Introduction to 4×4 Keypads
A 4×4 keypad is a matrix-style input device commonly used in electronic projects, such as microcontroller-based systems, to provide a user-friendly interface for data entry. The keypad consists of 16 buttons arranged in a 4×4 grid, allowing users to input numbers, letters, and symbols. This comprehensive guide will explore the fundamentals of 4×4 keypads, their working principles, and how to integrate them into your projects.
Understanding the 4×4 Keypad Layout
The 4×4 keypad features 16 buttons arranged in four rows and four columns. Each button is labeled with a unique character or symbol, typically including numbers 0-9, letters A-D, and symbols like ‘*’ and ‘#’. The keypad layout is as follows:
1 | 2 | 3 | A |
---|---|---|---|
4 | 5 | 6 | B |
7 | 8 | 9 | C |
* | 0 | # | D |
This arrangement allows for easy and intuitive data entry, making 4×4 keypads a popular choice for various applications.
How 4×4 Keypads Work
Matrix Scanning
4×4 keypads work on the principle of matrix scanning. The keypad has eight pins: four rows and four columns. Each button is connected to a unique combination of a row and a column pin. When a button is pressed, it establishes an electrical connection between the corresponding row and column.
To detect button presses, the microcontroller performs a process called matrix scanning. It follows these steps:
- Set all column pins as outputs and all row pins as inputs with pull-up resistors enabled.
- Set one column pin to LOW and the others to HIGH.
- Read the state of each row pin. If a row pin is LOW, the button at the intersection of the active column and row is pressed.
- Repeat steps 2 and 3 for each column pin.
By systematically scanning the keypad matrix, the microcontroller can determine which button is pressed at any given time.
Debouncing
When a button is pressed or released, mechanical contacts may bounce, causing multiple transitions between HIGH and LOW states. This phenomenon, known as contact bouncing, can lead to false readings and unintended behavior. To mitigate this issue, a technique called debouncing is employed.
Debouncing can be implemented in hardware or software:
- Hardware debouncing: A capacitor is added in parallel with each button to smooth out the transitions and eliminate bouncing.
- Software debouncing: The microcontroller waits for a short period (typically a few milliseconds) after detecting a button press to allow the signal to stabilize before registering the input.
Implementing proper debouncing ensures reliable and accurate keypad input.
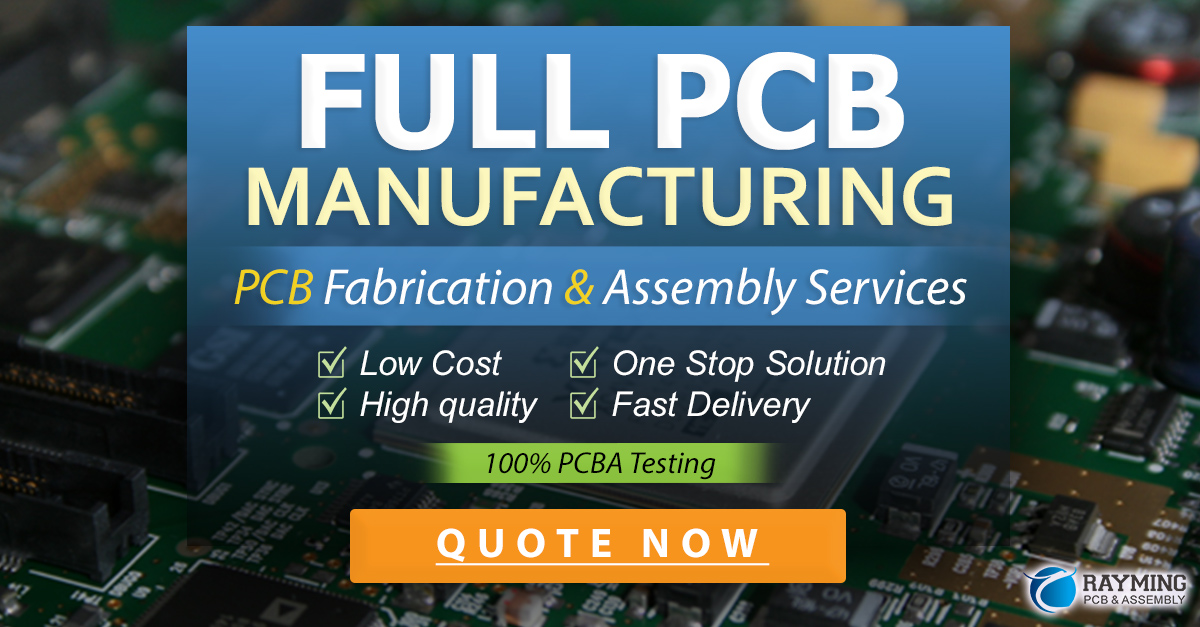
Interfacing a 4×4 Keypad with a Microcontroller
To interface a 4×4 keypad with a microcontroller, such as an Arduino, you need to make the necessary connections and write the appropriate code.
Wiring the Keypad
The 4×4 keypad typically has eight pins: four row pins and four column pins. Connect these pins to the digital I/O pins of your microcontroller as follows:
- Row pins: Connect to digital input pins with pull-up resistors enabled.
- Column pins: Connect to digital output pins.
Consult the keypad’s datasheet or pinout diagram for the specific pin assignments.
Writing the Keypad Library
To simplify the usage of the 4×4 keypad, you can create a library that encapsulates the matrix scanning and debouncing functionality. The library should provide functions for initializing the keypad, reading button presses, and returning the corresponding characters.
Here’s a sample code structure for a 4×4 keypad library:
class Keypad {
private:
uint8_t rowPins[4];
uint8_t colPins[4];
char keys[4][4];
public:
Keypad(uint8_t rowPins[4], uint8_t colPins[4], char keys[4][4]);
void init();
char getKey();
};
The library constructor takes arrays for row pins, column pins, and the keypad layout. The init()
function sets up the pins as inputs and outputs, while the getKey()
function performs matrix scanning and returns the pressed key.
Using the Keypad Library
Once you have the keypad library, using it in your sketches becomes straightforward. Here’s an example of how to use the library:
#include "Keypad.h"
uint8_t rowPins[4] = {2, 3, 4, 5};
uint8_t colPins[4] = {6, 7, 8, 9};
char keys[4][4] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
Keypad keypad(rowPins, colPins, keys);
void setup() {
keypad.init();
Serial.begin(9600);
}
void loop() {
char key = keypad.getKey();
if (key != 0) {
Serial.println(key);
}
}
In this example, the keypad is initialized with the specified row pins, column pins, and layout. The loop()
function continuously checks for button presses and prints the corresponding characters to the serial monitor.
Applications of 4×4 Keypads
4×4 keypads find applications in various projects and systems where user input is required. Some common applications include:
- Access control systems: Keypads are used for entering passwords or PINs to grant access to secure areas or devices.
- Calculator projects: Keypads provide a convenient way to input numbers and perform calculations in DIY calculator projects.
- Menu navigation: Keypads can be used to navigate through menus and make selections in embedded systems or user interfaces.
- Data entry: Keypads allow users to input data, such as names, addresses, or measurements, in projects that require user input.
- Gaming controllers: Keypads can be used as input devices for gaming projects, enabling players to control characters or make selections.
The versatility and simplicity of 4×4 keypads make them a valuable addition to many electronic projects.
Troubleshooting Common Issues
When working with 4×4 keypads, you may encounter some common issues. Here are a few troubleshooting tips:
- Keypad not responding: Ensure that the wiring connections are correct and secure. Double-check the pin assignments in your code and the keypad’s datasheet.
- Multiple button presses detected: Implement proper debouncing techniques, either in hardware or software, to eliminate false readings caused by contact bouncing.
- Incorrect characters returned: Verify that the keypad layout in your code matches the actual layout of your keypad. Make sure the characters in the
keys
array correspond to the correct button positions. - Interference with other components: If the keypad is connected to pins shared with other components, such as displays or sensors, ensure that there are no conflicts or signal interference. Use separate pins or consider using a multiplexer if necessary.
- Unresponsive buttons: Inspect the keypad for any physical damage or wear. Check for loose connections or damaged traces on the keypad’s PCB. If a button remains unresponsive, it may need to be replaced.
By addressing these common issues, you can ensure reliable and consistent performance of your 4×4 keypad.
Frequently Asked Questions (FAQ)
-
Q: Can I use a 4×4 keypad with any microcontroller?
A: Yes, 4×4 keypads can be used with various microcontrollers, including Arduino, Raspberry Pi, and others that have digital I/O pins. -
Q: How many pins are required to interface a 4×4 keypad?
A: A 4×4 keypad requires eight pins: four row pins and four column pins. However, you can use techniques like multiplexing to reduce the number of pins needed. -
Q: What is the typical operating voltage of a 4×4 keypad?
A: Most 4×4 keypads operate at a voltage range of 3.3V to 5V, making them compatible with common microcontrollers and development boards. -
Q: Can I customize the keypad layout or use different characters?
A: Yes, you can customize the keypad layout by modifying thekeys
array in your code to match your desired characters or symbols. -
Q: Are there any limitations on the key press speed or responsiveness?
A: The responsiveness of the keypad depends on factors like the scanning rate and debounce delay. By optimizing these parameters, you can achieve fast and responsive key presses. However, the mechanical nature of the buttons may impose some limitations on the maximum speed.
Conclusion
4×4 keypads offer a simple and effective way to add user input capabilities to your electronic projects. By understanding the keypad layout, working principles, and interfacing techniques, you can easily integrate a 4×4 keypad into your designs.
This in-depth guide has covered the fundamentals of 4×4 keypads, including matrix scanning, debouncing, wiring, and library creation. We have also explored various applications and troubleshooting tips to help you make the most of your 4×4 keypad.
With the knowledge gained from this guide, you can confidently incorporate 4×4 keypads into your projects, enabling intuitive and interactive user experiences. Whether you’re building an access control system, a calculator, or a gaming controller, a 4×4 keypad can provide a reliable and versatile input solution.
So, go ahead and experiment with 4×4 keypads in your next project, and unlock the potential of user input in your electronic designs!
Leave a Reply