Types of Motion Sensors
Before diving into the DIY Motion Detector circuits, let’s take a look at the different types of motion sensors available:
-
Passive Infrared (PIR) Sensors: PIR Sensors detect changes in infrared radiation emitted by moving objects or people. They are the most commonly used motion sensors in security systems and automation projects.
-
Microwave Sensors: These sensors emit high-frequency microwave signals and detect motion based on the Doppler shift in the reflected signals caused by moving objects.
-
Ultrasonic Sensors: Ultrasonic sensors work by emitting high-frequency sound waves and measuring the time it takes for the waves to bounce back from objects. They can detect motion based on changes in the reflected sound waves.
-
Dual Technology Sensors: These sensors combine two or more types of sensing technologies, such as PIR and microwave, to reduce false alarms and improve detection accuracy.
DIY Motion Detector Circuits
1. PIR Sensor Circuit
A PIR sensor circuit is the simplest and most popular way to build a motion detector. Here’s how to create one:
Components Required:
– PIR sensor module (e.g., HC-SR501)
– Arduino board (e.g., Arduino Uno)
– Jumper wires
– Breadboard (optional)
– LED (optional, for visual indication)
Circuit Diagram:
PIR Sensor | Arduino |
---|---|
VCC | 5V |
GND | GND |
OUT | Digital Pin (e.g., Pin 2) |
Code:
const int pirPin = 2;
const int ledPin = 13;
void setup() {
pinMode(pirPin, INPUT);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
int pirValue = digitalRead(pirPin);
if (pirValue == HIGH) {
digitalWrite(ledPin, HIGH);
Serial.println("Motion detected!");
} else {
digitalWrite(ledPin, LOW);
Serial.println("No motion.");
}
delay(500);
}
2. Microwave Sensor Circuit
Microwave sensors are more sensitive than PIR sensors and can detect motion through walls and other obstacles. Here’s how to build a microwave sensor circuit:
Components Required:
– Microwave sensor module (e.g., RCWL-0516)
– Arduino board (e.g., Arduino Uno)
– Jumper wires
– Breadboard (optional)
– LED (optional, for visual indication)
Circuit Diagram:
Microwave Sensor | Arduino |
---|---|
VCC | 5V |
GND | GND |
OUT | Digital Pin (e.g., Pin 2) |
Code:
const int microwavePin = 2;
const int ledPin = 13;
void setup() {
pinMode(microwavePin, INPUT);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
int microwaveValue = digitalRead(microwavePin);
if (microwaveValue == HIGH) {
digitalWrite(ledPin, HIGH);
Serial.println("Motion detected!");
} else {
digitalWrite(ledPin, LOW);
Serial.println("No motion.");
}
delay(500);
}
3. Ultrasonic Sensor Circuit
Ultrasonic sensors are useful for detecting motion and measuring distances. Here’s how to create an ultrasonic sensor circuit for motion detection:
Components Required:
– Ultrasonic sensor module (e.g., HC-SR04)
– Arduino board (e.g., Arduino Uno)
– Jumper wires
– Breadboard (optional)
– LED (optional, for visual indication)
Circuit Diagram:
Ultrasonic Sensor | Arduino |
---|---|
VCC | 5V |
GND | GND |
Trig | Digital Pin (e.g., Pin 9) |
Echo | Digital Pin (e.g., Pin 10) |
Code:
const int trigPin = 9;
const int echoPin = 10;
const int ledPin = 13;
const int threshold = 20; // Distance threshold in centimeters
void setup() {
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
long duration = pulseIn(echoPin, HIGH);
int distance = duration * 0.034 / 2;
if (distance < threshold) {
digitalWrite(ledPin, HIGH);
Serial.println("Motion detected!");
} else {
digitalWrite(ledPin, LOW);
Serial.println("No motion.");
}
delay(500);
}
4. Dual Technology Motion Detector Circuit
Combining PIR and microwave sensors can help reduce false alarms and improve detection accuracy. Here’s how to build a dual technology motion detector circuit:
Components Required:
– PIR sensor module (e.g., HC-SR501)
– Microwave sensor module (e.g., RCWL-0516)
– Arduino board (e.g., Arduino Uno)
– Jumper wires
– Breadboard (optional)
– LED (optional, for visual indication)
Circuit Diagram:
PIR Sensor | Arduino |
---|---|
VCC | 5V |
GND | GND |
OUT | Digital Pin (e.g., Pin 2) |
Microwave Sensor | Arduino |
---|---|
VCC | 5V |
GND | GND |
OUT | Digital Pin (e.g., Pin 3) |
Code:
const int pirPin = 2;
const int microwavePin = 3;
const int ledPin = 13;
void setup() {
pinMode(pirPin, INPUT);
pinMode(microwavePin, INPUT);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
int pirValue = digitalRead(pirPin);
int microwaveValue = digitalRead(microwavePin);
if (pirValue == HIGH && microwaveValue == HIGH) {
digitalWrite(ledPin, HIGH);
Serial.println("Motion detected by both sensors!");
} else if (pirValue == HIGH || microwaveValue == HIGH) {
digitalWrite(ledPin, HIGH);
Serial.println("Motion detected by one sensor!");
} else {
digitalWrite(ledPin, LOW);
Serial.println("No motion.");
}
delay(500);
}
5. Wireless Motion Detector Circuit
To create a wireless motion detector, you can use a PIR sensor and a wireless communication module, such as an ESP8266 or nRF24L01+. Here’s an example using an ESP8266:
Components Required:
– PIR sensor module (e.g., HC-SR501)
– ESP8266 module (e.g., NodeMCU)
– Jumper wires
– Breadboard (optional)
– LED (optional, for visual indication)
Circuit Diagram:
PIR Sensor | ESP8266 |
---|---|
VCC | 3.3V |
GND | GND |
OUT | Digital Pin (e.g., D1) |
Code:
#include <ESP8266WiFi.h>
const char* ssid = "your_wifi_ssid";
const char* password = "your_wifi_password";
const char* serverHost = "your_server_ip";
const int serverPort = 80;
const int pirPin = 5; // D1
void setup() {
pinMode(pirPin, INPUT);
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
void loop() {
int pirValue = digitalRead(pirPin);
if (pirValue == HIGH) {
Serial.println("Motion detected!");
sendDataToServer("Motion detected");
} else {
Serial.println("No motion.");
}
delay(500);
}
void sendDataToServer(String data) {
WiFiClient client;
if (client.connect(serverHost, serverPort)) {
client.println("POST /motion HTTP/1.1");
client.println("Host: " + String(serverHost));
client.println("Content-Type: text/plain");
client.println("Content-Length: " + String(data.length()));
client.println();
client.println(data);
}
client.stop();
}
Frequently Asked Questions (FAQ)
-
What is the detection range of PIR sensors?
PIR sensors typically have a detection range of 5-12 meters (16-40 feet) and a detection angle of 90-110 degrees. -
Can motion detectors be used outdoors?
Yes, there are outdoor-specific motion detectors available that are designed to withstand weather conditions and reduce false alarms caused by environmental factors like wind, rain, or small animals. -
How can I reduce false alarms in my motion detector circuit?
You can reduce false alarms by adjusting the sensitivity settings on your sensor, using dual technology sensors that combine PIR and microwave or ultrasonic sensors, or implementing software algorithms to filter out false triggers. -
Can I connect multiple motion sensors to a single Arduino board?
Yes, you can connect multiple motion sensors to a single Arduino board by using different digital pins for each sensor and modifying the code to handle multiple inputs. -
How can I power my motion detector circuit?
You can power your motion detector circuit using batteries, a wall adapter, or by connecting it to a power bank or solar panel, depending on your specific requirements and the components used in your circuit.
In conclusion, building a motion detector circuit is a fun and practical DIY project that can be achieved using various types of sensors and Arduino boards. By following the circuit diagrams and code examples provided in this article, you can create your own motion detector for security, automation, or energy-saving applications. Remember to choose the appropriate sensor type based on your specific needs and to adjust the sensitivity settings to reduce false alarms.
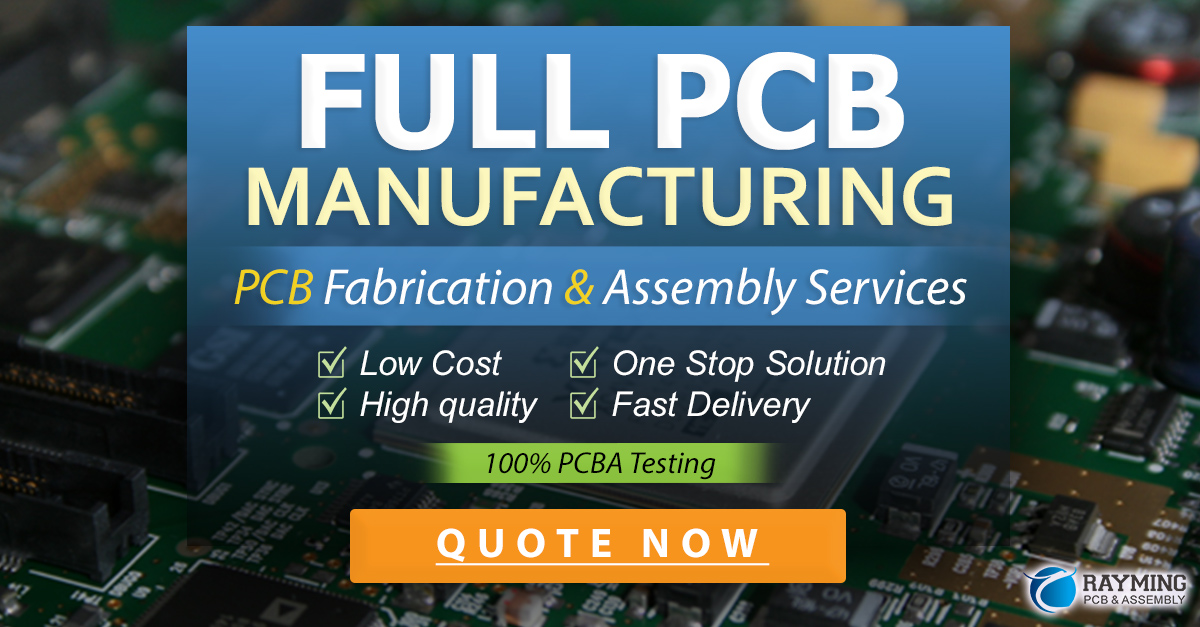
Leave a Reply