Introduction to Heart Rate Monitoring
Monitoring heart rate is an important aspect of assessing an individual’s cardiovascular health and fitness level. Heart rate, measured in beats per minute (BPM), indicates how efficiently the heart is pumping blood throughout the body. A heart rate monitor circuit is an electronic device that detects and displays a person’s heart rate in real-time. This guide will provide a comprehensive overview of heart rate monitoring, including the principles behind it, the components required to build a heart rate monitor circuit, and step-by-step instructions for assembling the circuit.
Importance of Heart Rate Monitoring
Heart rate monitoring is crucial for various reasons:
-
Fitness Training: Athletes and fitness enthusiasts use heart rate monitors to optimize their training sessions. By maintaining a target heart rate range, they can ensure they are working out at the appropriate intensity level for their goals.
-
Medical Diagnosis: Healthcare professionals use heart rate monitoring to diagnose and monitor various cardiovascular conditions, such as arrhythmias, tachycardia (high heart rate), and bradycardia (low heart rate).
-
Stress Management: Heart rate can be an indicator of stress levels. Monitoring heart rate can help individuals identify stressful situations and take necessary steps to manage their stress.
Principles of Heart Rate Monitoring
Photoplethysmography (PPG)
The most common method used in heart rate monitor circuits is photoplethysmography (PPG). PPG is an optical technique that detects changes in blood volume in the microvascular bed of tissue. It relies on the fact that blood absorbs more light than the surrounding tissue.
A typical PPG sensor consists of an LED (light-emitting diode) and a photodetector. The LED emits light onto the skin, and the photodetector measures the amount of light that is reflected or transmitted through the tissue. As the heart pumps blood, the blood volume in the arteries and capillaries increases and decreases, causing variations in the amount of light absorbed. These variations are detected by the photodetector and can be used to calculate the heart rate.
Electrode-Based Monitoring
Another method for heart rate monitoring is using electrodes to detect the electrical activity of the heart. This method, known as electrocardiography (ECG), requires placing electrodes on the chest to measure the electrical impulses generated by the heart muscle during each heartbeat. While ECG provides more detailed information about the heart’s activity, it is less commonly used in wearable heart rate monitor circuits due to its complexity and the need for precise electrode placement.
Components of a Heart Rate Monitor Circuit
To build a heart rate monitor circuit, you will need the following components:
-
PPG Sensor: A reflective PPG sensor, such as the MAX30102 or the SEN-11574, which includes an integrated LED and photodetector.
-
Microcontroller: A microcontroller, such as the Arduino Uno or the ATmega328P, to process the PPG sensor data and calculate the heart rate.
-
Display: An LCD or OLED display to show the heart rate readings.
-
Power Source: A battery or power supply to power the circuit. A 3.7V lithium-ion battery is a common choice for portable heart rate monitor circuits.
-
Passive Components: Resistors, capacitors, and other passive components as required by the specific circuit design.
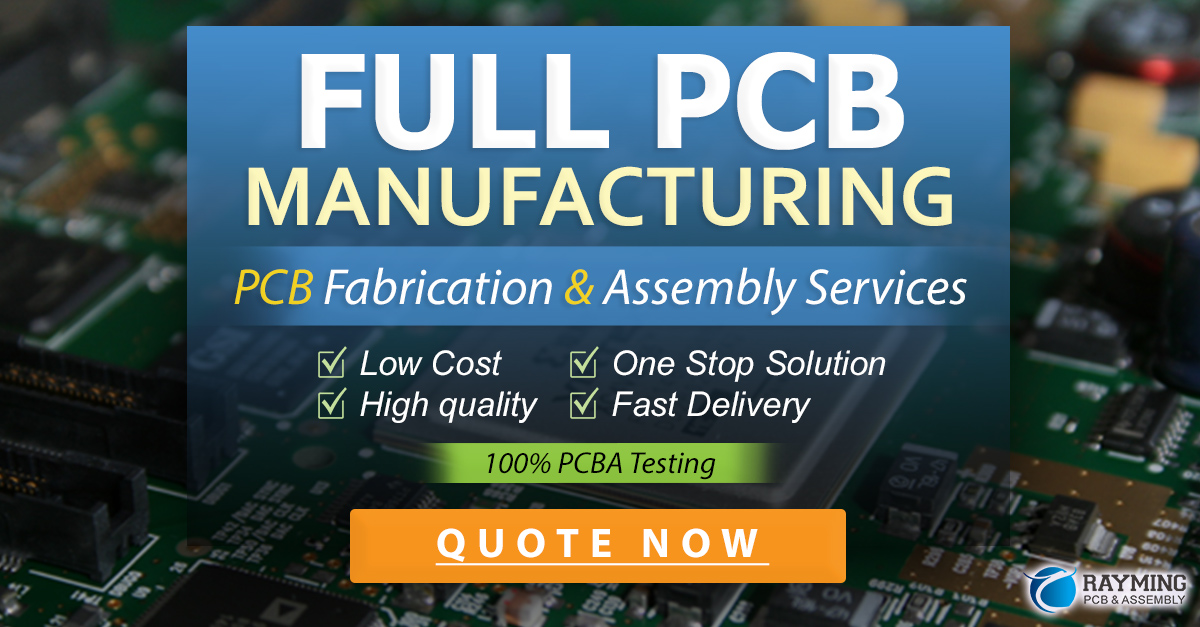
Building the Heart Rate Monitor Circuit
Step 1: Connect the PPG Sensor
Connect the PPG sensor to the microcontroller according to the sensor’s datasheet. Typically, the sensor will have the following connections:
- VCC: Connect to the microcontroller’s 3.3V or 5V power supply, depending on the sensor’s specifications.
- GND: Connect to the microcontroller’s ground.
- SDA: Connect to the microcontroller’s I2C data pin (e.g., A4 on Arduino Uno).
- SCL: Connect to the microcontroller’s I2C clock pin (e.g., A5 on Arduino Uno).
Step 2: Connect the Display
Connect the LCD or OLED display to the microcontroller according to the display’s datasheet. The connection will vary depending on the type of display used. For example, a 16×2 LCD display typically has the following connections:
- VSS: Connect to the microcontroller’s ground.
- VDD: Connect to the microcontroller’s 5V power supply.
- VO: Connect to the middle pin of a potentiometer for contrast adjustment.
- RS, RW, and E: Connect to the microcontroller’s digital pins for communication.
- D4-D7: Connect to the microcontroller’s digital pins for data transfer.
- LED+ and LED-: Connect to the microcontroller’s 5V and ground, respectively, with a current-limiting resistor in series.
Step 3: Power the Circuit
Connect the power source to the microcontroller and ensure that all components receive the appropriate voltage levels. If using a 3.7V lithium-ion battery, you may need to use a voltage regulator to step up the voltage for the microcontroller and display.
Step 4: Upload the Code
Write the code for the heart rate monitor circuit using the Arduino IDE or another suitable programming environment. The code should perform the following tasks:
- Initialize the PPG sensor and display.
- Continuously read the PPG sensor data.
- Process the PPG data to calculate the heart rate using peak detection or frequency analysis algorithms.
- Display the calculated heart rate on the LCD or OLED display.
- Implement any additional features, such as heart rate zone tracking or data logging.
Here’s a sample code snippet for reading heart rate data from the MAX30102 sensor:
#include <Wire.h>
#include "MAX30105.h"
MAX30105 particleSensor;
const byte RATE_SIZE = 4; // Increase this for more averaging. 4 is good.
byte rates[RATE_SIZE]; // Array of heart rates
byte rateSpot = 0;
long lastBeat = 0; // Time at which the last beat occurred
float beatsPerMinute;
int beatAvg;
void setup() {
Serial.begin(115200);
particleSensor.begin(Wire, I2C_SPEED_FAST); // Use default I2C port, 400kHz speed
particleSensor.setup(); // Configure sensor with default settings
particleSensor.setPulseAmplitudeRed(0x0A); // Turn Red LED to low to indicate sensor is running
}
void loop() {
long irValue = particleSensor.getIR();
if (checkForBeat(irValue) == true) {
// We sensed a beat!
long delta = millis() - lastBeat;
lastBeat = millis();
beatsPerMinute = 60 / (delta / 1000.0);
if (beatsPerMinute < 255 && beatsPerMinute > 20) {
rates[rateSpot++] = (byte)beatsPerMinute; // Store this reading in the array
rateSpot %= RATE_SIZE; // Wrap variable
// Take average of readings
beatAvg = 0;
for (byte x = 0; x < RATE_SIZE; x++)
beatAvg += rates[x];
beatAvg /= RATE_SIZE;
}
}
Serial.print("IR=");
Serial.print(irValue);
Serial.print(", BPM=");
Serial.print(beatsPerMinute);
Serial.print(", Avg BPM=");
Serial.print(beatAvg);
if (irValue < 50000)
Serial.print(" No finger?");
Serial.println();
}
This code uses the MAX30102 library to read the infrared (IR) value from the sensor, detect heart beats, and calculate the beats per minute (BPM). It also takes an average of the last 4 readings to smooth out the heart rate data.
Step 5: Test and Calibrate
Once the circuit is assembled and the code is uploaded, test the heart rate monitor by placing your finger on the PPG sensor. The display should show your current heart rate. If the readings appear inaccurate or erratic, you may need to calibrate the sensor or adjust the code parameters, such as the threshold for detecting heart beats.
Heart Rate Data Analysis
After successfully building and testing the heart rate monitor circuit, you can further analyze the collected heart rate data to gain insights into your cardiovascular health and fitness. Some common heart rate data analysis techniques include:
-
Resting Heart Rate (RHR): RHR is the heart rate measured while at rest, usually immediately after waking up in the morning. Tracking RHR over time can indicate changes in cardiovascular fitness, with lower RHR generally signifying better fitness.
-
Maximum Heart Rate (MHR): MHR is the highest heart rate an individual can safely achieve during intense exercise. It can be estimated using the formula: MHR = 220 – age. However, this formula is a general guideline, and actual MHR may vary among individuals.
-
Heart Rate Zones: Heart rate zones are ranges of heart rates that correspond to different levels of exercise intensity. These zones can be used to target specific training goals, such as fat burning or improving cardiovascular endurance. A common heart rate zone model is as follows:
Zone | Intensity | Heart Rate Range (% of MHR) |
---|---|---|
1 | Very Light | 50-60% |
2 | Light | 60-70% |
3 | Moderate | 70-80% |
4 | Hard | 80-90% |
5 | Maximum | 90-100% |
- Heart Rate Variability (HRV): HRV is the variation in time between consecutive heart beats. It can be used as an indicator of overall health, stress levels, and recovery status. Higher HRV generally indicates better cardiovascular fitness and resilience to stress.
By incorporating these analysis techniques into your heart rate monitor circuit project, you can gain a deeper understanding of your heart health and make data-driven decisions to optimize your fitness training and lifestyle.
FAQ
1. Can I use a different microcontroller for this project?
Yes, you can use any microcontroller that supports I2C communication and has enough memory and processing power to handle the PPG sensor data and calculations. Some popular alternatives to the Arduino Uno include the ESP32, Raspberry Pi Pico, and STM32 series microcontrollers.
2. How accurate are heart rate monitor circuits?
The accuracy of a heart rate monitor circuit depends on various factors, such as the quality of the PPG sensor, the signal processing algorithms used, and the placement of the sensor on the body. Generally, well-designed heart rate monitor circuits can achieve an accuracy of ±2-5 BPM compared to medical-grade ECG devices. However, factors like motion artifacts, ambient light interference, and individual skin characteristics can affect the accuracy.
3. Can I use this heart rate monitor circuit for medical purposes?
While heart rate monitor circuits can provide useful information about heart rate and cardiovascular health, they should not be used as a substitute for professional medical advice or diagnosis. If you have concerns about your heart health, consult a qualified healthcare provider who can perform a thorough evaluation using medical-grade equipment.
4. How can I improve the battery life of my heart rate monitor circuit?
To improve the battery life of your heart rate monitor circuit, consider the following tips:
- Use a low-power microcontroller and optimize your code for power efficiency.
- Implement sleep modes or power-down features when the device is not in use.
- Choose a power-efficient PPG sensor and optimize its sampling rate and LED brightness.
- Use a high-capacity battery, such as a lithium-ion or lithium-polymer cell.
- Employ power management techniques, such as voltage regulation and power gating.
5. Can I add additional features to the heart rate monitor circuit?
Yes, you can extend the functionality of your heart rate monitor circuit by adding features such as:
- Data logging and storage using an SD card or EEPROM.
- Wireless connectivity (e.g., Bluetooth or Wi-Fi) for data transmission to a smartphone app or web server.
- Integration with other sensors, such as an accelerometer or SpO2 sensor, for comprehensive health monitoring.
- Audio or visual alerts for high or low heart rates.
- GPS tracking for outdoor fitness activities.
Remember to consider the power consumption and complexity of additional features and ensure that your microcontroller and power source can support them.
Conclusion
Building a heart rate monitor circuit is an engaging and educational project that combines hardware and software skills. By understanding the principles of photoplethysmography, selecting the appropriate components, and writing efficient code, you can create a device that accurately measures and displays heart rate in real-time. This project not only provides insight into your own cardiovascular health but also serves as a foundation for exploring more advanced health monitoring applications. As you continue to refine your heart rate monitor circuit and analyze the data it collects, you will gain a deeper appreciation for the power of electronics and programming in the field of personal health and fitness.
Leave a Reply