Introduction to Void Pointers
In the vast world of programming, pointers play a crucial role in efficiently manipulating data and memory. Among the various types of pointers available in the C programming language, void pointers stand out as a powerful and versatile tool. Void pointers, denoted as void*
, offer a unique ability to store the address of any data type, making them indispensable in a wide range of coding scenarios.
What are Void Pointers?
A void pointer is a special type of pointer that can hold the address of any data type, regardless of its size or representation. Unlike other pointers that are specific to a particular data type (e.g., int*
for integers, char*
for characters), void pointers provide a generic way to store and manipulate memory addresses.
The declaration of a void pointer is as follows:
void* ptr;
Here, ptr
is a void pointer that can store the address of any data type.
Advantages of Void Pointers
Void pointers offer several advantages that make them valuable in various programming situations:
-
Flexibility: Void pointers can store the address of any data type, allowing you to write generic code that can handle different types of data without the need for type-specific pointers.
-
Code Reusability: By using void pointers, you can create functions or data structures that can work with different data types, promoting code reusability and reducing duplication.
-
Dynamic Memory Allocation: Void pointers are commonly used in dynamic memory allocation functions, such as
malloc()
andcalloc()
, which return a void pointer to the allocated memory block. -
Polymorphism: Void pointers enable a form of polymorphism in C, allowing you to write functions that can accept and operate on different data types through a single interface.
Using Void Pointers
Assigning Addresses to Void Pointers
To assign the address of a variable to a void pointer, you can simply use the address-of operator (&
):
int num = 10;
void* ptr = #
In this example, ptr
stores the address of the integer variable num
.
Dereferencing Void Pointers
To access the value stored at the address pointed to by a void pointer, you need to explicitly cast the void pointer to the appropriate data type before dereferencing it:
int num = 10;
void* ptr = #
int value = *(int*)ptr;
Here, ptr
is cast to an int*
pointer before dereferencing it to retrieve the value of num
.
Void Pointers in Function Parameters
Void pointers are commonly used as function parameters to create generic functions that can accept different data types. For example:
void printValue(void* ptr, char type) {
switch (type) {
case 'i':
printf("Integer: %d\n", *(int*)ptr);
break;
case 'f':
printf("Float: %.2f\n", *(float*)ptr);
break;
case 'c':
printf("Character: %c\n", *(char*)ptr);
break;
}
}
In this function, ptr
is a void pointer that can accept the address of different data types, and type
is a character that specifies the actual data type being passed. Based on the type
, the void pointer is cast to the appropriate pointer type and dereferenced to access the value.
Void Pointers and Dynamic Memory Allocation
Void pointers are frequently used in dynamic memory allocation functions, such as malloc()
and calloc()
, which return a void pointer to the allocated memory block. Here’s an example:
int* arr = (int*)malloc(10 * sizeof(int));
In this case, malloc()
allocates memory for an array of 10 integers and returns a void pointer to the allocated memory. The void pointer is then explicitly cast to an int*
pointer to store the address of the integer array.
Limitations and Precautions
While void pointers offer flexibility and power, there are some limitations and precautions to keep in mind when using them:
-
Type Safety: Void pointers disable type checking, making it the programmer’s responsibility to ensure that the correct data type is used when dereferencing the pointer. Mismatching the data type can lead to undefined behavior and potential memory corruption.
-
Pointer Arithmetic: Performing pointer arithmetic on void pointers is not allowed in C. You cannot increment or decrement a void pointer directly. Instead, you need to cast the void pointer to a specific pointer type before performing arithmetic operations.
-
Readability and Maintainability: Excessive use of void pointers can make code harder to read and maintain. It is important to use meaningful names and provide clear documentation to indicate the intended data type and usage of void pointers.
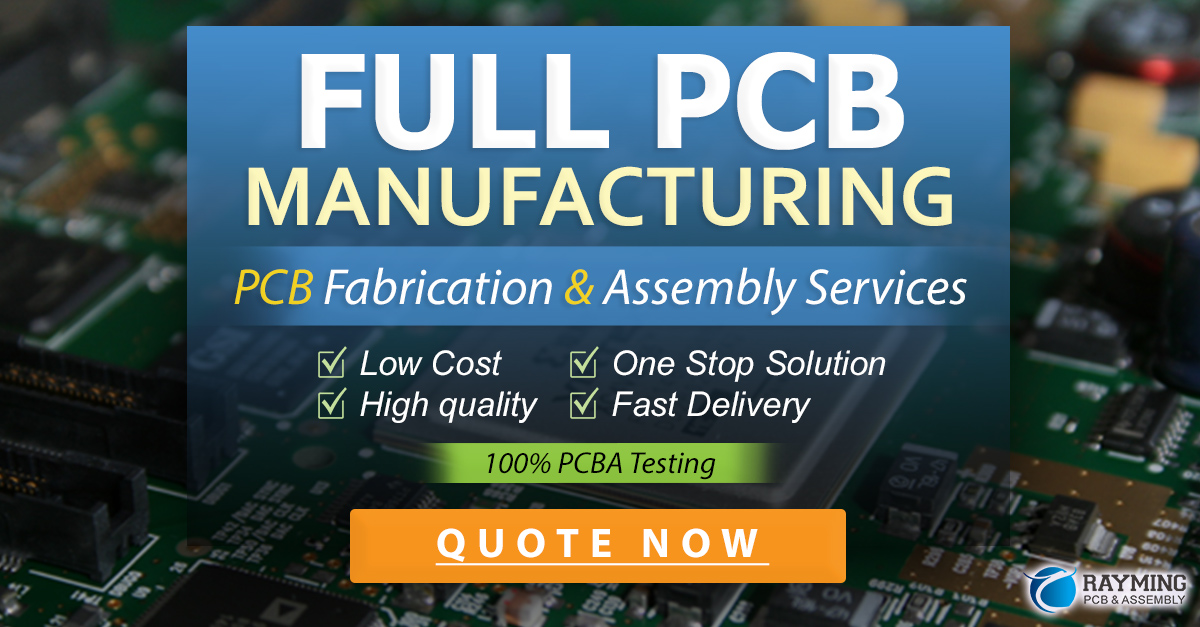
Real-World Applications
Void pointers find applications in various real-world scenarios. Let’s explore a few examples:
1. Generic Data Structures
Void pointers are commonly used to implement generic data structures, such as linked lists, trees, or hash tables, that can store elements of different data types. By using void pointers, you can create a single data structure that can handle different types of data without the need for separate implementations for each data type.
Here’s an example of a simple generic linked list node using void pointers:
struct Node {
void* data;
struct Node* next;
};
In this structure, the data
field is a void pointer that can store the address of any data type, allowing the linked list to store elements of different types.
2. Callback Functions
Void pointers are often used in callback functions to provide a generic way to pass data between functions. Callback functions are functions that are passed as arguments to other functions and are invoked later based on certain conditions or events.
Consider an example of a sorting function that accepts a comparison callback:
void sort(void* arr, int size, int elemSize, int (*compare)(const void*, const void*)) {
// Sorting logic using the compare callback
}
In this function, arr
is a void pointer to the array to be sorted, size
is the number of elements, elemSize
is the size of each element, and compare
is a callback function that compares two elements. The callback function takes two void pointers as arguments, allowing it to compare elements of any data type.
3. Plugin Systems
Void pointers are useful in designing plugin systems or extensible architectures. By defining a common interface using void pointers, you can create a mechanism for dynamically loading and invoking plugins or extensions without having to know their specific data types at compile time.
Here’s a simplified example of a plugin system using void pointers:
typedef struct {
void* (*initialize)(void);
void (*process)(void* data);
void (*cleanup)(void* data);
} Plugin;
void loadPlugin(const char* pluginPath) {
void* handle = dlopen(pluginPath, RTLD_LAZY);
if (handle) {
Plugin* plugin = (Plugin*)dlsym(handle, "plugin");
if (plugin) {
void* data = plugin->initialize();
plugin->process(data);
plugin->cleanup(data);
}
dlclose(handle);
}
}
In this example, the Plugin
structure defines a common interface for plugins, using void pointers for the initialize
, process
, and cleanup
functions. The loadPlugin
function dynamically loads a plugin library, retrieves the plugin structure using dlsym
, and invokes the plugin’s functions through the void pointers.
Best Practices
When working with void pointers, it’s important to follow some best practices to ensure code clarity, maintainability, and avoid potential pitfalls:
-
Use meaningful names: Choose descriptive names for void pointers that convey their purpose and the type of data they are intended to store.
-
Document the intended data type: Provide clear documentation or comments indicating the expected data type of the void pointer, especially when passing it to functions or storing it in data structures.
-
Ensure proper casting: Always cast void pointers to the appropriate data type before dereferencing or performing any operations on them. Mismatched casting can lead to undefined behavior.
-
Avoid excessive use: While void pointers provide flexibility, overusing them can make the code harder to understand and maintain. Use them judiciously and consider alternative approaches when possible.
-
Handle memory management carefully: When using void pointers with dynamically allocated memory, ensure proper memory management by allocating and deallocating memory consistently and avoiding memory leaks.
Frequently Asked Questions (FAQ)
-
Q: Can void pointers be used to store the address of any data type?
A: Yes, void pointers can store the address of any data type, regardless of its size or representation. -
Q: How do you dereference a void pointer?
A: To dereference a void pointer, you need to explicitly cast it to the appropriate pointer type before using the dereference operator (*
). For example:int value = *(int*)ptr;
. -
Q: Can you perform pointer arithmetic on void pointers?
A: No, pointer arithmetic is not allowed on void pointers directly. You need to cast the void pointer to a specific pointer type before performing arithmetic operations. -
Q: What happens if you dereference a void pointer without casting it to the correct type?
A: Dereferencing a void pointer without casting it to the correct type can lead to undefined behavior and potential memory corruption. It is crucial to ensure proper casting before dereferencing. -
Q: Are there any limitations or precautions when using void pointers?
A: Yes, void pointers disable type checking, making it the programmer’s responsibility to ensure the correct data type is used when dereferencing. Mismatching the data type can lead to undefined behavior. Additionally, excessive use of void pointers can make code harder to read and maintain.
Conclusion
Void pointers in C provide a powerful and flexible mechanism for storing and manipulating memory addresses of any data type. They offer benefits such as code reusability, polymorphism, and the ability to create generic functions and data structures. However, it is important to use void pointers judiciously, ensure proper casting, and follow best practices to maintain code clarity and avoid potential issues.
By understanding the concepts and applications of void pointers, you can enhance your coding experience and tackle a wide range of programming challenges effectively. Embrace the power of void pointers in your C projects, and unlock new possibilities in your software development journey.
Leave a Reply