;
}
SPI (Serial Peripheral Interface)
SPI is a synchronous serial communication protocol used for communication between the Atmega2560 and other SPI-compatible devices. It uses four lines: MOSI (Master Out Slave In), MISO (Master In Slave Out), SCK (Serial Clock), and SS (Slave Select).
To use SPI, you need to initialize the SPI module and set the desired clock frequency and mode. Here’s an example of SPI initialization:
void spi_init(void)
{
DDRB |= (1 << PB2) | (1 << PB3) | (1 << PB5);
SPCR = (1 << SPE) | (1 << MSTR) | (1 << SPR0);
}
To transmit and receive data over SPI, you can use the spi_transfer
function:
uint8_t spi_transfer(uint8_t data)
{
SPDR = data;
while (!(SPSR & (1 << SPIF)));
return SPDR;
}
Analog-to-Digital Converter (ADC)
The Atmega2560 features a 10-bit Analog-to-Digital Converter (ADC) with 16 channels. It allows you to convert analog signals into digital values for further processing.
To use the ADC, you need to initialize the ADC module and select the desired reference voltage and prescaler. Here’s an example of ADC initialization:
void adc_init(void)
{
ADMUX = (1 << REFS0);
ADCSRA = (1 << ADEN) | (1 << ADPS2) | (1 << ADPS1) | (1 << ADPS0);
}
To read the ADC value from a specific channel, you can use the adc_read
function:
uint16_t adc_read(uint8_t channel)
{
ADMUX = (ADMUX & 0xF0) | (channel & 0x0F);
ADCSRA |= (1 << ADSC);
while (ADCSRA & (1 << ADSC));
return ADC;
}
Pulse Width Modulation (PWM)
PWM is a technique used to generate analog-like signals using digital means. The Atmega2560 provides 16 PWM channels that can be used for controlling the brightness of LEDs, motor speed, and other applications.
To use PWM, you need to configure the Timer/Counter module associated with the PWM channel. Here’s an example of PWM initialization and usage:
void pwm_init(void)
{
DDRB |= (1 << PB7);
TCCR1A = (1 << COM1A1) | (1 << WGM11);
TCCR1B = (1 << WGM13) | (1 << WGM12) | (1 << CS11);
ICR1 = 65535;
}
void pwm_set_duty(uint16_t duty)
{
OCR1A = duty;
}
In this example, PWM is generated on the PB7 pin using Timer/Counter 1. The pwm_set_duty
function can be used to set the duty cycle of the PWM signal.
Interrupts
Interrupts are a way to handle events or conditions that require immediate attention from the microcontroller. The Atmega2560 provides various interrupt sources, including external interrupts, timer interrupts, and peripheral interrupts.
To use interrupts, you need to enable the desired interrupt source and define the corresponding interrupt service routine (ISR). Here’s an example of enabling and handling an external interrupt:
#include <avr/interrupt.h>
ISR(INT0_vect)
{
// Interrupt service routine code
}
void interrupt_init(void)
{
EICRA = (1 << ISC01) | (1 << ISC00);
EIMSK = (1 << INT0);
sei();
}
In this example, the INT0 external interrupt is enabled and configured to trigger on a rising edge. The ISR(INT0_vect)
function is called whenever the interrupt occurs.
Frequently Asked Questions (FAQ)
- What is the difference between Atmega2560 and Arduino Mega 2560?
-
The Atmega2560 is the microcontroller chip used on the Arduino Mega 2560 board. Arduino Mega 2560 is a development board that provides additional features and simplifies the usage of the Atmega2560 by offering a standardized form factor and peripheral connections.
-
Can I program the Atmega2560 using Arduino IDE?
-
Yes, you can program the Atmega2560 using the Arduino IDE. The Arduino IDE provides built-in support for the Arduino Mega 2560 board, which uses the Atmega2560 microcontroller. You can write code in the Arduino language (based on C/C++) and upload it to the Atmega2560 using the Arduino IDE.
-
What is the maximum clock frequency of the Atmega2560?
-
The Atmega2560 can operate at a maximum clock frequency of 16 MHz when powered with 5V. However, it can also operate at lower clock frequencies to reduce power consumption.
-
How much Flash memory and SRAM does the Atmega2560 have?
-
The Atmega2560 has 256 KB of Flash memory for program storage and 8 KB of SRAM for data storage during program execution.
-
Can I use the Atmega2560 for standalone projects without an Arduino board?
- Yes, you can use the Atmega2560 as a standalone microcontroller in your projects. You need to provide the necessary power supply, clock source, and other required circuitry. You can program the Atmega2560 using a programmer like Atmel-ICE or AVRISP mkII.
Conclusion
The Atmega2560 is a versatile and powerful microcontroller that offers a wide range of features and peripherals. This manual has provided an overview of the Atmega2560, including its pin configuration, development environment setup, programming basics, peripheral interfaces, and frequently asked questions.
By understanding the capabilities and functionalities of the Atmega2560, you can create diverse projects and applications in the fields of robotics, automation, embedded systems, and more. With its extensive documentation and community support, the Atmega2560 is a popular choice among hobbyists, students, and professionals alike.
Remember to refer to the official Atmega2560 datasheet and application notes for more detailed information and specific use cases. Happy coding and exploring with the Atmega2560!
Leave a Reply