Project 1: Basic IR Remote Control Tester
Materials Needed
Component | Quantity |
---|---|
IR Receiver Module (e.g., TSOP4838) | 1 |
Arduino Uno or Compatible Board | 1 |
Breadboard | 1 |
Jumper Wires | As needed |
USB Cable | 1 |
Step-by-Step Instructions
- Connect the IR receiver module to the Arduino board:
- Connect the VCC pin of the IR receiver to the 5V pin on the Arduino.
- Connect the GND pin of the IR receiver to the GND pin on the Arduino.
-
Connect the OUT pin of the IR receiver to digital pin 11 on the Arduino.
-
Open the Arduino IDE and create a new sketch.
-
Copy and paste the following code into the sketch:
#include <IRremote.h>
int RECV_PIN = 11;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup() {
Serial.begin(9600);
irrecv.enableIRIn();
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
irrecv.resume();
}
}
-
Upload the sketch to the Arduino board.
-
Open the Serial Monitor in the Arduino IDE.
-
Point your IR remote control at the IR receiver and press a button.
-
Observe the hexadecimal code of the button press in the Serial Monitor.
How It Works
The basic IR remote control tester uses an IR receiver module (such as the TSOP4838) to detect the infrared signals emitted by the remote control. The IR receiver is connected to an Arduino board, which processes the received signal and displays the corresponding hexadecimal code on the Serial Monitor.
The code utilizes the IRremote library to simplify the process of receiving and decoding IR signals. When a button on the remote control is pressed, the IR receiver captures the signal, and the Arduino board decodes it using the IRremote library. The decoded hexadecimal code is then displayed on the Serial Monitor, allowing you to identify the specific button press.
Project 2: Advanced IR Remote Control Tester with LCD Display
Materials Needed
Component | Quantity |
---|---|
IR Receiver Module (e.g., TSOP4838) | 1 |
Arduino Uno or Compatible Board | 1 |
16×2 LCD Display | 1 |
10K Potentiometer | 1 |
Breadboard | 1 |
Jumper Wires | As needed |
USB Cable | 1 |
Step-by-Step Instructions
-
Connect the IR receiver module to the Arduino board as described in Project 1.
-
Connect the LCD display to the Arduino board:
- Connect the VSS pin of the LCD to GND.
- Connect the VDD pin of the LCD to 5V.
- Connect the V0 pin of the LCD to the middle pin of the potentiometer.
- Connect the RS pin of the LCD to digital pin 12 on the Arduino.
- Connect the RW pin of the LCD to GND.
- Connect the E pin of the LCD to digital pin 11 on the Arduino.
- Connect data pins D4, D5, D6, and D7 of the LCD to digital pins 5, 4, 3, and 2 on the Arduino, respectively.
- Connect the A pin of the LCD to 5V.
-
Connect the K pin of the LCD to GND.
-
Connect the outer pins of the potentiometer to 5V and GND.
-
Open the Arduino IDE and create a new sketch.
-
Copy and paste the following code into the sketch:
#include <IRremote.h>
#include <LiquidCrystal.h>
int RECV_PIN = 10;
IRrecv irrecv(RECV_PIN);
decode_results results;
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
irrecv.enableIRIn();
lcd.begin(16, 2);
lcd.print("IR Remote Tester");
}
void loop() {
if (irrecv.decode(&results)) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Code: 0x");
lcd.print(results.value, HEX);
lcd.setCursor(0, 1);
lcd.print("Bits: ");
lcd.print(results.bits);
irrecv.resume();
delay(500);
}
}
-
Upload the sketch to the Arduino board.
-
Point your IR remote control at the IR receiver and press a button.
-
Observe the hexadecimal code and the number of bits of the button press on the LCD display.
How It Works
The advanced IR remote control tester builds upon the basic tester by adding an LCD display to provide a more user-friendly interface. The LCD display shows the hexadecimal code and the number of bits of the received IR signal.
The IR receiver module captures the infrared signal from the remote control, and the Arduino board decodes it using the IRremote library. The decoded information, including the hexadecimal code and the number of bits, is then displayed on the LCD screen.
The potentiometer connected to the V0 pin of the LCD allows you to adjust the contrast of the display for better visibility.
Project 3: IR Remote Control Tester with Database Lookup
Materials Needed
Component | Quantity |
---|---|
IR Receiver Module (e.g., TSOP4838) | 1 |
Arduino Uno or Compatible Board | 1 |
16×2 LCD Display | 1 |
10K Potentiometer | 1 |
Breadboard | 1 |
Jumper Wires | As needed |
USB Cable | 1 |
Step-by-Step Instructions
-
Set up the hardware connections as described in Project 2.
-
Open the Arduino IDE and create a new sketch.
-
Copy and paste the following code into the sketch:
#include <IRremote.h>
#include <LiquidCrystal.h>
int RECV_PIN = 10;
IRrecv irrecv(RECV_PIN);
decode_results results;
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
// IR remote control codes and corresponding button names
const unsigned long CODES[] = {0x1FE48B7, 0x1FE50AF, 0x1FE708F, 0x1FE807F};
const char* BUTTONS[] = {"Power", "Volume+", "Volume-", "Mute"};
const int NUM_CODES = sizeof(CODES) / sizeof(CODES[0]);
void setup() {
irrecv.enableIRIn();
lcd.begin(16, 2);
lcd.print("IR Remote Tester");
}
void loop() {
if (irrecv.decode(&results)) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Code: 0x");
lcd.print(results.value, HEX);
lcd.setCursor(0, 1);
bool found = false;
for (int i = 0; i < NUM_CODES; i++) {
if (results.value == CODES[i]) {
lcd.print(BUTTONS[i]);
found = true;
break;
}
}
if (!found) {
lcd.print("Unknown");
}
irrecv.resume();
delay(500);
}
}
-
Modify the
CODES
andBUTTONS
arrays in the code to match the IR codes and corresponding button names of your specific remote control. -
Upload the sketch to the Arduino board.
-
Point your IR remote control at the IR receiver and press a button.
-
Observe the hexadecimal code and the corresponding button name (if available) on the LCD display.
How It Works
The IR remote control tester with database lookup extends the functionality of the previous projects by adding a database of known IR codes and their corresponding button names. This allows the tester to display not only the hexadecimal code of the received IR signal but also the actual button name associated with that code.
The database is implemented as two arrays in the code: CODES
and BUTTONS
. The CODES
array contains the known IR codes, and the BUTTONS
array contains the corresponding button names. When an IR signal is received, the tester compares the received code with the codes in the database. If a match is found, the corresponding button name is displayed on the LCD. If no match is found, “Unknown” is displayed.
You can customize the CODES
and BUTTONS
arrays to include the IR codes and button names specific to your remote control. This allows you to quickly identify the buttons being pressed without having to refer to a separate code table.
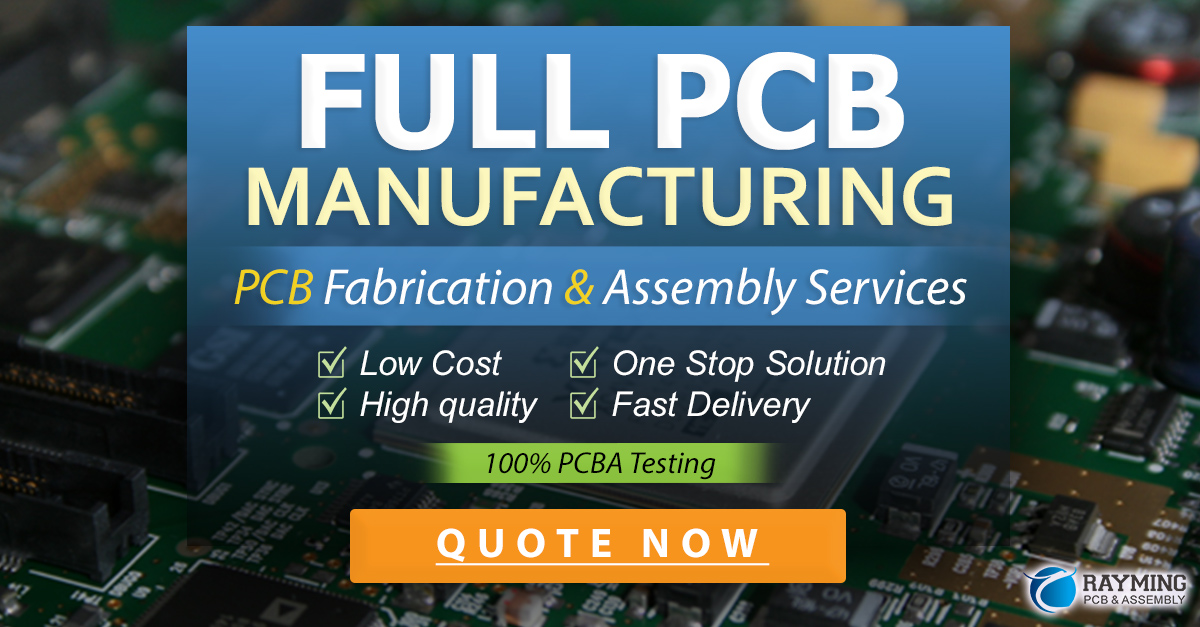
Frequently Asked Questions (FAQ)
-
Q: What is an IR remote control tester?
A: An IR remote control tester is a device that allows you to test and troubleshoot infrared remote controls. It receives the IR signals emitted by the remote control and displays information about the received signal, such as the hexadecimal code and the corresponding button press. -
Q: What components do I need to build an IR remote control tester?
A: To build a basic IR remote control tester, you’ll need an IR receiver module (such as TSOP4838), an Arduino board, a breadboard, jumper wires, and a USB cable. For more advanced projects, you may also need an LCD display and a potentiometer. -
Q: Can I use any Arduino board for these projects?
A: Yes, you can use any Arduino board or compatible board that has sufficient digital pins and supports the Arduino IDE. The most common choice is the Arduino Uno, but you can also use boards like the Arduino Nano or Arduino Mega. -
Q: How do I connect the IR receiver module to the Arduino board?
A: To connect the IR receiver module to the Arduino board, you need to connect the VCC pin of the receiver to the 5V pin on the Arduino, the GND pin of the receiver to the GND pin on the Arduino, and the OUT pin of the receiver to a digital pin on the Arduino (e.g., pin 11). -
Q: Can I customize the IR remote control tester to work with my specific remote control?
A: Yes, you can customize the IR remote control tester to work with your specific remote control. In Project 3, you can modify theCODES
andBUTTONS
arrays in the code to include the IR codes and corresponding button names of your remote control. This allows the tester to display the actual button names when you press the buttons on your remote.
Building an IR remote control tester is a great way to learn about infrared communication and troubleshoot remote control issues. Whether you choose to build a basic tester or a more advanced one with an LCD display and database lookup, these DIY Projects will enhance your electronics skills and provide you with a useful tool for testing and debugging remote controls.
Leave a Reply