Introduction to the DS1307 Real-Time Clock Module
The DS1307 is a popular real-time clock (RTC) module that provides accurate timekeeping for embedded systems, including Arduino projects. This low-power chip maintains the current date and time, even when the main power supply is interrupted, thanks to its built-in backup battery. In this article, we will explore how to interface the DS1307 with an Arduino board and utilize its features in your projects.
Key Features of the DS1307
- Accurate timekeeping with seconds, minutes, hours, day, date, month, and year
- 56-byte non-volatile RAM for data storage
- I2C interface for easy communication with microcontrollers
- Low-power consumption and automatic power-fail detect and switch circuitry
- Operating voltage range: 4.5V to 5.5V
Hardware Requirements
To interface the DS1307 with an Arduino, you will need the following components:
- Arduino board (e.g., Arduino Uno)
- DS1307 RTC module
- Breadboard
- Jumper wires
- 4.7k ohm pull-up resistors (optional, depending on your module)
Wiring the DS1307 to the Arduino
The DS1307 communicates with the Arduino using the I2C protocol. Follow these steps to connect the DS1307 to your Arduino:
- Connect the VCC pin of the DS1307 to the 5V pin on the Arduino.
- Connect the GND pin of the DS1307 to any GND pin on the Arduino.
- Connect the SDA pin of the DS1307 to the SDA pin on the Arduino (A4 on Arduino Uno).
- Connect the SCL pin of the DS1307 to the SCL pin on the Arduino (A5 on Arduino Uno).
If your DS1307 module does not have built-in pull-up resistors, you will need to add 4.7k ohm pull-up resistors between the SDA and VCC pins, and between the SCL and VCC pins.
DS1307 Pin | Arduino Pin |
---|---|
VCC | 5V |
GND | GND |
SDA | A4 (SDA) |
SCL | A5 (SCL) |
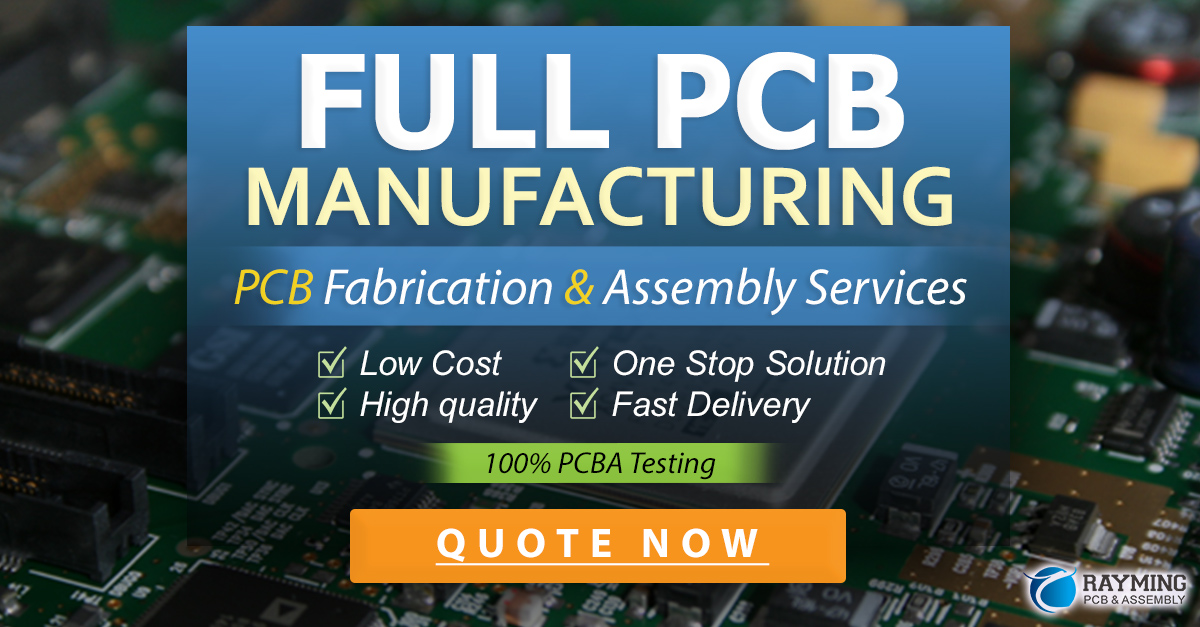
Installing the RTClib Library
To simplify the process of communicating with the DS1307, we will use the RTClib library by Adafruit. Follow these steps to install the library:
- Open the Arduino IDE.
- Navigate to Sketch > Include Library > Manage Libraries.
- In the Library Manager window, search for “RTClib”.
- Click on the “RTClib by Adafruit” entry and click the “Install” button.
Setting the Current Time on the DS1307
Before using the DS1307 to keep track of time, you need to set the current date and time. Use the following Arduino sketch to set the initial time:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
rtc.begin();
// Uncomment the line below to set the time (once) and upload the sketch
// rtc.adjust(DateTime(2023, 4, 15, 10, 30, 0)); // YYYY, MM, DD, HH, MM, SS
}
void loop() {
// Empty loop
}
Uncomment the rtc.adjust()
line, replace the date and time with the current values, and upload the sketch to your Arduino. After uploading, comment out the rtc.adjust()
line to prevent the time from being reset every time the Arduino restarts.
Reading the Current Time from the DS1307
To read the current date and time from the DS1307, use the following Arduino sketch:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
rtc.begin();
}
void loop() {
DateTime now = rtc.now();
Serial.print("Date: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" Time: ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.print(now.second(), DEC);
Serial.println();
delay(1000);
}
This sketch reads the current date and time from the DS1307 every second and displays the information in the Serial Monitor.
Using the DS1307’s Non-Volatile RAM
The DS1307 features 56 bytes of non-volatile RAM that can be used to store small amounts of data. This data will persist even when the power is disconnected. To read and write data to the DS1307’s RAM, use the following functions:
rtc.readnvram(address)
: Reads a byte from the specified address (0-55) in the DS1307’s RAM.rtc.writenvram(address, value)
: Writes a byte to the specified address (0-55) in the DS1307’s RAM.
Here’s an example sketch that demonstrates writing and reading data from the DS1307’s RAM:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
rtc.begin();
// Write a value to the DS1307's RAM
rtc.writenvram(0, 42);
}
void loop() {
// Read the value from the DS1307's RAM
byte value = rtc.readnvram(0);
Serial.print("Value stored in DS1307 RAM: ");
Serial.println(value);
delay(1000);
}
Troubleshooting Common Issues
Incorrect Time Displayed
If the DS1307 displays an incorrect time, ensure that you have set the initial time correctly using the rtc.adjust()
function. Double-check the wiring connections and make sure the backup battery is installed and functioning properly.
Communication Issues
If you encounter communication issues between the Arduino and the DS1307, verify the following:
- The wiring connections are correct and secure.
- The I2C address of the DS1307 is correct (0x68 by default).
- The necessary pull-up resistors are in place if your module does not have built-in pull-up resistors.
- The RTClib library is installed correctly.
Frequently Asked Questions (FAQ)
-
Can I use the DS1307 without a backup battery?
Yes, you can use the DS1307 without a backup battery. However, the time will be lost when the main power supply is disconnected, and you will need to reset the time each time the Arduino restarts. -
How accurate is the DS1307?
The DS1307 has a typical accuracy of ±2ppm at 25°C, which translates to about ±1 minute per year. The accuracy may vary depending on factors such as temperature and aging. -
Can I use multiple DS1307 modules with a single Arduino?
Yes, you can connect multiple DS1307 modules to a single Arduino using the I2C bus. Each module must have a unique I2C address, which can be set using the solder pads on the module. -
How long does the backup battery last?
The backup battery life depends on factors such as the battery type, capacity, and temperature. A typical CR2032 coin cell battery can last several years when used as a backup for the DS1307. -
Can I use the DS1307 with other microcontrollers besides Arduino?
Yes, the DS1307 can be used with any microcontroller that supports the I2C communication protocol. The specific wiring and software implementation may vary depending on the microcontroller and programming language used.
Conclusion
Interfacing the DS1307 real-time clock module with an Arduino is a straightforward process that enables accurate timekeeping in your projects. By following the wiring instructions, installing the RTClib library, and using the provided example sketches, you can easily incorporate the DS1307 into your Arduino projects. The module’s non-volatile RAM also offers the added benefit of storing small amounts of persistent data. With its low power consumption and reliable performance, the DS1307 is an excellent choice for a wide range of applications that require precise timekeeping.
Leave a Reply