Introduction to L293D Motor Driver
The L293D is a popular motor driver IC that allows you to control the speed and direction of two DC motors simultaneously. It is widely used in robotics and automation projects due to its simplicity and reliability. In this article, we will explore how to connect the L293D motor driver to a DC motor using an Arduino board.
What is an L293D Motor Driver?
The L293D is a 16-pin IC that can control two DC motors independently. It is based on the H-bridge configuration, which allows the motor to be driven in both clockwise and counterclockwise directions. The L293D can handle a maximum current of 600mA per channel and operates at a voltage range of 4.5V to 36V.
Key Features of L293D Motor Driver
- Dual H-bridge motor driver
- Can control two DC motors independently
- Bidirectional control of motors
- Maximum current capacity of 600mA per channel
- Wide operating voltage range (4.5V to 36V)
- Built-in diodes for protection against back EMF
- Simple interface with microcontrollers like Arduino
Understanding the L293D Pinout
Before we dive into connecting the L293D to a DC motor and Arduino, let’s take a closer look at the pinout of the L293D IC.
Pin Number | Pin Name | Description |
---|---|---|
1 | Enable 1 | Enables motor 1 when HIGH |
2 | Input 1 | Motor 1 input 1 |
3 | Output 1 | Motor 1 output 1 |
4 | GND | Ground |
5 | GND | Ground |
6 | Output 2 | Motor 1 output 2 |
7 | Input 2 | Motor 1 input 2 |
8 | Vcc2 | Supply voltage for motors (up to 36V) |
9 | Enable 2 | Enables motor 2 when HIGH |
10 | Input 3 | Motor 2 input 1 |
11 | Output 3 | Motor 2 output 1 |
12 | GND | Ground |
13 | GND | Ground |
14 | Output 4 | Motor 2 output 2 |
15 | Input 4 | Motor 2 input 2 |
16 | Vcc1 | Supply voltage for logic (5V) |
Connecting L293D to a DC Motor and Arduino
Now that we understand the pinout of the L293D, let’s see how to connect it to a DC motor and Arduino.
Components Required
- L293D motor driver IC
- DC motor
- Arduino board (e.g., Arduino Uno)
- Breadboard
- Jumper wires
- External power supply (up to 36V)
Connection Diagram
Here’s a step-by-step guide on how to connect the L293D to a DC motor and Arduino:
- Connect the Vcc1 pin (pin 16) of the L293D to the 5V pin of the Arduino.
- Connect the Vcc2 pin (pin 8) of the L293D to the positive terminal of the external power supply.
- Connect the GND pins (pins 4, 5, 12, and 13) of the L293D to the GND pin of the Arduino and the negative terminal of the external power supply.
- Connect the Input 1 and Input 2 pins (pins 2 and 7) of the L293D to digital output pins of the Arduino (e.g., pins 2 and 3).
- Connect the Output 1 and Output 2 pins (pins 3 and 6) of the L293D to the terminals of the DC motor.
- Connect the Enable 1 pin (pin 1) of the L293D to a PWM pin of the Arduino (e.g., pin 9) to control the speed of the motor.
Here’s a visual representation of the connection diagram:
[Insert connection diagram image here]
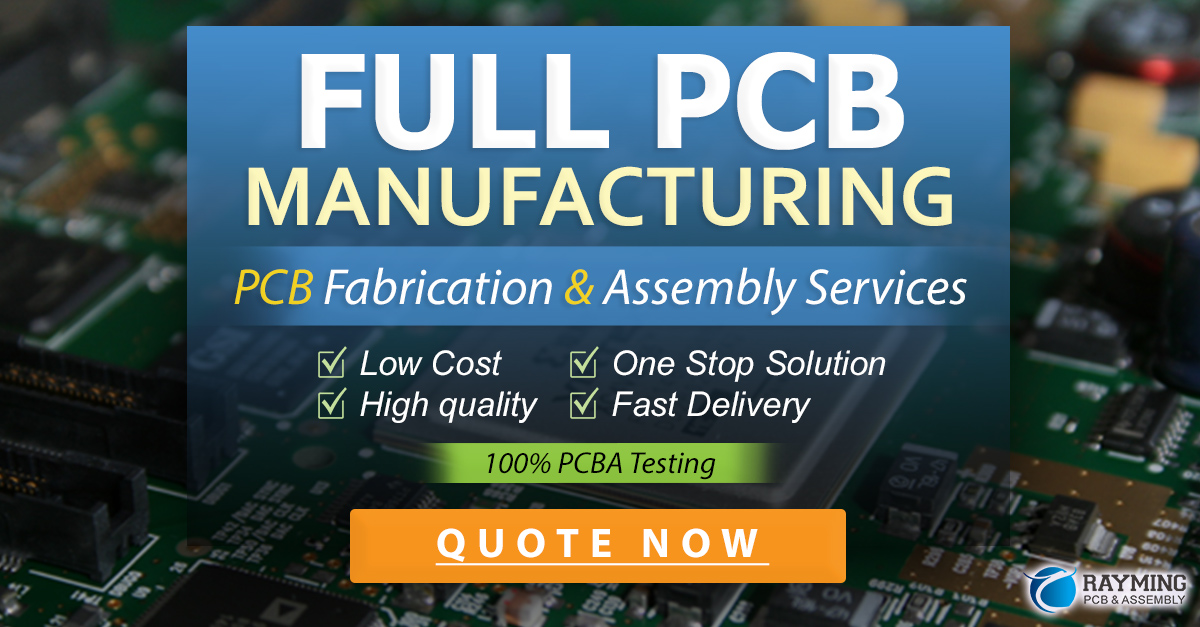
Controlling the DC Motor with Arduino
Now that we have connected the L293D to the DC motor and Arduino, let’s see how to control the motor using Arduino code.
Arduino Code Example
Here’s an example Arduino sketch that demonstrates how to control the speed and direction of the DC motor using the L293D:
const int enablePin = 9;
const int input1Pin = 2;
const int input2Pin = 3;
void setup() {
pinMode(enablePin, OUTPUT);
pinMode(input1Pin, OUTPUT);
pinMode(input2Pin, OUTPUT);
}
void loop() {
// Set motor direction: clockwise
digitalWrite(input1Pin, HIGH);
digitalWrite(input2Pin, LOW);
// Set motor speed: 50% duty cycle
analogWrite(enablePin, 128);
delay(2000); // Run for 2 seconds
// Set motor direction: counterclockwise
digitalWrite(input1Pin, LOW);
digitalWrite(input2Pin, HIGH);
// Set motor speed: 75% duty cycle
analogWrite(enablePin, 192);
delay(2000); // Run for 2 seconds
// Stop the motor
digitalWrite(input1Pin, LOW);
digitalWrite(input2Pin, LOW);
delay(1000); // Pause for 1 second
}
In this code:
– We define the pin numbers for the enable, input1, and input2 pins.
– In the setup()
function, we set the pin modes as OUTPUT.
– In the loop()
function, we control the direction and speed of the motor:
– To set the motor direction, we set input1 and input2 pins to HIGH or LOW accordingly.
– To set the motor speed, we use analogWrite()
on the enable pin with a value between 0 (0% duty cycle) and 255 (100% duty cycle).
– We run the motor in one direction for 2 seconds, then change the direction and run it for another 2 seconds.
– Finally, we stop the motor by setting both input pins to LOW and pause for 1 second.
Upload this sketch to your Arduino board and observe the DC motor’s behavior.
Controlling Multiple Motors with L293D
The L293D can control two DC motors independently. To control multiple motors, you can follow the same connection diagram and code structure as described above, but with additional input and output pins for the second motor.
Connection Diagram for Multiple Motors
[Insert connection diagram for multiple motors here]
Arduino Code Example for Multiple Motors
const int enablePin1 = 9;
const int input1Pin1 = 2;
const int input2Pin1 = 3;
const int enablePin2 = 10;
const int input1Pin2 = 4;
const int input2Pin2 = 5;
void setup() {
pinMode(enablePin1, OUTPUT);
pinMode(input1Pin1, OUTPUT);
pinMode(input2Pin1, OUTPUT);
pinMode(enablePin2, OUTPUT);
pinMode(input1Pin2, OUTPUT);
pinMode(input2Pin2, OUTPUT);
}
void loop() {
// Motor 1: clockwise, 50% speed
digitalWrite(input1Pin1, HIGH);
digitalWrite(input2Pin1, LOW);
analogWrite(enablePin1, 128);
// Motor 2: counterclockwise, 75% speed
digitalWrite(input1Pin2, LOW);
digitalWrite(input2Pin2, HIGH);
analogWrite(enablePin2, 192);
delay(2000); // Run for 2 seconds
// Stop both motors
digitalWrite(input1Pin1, LOW);
digitalWrite(input2Pin1, LOW);
digitalWrite(input1Pin2, LOW);
digitalWrite(input2Pin2, LOW);
delay(1000); // Pause for 1 second
}
In this code, we define the pin numbers for both motors and control them independently using separate enable, input1, and input2 pins.
Tips and Troubleshooting
- Make sure to connect the L293D and DC motor to the appropriate voltage sources. The Vcc1 pin should be connected to the Arduino’s 5V, while the Vcc2 pin should be connected to an external power supply that matches the motor’s voltage rating.
- If the motor is not running, double-check your connections and ensure that the enable pin is receiving a PWM signal.
- If the motor is running in the opposite direction than expected, swap the connections of the output pins to the motor terminals.
- Be mindful of the current consumption of your motors. The L293D can handle up to 600mA per channel. If your motors require more current, consider using an external power supply and a higher-rated motor driver.
Conclusion
The L293D motor driver is a versatile and easy-to-use IC for controlling DC motors with Arduino. By following the connection diagram and example code provided in this article, you can quickly get started with controlling the speed and direction of your motors. Whether you’re building a robot, an automated system, or any other project that requires motor control, the L293D is a reliable choice.
Frequently Asked Questions (FAQ)
-
Can I control stepper motors with the L293D?
No, the L293D is designed specifically for DC motors. To control stepper motors, you would need a dedicated stepper motor driver, such as the A4988 or DRV8825. -
How much current can the L293D handle?
The L293D can handle a maximum current of 600mA per channel. If your motors require more current, you should consider using a higher-rated motor driver or an external power supply. -
Can I control more than two motors with the L293D?
The L293D can control two DC motors independently. If you need to control more than two motors, you can use multiple L293D ICs or consider using a motor driver with more channels, such as the L298N. -
What is the purpose of the enable pin on the L293D?
The enable pin allows you to control the speed of the motor using PWM (Pulse Width Modulation). By applying a PWM signal to the enable pin, you can vary the motor’s speed from 0% to 100%. -
Do I need an external power supply for the L293D?
Yes, it is recommended to use an external power supply to provide sufficient current to the motors. The Vcc2 pin of the L293D should be connected to the positive terminal of the external power supply, while the GND pins should be connected to the negative terminal and the Arduino’s GND.
Leave a Reply