What is Software Serial Arduino?
Software Serial Arduino is a library that allows you to create additional serial ports on your Arduino board using software instead of relying on the hardware serial ports. It enables serial communication on digital pins other than the default RX and TX pins, providing flexibility in your project design.
With Software Serial Arduino, you can:
– Establish serial communication between your Arduino and other devices
– Create multiple software serial ports on a single Arduino board
– Use any available digital pins for serial communication
– Communicate with devices that require different baud rates or communication protocols
How Does Software Serial Arduino Work?
Software Serial Arduino works by emulating the functionality of a hardware serial port using software. It uses interrupts and precise timing to receive and transmit data through the specified digital pins.
Here’s a basic overview of how Software Serial Arduino operates:
1. Initialization: You create an instance of the SoftwareSerial class, specifying the RX and TX pins you want to use for serial communication.
2. Receiving Data: When data is received on the RX pin, the library triggers an interrupt and stores the incoming data in a buffer.
3. Transmitting Data: When you want to send data, you use the write()
function to send data through the TX pin. The library handles the timing and sends the data bit by bit.
4. Baud Rate: You set the desired baud rate for communication using the begin()
function. The library takes care of the necessary timing calculations.
Setting Up Software Serial Arduino
To start using Software Serial Arduino in your project, you need to follow these steps:
- Include the SoftwareSerial library in your Arduino sketch:
#include <SoftwareSerial.h>
- Create an instance of the SoftwareSerial class, specifying the RX and TX pins:
SoftwareSerial mySerial(2, 3); // RX: Pin 2, TX: Pin 3
- Initialize the software serial port in the
setup()
function:
void setup() {
mySerial.begin(9600); // Set the baud rate
}
- Use the
available()
,read()
, andwrite()
functions to receive and send data:
void loop() {
if (mySerial.available()) {
char data = mySerial.read();
// Process the received data
}
mySerial.write("Hello, Arduino!");
}
That’s it! You’re now ready to use Software Serial Arduino in your project.
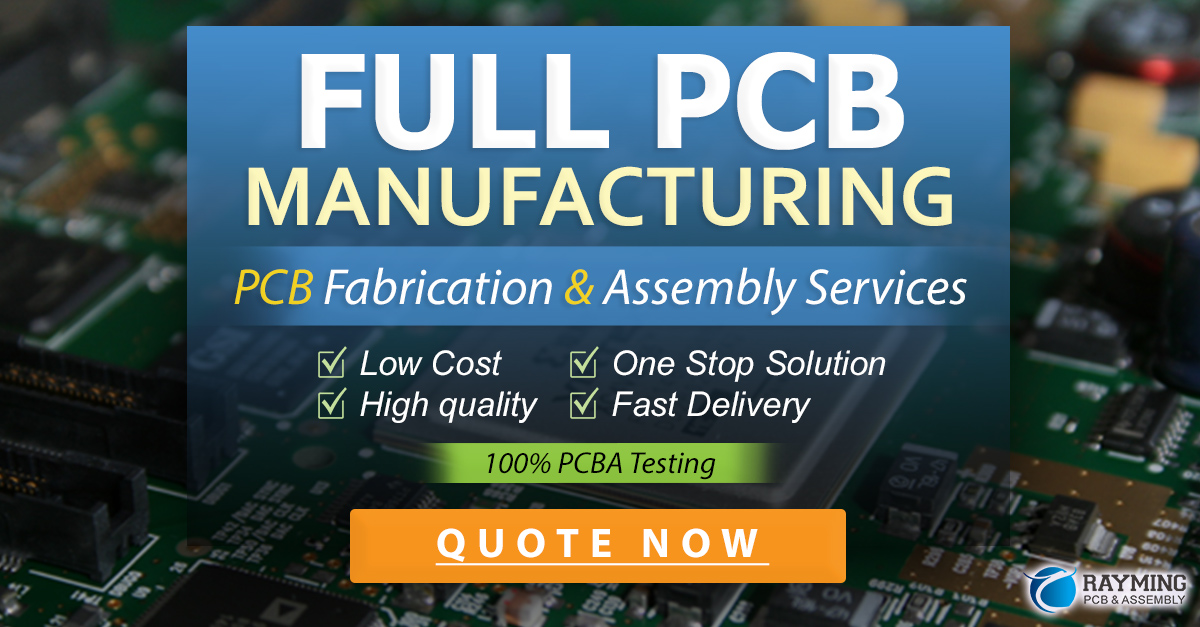
Advantages of Software Serial Arduino
Software Serial Arduino offers several advantages over using the hardware serial ports:
-
Multiple Serial Ports: With Software Serial Arduino, you can create multiple software serial ports on a single Arduino board. This is particularly useful when you need to communicate with multiple devices simultaneously.
-
Flexibility in Pin Selection: Software Serial Arduino allows you to use any available digital pins for serial communication. This gives you more flexibility in designing your project and enables you to optimize pin usage based on your specific requirements.
-
Baud Rate Customization: Software Serial Arduino supports a wide range of baud rates, allowing you to communicate with devices that require different communication speeds. You can easily set the desired baud rate using the
begin()
function. -
Compatibility with Different Protocols: Software Serial Arduino can be used to communicate with devices that use various serial communication protocols, such as UART, RS-232, or even custom protocols. By configuring the appropriate settings, you can establish communication with a wide range of devices.
Limitations of Software Serial Arduino
While Software Serial Arduino offers many benefits, it also has some limitations that you should be aware of:
-
Interrupt-Based: Software Serial Arduino relies on interrupts to receive and transmit data. This means that it can be affected by other interrupt-based libraries or functions in your sketch. If you have time-critical tasks or are using other interrupt-based libraries, you may need to carefully manage the interrupt priorities.
-
Limited Baud Rates: Although Software Serial Arduino supports a wide range of baud rates, it may not be able to achieve the highest baud rates supported by hardware serial ports. The maximum achievable baud rate depends on the Arduino board and the specific pins used for communication.
-
Potential Timing Issues: Since Software Serial Arduino emulates serial communication using software, it relies on precise timing. If your sketch has other time-consuming tasks or delays, it may affect the timing of the software serial communication, leading to potential data loss or corruption.
-
Reduced Performance: Compared to hardware serial communication, Software Serial Arduino may have slightly reduced performance due to the software overhead involved. If you require high-speed or low-latency communication, using hardware serial ports may be more suitable.
Tips for Using Software Serial Arduino
To make the most of Software Serial Arduino and ensure reliable communication, consider the following tips:
-
Choose Appropriate Pins: Select digital pins that are not used by other functions or libraries in your sketch. Avoid using pins that have special functions, such as PWM or interrupt pins, unless necessary.
-
Manage Interrupt Priorities: If you are using other interrupt-based libraries or functions in your sketch, make sure to manage the interrupt priorities appropriately. You may need to adjust the priorities to ensure that the software serial communication is not disrupted.
-
Use Adequate Delays: When sending data using Software Serial Arduino, it’s important to include adequate delays between transmissions to allow the receiving device to process the data. The specific delay required depends on the baud rate and the processing speed of the receiving device.
-
Handle Data Efficiently: When receiving data using Software Serial Arduino, process the data efficiently to avoid buffer overflow. Read the available data promptly and handle it accordingly to prevent data loss.
-
Consider Hardware Serial Ports: If your project requires high-speed or low-latency communication, consider using the hardware serial ports instead of Software Serial Arduino. Hardware serial ports offer better performance and are less prone to timing issues.
Examples and Use Cases
Software Serial Arduino finds applications in a wide range of projects and scenarios. Here are a few examples and use cases:
-
Communicating with GPS Modules: Software Serial Arduino can be used to establish communication between an Arduino board and a GPS module. By connecting the GPS module to the software serial pins, you can receive GPS data and process it in your sketch.
-
Interfacing with Bluetooth Modules: With Software Serial Arduino, you can easily interface your Arduino board with Bluetooth modules, such as the HC-05 or HC-06. This enables wireless communication between your Arduino and other Bluetooth-enabled devices, such as smartphones or computers.
-
Controlling LCD Displays: Software Serial Arduino can be used to control LCD displays that use serial communication protocols, such as the I2C or SPI. By connecting the LCD display to the software serial pins, you can send commands and display data on the screen.
-
Communicating with Other Microcontrollers: Software Serial Arduino allows you to establish communication between multiple Arduino boards or other microcontrollers. By connecting the TX pin of one board to the RX pin of another, you can exchange data and synchronize actions between the devices.
Frequently Asked Questions (FAQ)
-
Q: Can I use Software Serial Arduino with any Arduino board?
A: Yes, Software Serial Arduino is compatible with most Arduino boards, including Arduino Uno, Arduino Mega, Arduino Nano, and others. -
Q: How many software serial ports can I create on an Arduino board?
A: The number of software serial ports you can create depends on the available memory and processing power of your Arduino board. Typically, you can create multiple software serial ports, but keep in mind that each port consumes resources and may impact overall performance. -
Q: Can I use Software Serial Arduino and hardware serial ports simultaneously?
A: Yes, you can use both software serial ports and hardware serial ports simultaneously on an Arduino board. Just make sure to use different pins for each serial port to avoid conflicts. -
Q: What is the maximum baud rate supported by Software Serial Arduino?
A: The maximum baud rate supported by Software Serial Arduino varies depending on the Arduino board and the specific pins used for communication. Generally, it is recommended to use baud rates up to 115200 for reliable communication, although higher baud rates may be possible in some cases. -
Q: Can I use Software Serial Arduino for communication with sensors or modules that require specific protocols?
A: Yes, Software Serial Arduino can be used to communicate with sensors or modules that use specific protocols, such as UART, RS-232, or custom protocols. You may need to configure the appropriate settings, such as baud rate, data bits, parity, and stop bits, to match the requirements of the device you are communicating with.
Conclusion
Software Serial Arduino is a powerful tool that expands the serial communication capabilities of your Arduino board. By allowing you to create additional serial ports using software, it provides flexibility and versatility in your project design. Whether you need to communicate with multiple devices, use custom pins for serial communication, or work with devices that require different baud rates or protocols, Software Serial Arduino has you covered.
In this article, we explored the fundamentals of Software Serial Arduino, including its working principle, setup process, advantages, limitations, and tips for effective usage. We also discussed various examples and use cases where Software Serial Arduino can be applied, such as communicating with GPS modules, Bluetooth modules, LCD displays, and other microcontrollers.
By understanding the concepts and techniques presented in this article, you can confidently integrate Software Serial Arduino into your projects and unleash the full potential of serial communication on your Arduino board. Remember to consider the specific requirements of your project, manage interrupt priorities, and handle data efficiently to ensure reliable and smooth communication.
Happy coding and exploring the world of Software Serial Arduino!
Leave a Reply