Introduction to Arduino Speakers
An Arduino speaker is a fun and educational project that allows you to play sounds and music using an Arduino Microcontroller board. By connecting a small speaker or piezo element to the Arduino, you can program it to generate tones and play audio files. Building an Arduino speaker is a great way to learn about electronics, programming, and the basics of digital audio.
In this article, we’ll walk through the process of building a simple Arduino speaker step-by-step. We’ll cover the necessary components, wiring diagram, Arduino code, and some ideas for expanding the project further. Whether you’re an Arduino beginner or have some experience, this guide will help you create your own functional Arduino speaker.
Components Needed for an Arduino Speaker
To build an Arduino speaker, you will need the following components:
Component | Description |
---|---|
Arduino board | The microcontroller that will run the speaker code. An Arduino Uno is a good choice for beginners. |
Breadboard | Used to prototype the circuit without soldering. |
Jumper wires | Used to make connections between components on the breadboard. |
Speaker or piezo element | The actual sound-producing component. A small 8 ohm speaker or piezo buzzer works well. |
220 ohm resistor | Limits current to the speaker to prevent damage. |
USB cable | For powering the Arduino and uploading code from your computer. |
You may also want some optional components for expanding the project:
- Potentiometer for adjusting volume
- Buttons or switches for triggering different sounds
- SD card module for playing audio files
- Battery pack for making the speaker portable
Wiring the Arduino Speaker Circuit
Once you have gathered the necessary components, it’s time to wire up the Arduino speaker circuit. Follow these steps:
- Place the Arduino board and breadboard in front of you.
- Insert the 220 ohm resistor into the breadboard.
- Connect one leg of the resistor to Arduino digital pin 8 using a jumper wire. This will be the pin that sends the audio signal.
- Connect the other leg of the resistor to one of the speaker terminals.
- Connect the other speaker terminal to Arduino GND (ground) using another jumper wire.
Here is a wiring diagram illustrating these connections:
[Insert wiring diagram image]
Double check that your wiring matches the diagram before proceeding. Incorrect connections could damage the components.
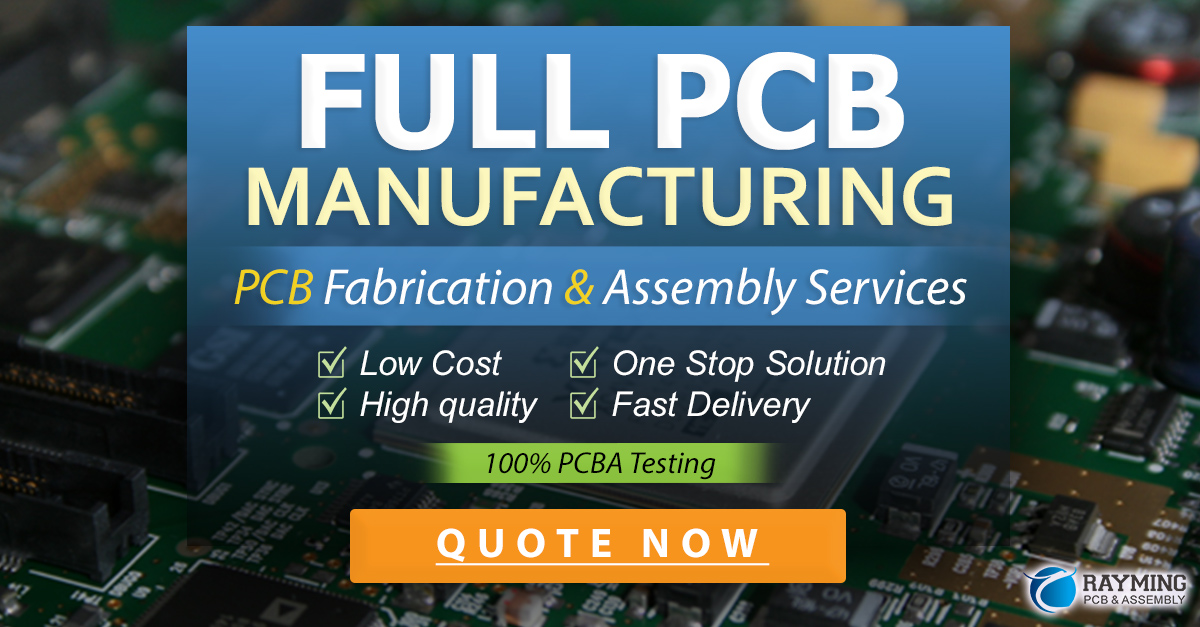
Programming the Arduino Speaker
With the circuit wired up, you can now program the Arduino to generate sounds through the speaker. The Arduino IDE (Integrated Development Environment) is used to write code and upload it to the Arduino board.
Installing the Arduino IDE
- Download the Arduino IDE from the official website: https://www.arduino.cc/en/software
- Install the IDE by running the downloaded file and following the prompts.
- Open the Arduino IDE.
Uploading the Speaker Code
- Connect the Arduino to your computer using the USB cable.
- In the Arduino IDE, go to File > New to create a new sketch.
- Copy and paste the following code into the sketch:
int speakerPin = 8;
void setup() {
pinMode(speakerPin, OUTPUT);
}
void loop() {
tone(speakerPin, 440, 500);
delay(1000);
tone(speakerPin, 880, 500);
delay(1000);
}
- Go to Tools > Board and select your Arduino board type.
- Go to Tools > Port and select the port your Arduino is connected to.
- Click the Upload button (arrow icon) to compile the code and upload it to the Arduino.
If the upload is successful, the Arduino should start playing two alternating tones through the speaker. One tone has a frequency of 440 Hz (musical note A4) and the other is 880 Hz (one octave higher). Each tone plays for 500 ms with a 1000 ms delay in between.
Understanding the Speaker Code
Let’s break down the code to understand how it works:
int speakerPin = 8;
declares a variable to store the number of the pin connected to the speaker (8 in our wiring).- The
setup()
function runs once when the Arduino powers on. It sets the specified pin as an OUTPUT usingpinMode()
. - The
loop()
function runs continuously aftersetup()
finishes. tone(pin, frequency, duration)
generates a square wave of the given frequency on the specified pin for the specified duration in milliseconds. The frequency determines the pitch of the sound.delay(ms)
pauses the program for the specified number of milliseconds.
So this code plays a tone, waits 1 second, plays a higher tone, waits 1 second, then repeats forever. You can change the frequency
and duration
arguments passed to tone()
to create different sounds and patterns.
Expanding the Arduino Speaker
Once you have the basic speaker working, there are many ways you can expand the project:
Adjustable Volume
To adjust the speaker volume, you can connect a potentiometer and use it to control the amplitude of the tones. Wire the potentiometer like this:
- Outside legs to Arduino 5V and GND
- Middle leg to an analog input pin (e.g. A0)
Then add this code at the top of your sketch to read the potentiometer:
int volumePin = A0;
int volume = 0;
void setup() {
...
pinMode(volumePin, INPUT);
}
void loop() {
volume = analogRead(volumePin) / 4;
...
}
And change the tone()
lines to use the volume
variable to scale the duration:
tone(speakerPin, 440, volume);
Now rotating the potentiometer knob will change the tone duration, giving a volume adjustment effect.
Playing Melodies
You can program more complex sequences of tones to play musical melodies. For example, here is code to play the first line of “Twinkle Twinkle Little Star”:
int melody[] = {262, 262, 393, 393, 440, 440, 393};
int noteDurations[] = {4, 4, 4, 4, 4, 4, 2};
void loop() {
for (int i = 0; i < 7; i++) {
int duration = 1000 / noteDurations[i];
tone(speakerPin, melody[i], duration);
delay(duration * 1.30);
}
delay(2000);
}
This uses two arrays to store the frequencies and relative durations of the notes in the melody. The for
loop plays each note in sequence.
Audio File Playback
For playing higher quality audio like speech, music and sound effects, you can use an SD card module and the TMRpcm library. See this tutorial for a guide on setting that up.
Triggering Sounds
To make the speaker interactive, you can connect buttons, switches or sensors to trigger different sounds. For example, a simple button could be used to play a doorbell sound. See this tutorial for how to wire and program a button.
Troubleshooting Tips
If your Arduino speaker is not working as expected, here are some things to check:
- Make sure the wiring matches the diagram, with the correct resistor value and pin connections.
- Check that the Arduino is connected to the computer and the correct board and port are selected in the IDE.
- Verify that the code compiles and uploads without errors.
- Test the speaker on its own by connecting it directly to a 5V power source and GND. It should make a small popping sound.
- Try simplifying the code to just play one tone to isolate issues.
- Check the Arduino IDE Serial Monitor for debugging output statements.
- Search Arduino forums and online communities for others who have encountered similar problems and solutions.
Conclusion
Congratulations, you now know how to build and program a basic Arduino speaker! By following the wiring diagram and uploading the example code, you can quickly get it playing tones. Expanding the project with volume control, melodies, audio files and interactivity provides even more possibilities for creating interesting sound projects.
Building an Arduino speaker is a great introduction to the world of Arduino and electronic music. The skills and concepts learned – like working with a breadboard, controlling a digital output pin, and generating frequencies – apply to many other Arduino projects as well. So have fun experimenting with different sounds and setups, and see what else you can create!
FAQs
What other speakers can I use besides a piezo buzzer?
You can use any 8 ohm speaker that can physically connect to the breadboard or Arduino. Salvaged speakers from old headphones or toys work well. Larger speakers will be louder but require an external amplifier.
How can I make the speaker louder?
The piezo buzzer used is quite small and not very loud. Using a larger speaker or adding an amplifier circuit will increase the volume. You can also try increasing the value of the resistor to allow more current to the speaker, but be careful not to exceed its power rating.
Can I use the Arduino tone() function for playing music?
The tone()
function is limited in terms of sound quality and polyphony (multiple simultaneous tones). It is okay for simple melodies but for more advanced music playback, look into using a dedicated audio shield or module such as the Adafruit Wave Shield.
What is the purpose of the 220 ohm resistor?
The resistor limits the current flowing to the speaker to a safe level. Without it, too much current could overload and damage the speaker. The resistor value is chosen based on the specifications of the particular speaker used.
Can I make the Arduino speaker wireless?
Yes, you can make the Arduino speaker wireless by using a Bluetooth or Wi-Fi module to receive audio data and control signals wirelessly from a remote device. The module would connect to the Arduino’s serial pins. Smartphone apps can be used to send audio to the Arduino speaker over Bluetooth.
Leave a Reply