What is the PIC16F877A Microcontroller?
The PIC16F877A is an 8-bit microcontroller developed by Microchip Technology. It belongs to the PIC16 family of microcontrollers and is based on the enhanced Harvard RISC architecture. This microcontroller is known for its high performance, low power consumption, and extensive peripheral set.
Key Features of the PIC16F877A
Feature | Description |
---|---|
CPU | 8-bit RISC CPU |
Operating Frequency | Up to 20 MHz |
Program Memory | 14 KB Flash |
Data Memory | 368 bytes SRAM |
EEPROM | 256 bytes |
I/O Pins | 33 |
Timers | 3 (2 x 8-bit, 1 x 16-bit) |
ADC | 8 channels, 10-bit resolution |
USART | Yes |
SPI | Yes |
I2C | Yes |
Parallel Slave Port | Yes |
Analog Comparators | 2 |
Instruction Set | 35 instructions |
The PIC16F877A offers a comprehensive set of peripherals, making it suitable for a wide range of applications. Its high operating frequency and efficient architecture enable fast execution of instructions, while the ample program and data memory provide sufficient space for complex applications.
Advantages of Using the PIC16F877A
-
Versatility: The PIC16F877A can be used in a wide range of applications, including embedded systems, automation, robotics, and data acquisition.
-
Easy to Program: With its simple instruction set and availability of development tools, the PIC16F877A is easy to program and debug.
-
Low Power Consumption: The microcontroller operates at low voltages and consumes minimal power, making it ideal for battery-powered applications.
-
Extensive Peripheral Set: The PIC16F877A offers a rich set of peripherals, including ADC, USART, SPI, I2C, and timers, enabling seamless interfacing with various sensors and devices.
-
Large User Community: Due to its popularity, the PIC16F877A has a large user community, providing access to abundant resources, tutorials, and support.
Selecting the Right PIC16F877A for Your Project
When choosing a PIC16F877A for your project, consider the following factors:
1. Package Type
The PIC16F877A is available in various package types, including DIP (Dual Inline Package), PLCC (Plastic Leaded Chip Carrier), and TQFP (Thin Quad Flat Pack). Consider the size constraints of your project and choose the appropriate package accordingly.
2. Temperature Range
Microcontrollers are available in different temperature ranges, such as commercial (0°C to 70°C) and industrial (-40°C to 85°C). Select the temperature range based on the operating environment of your project.
3. Oscillator Type
The PIC16F877A supports various oscillator modes, including HS (High-Speed Crystal), XT (Crystal), and RC (Resistor-Capacitor). Choose the oscillator type based on the required clock frequency and accuracy of your application.
4. Memory Requirements
Evaluate the memory requirements of your project, including program memory and data memory. Ensure that the PIC16F877A has sufficient memory to accommodate your application code and data.
5. Peripheral Requirements
Identify the peripheral interfaces required for your project, such as ADC, USART, SPI, or I2C. Verify that the PIC16F877A supports the necessary peripherals and has enough I/O pins to interface with external devices.
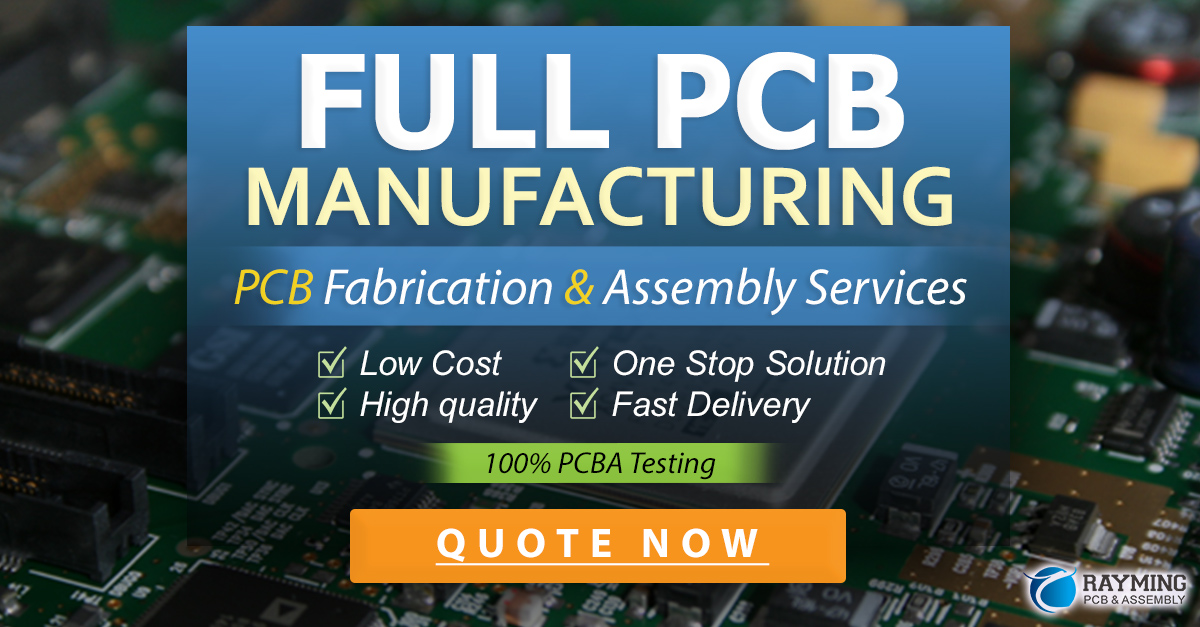
Getting Started with the PIC16F877A
To begin developing with the PIC16F877A, you’ll need the following:
-
Development Board: A development board provides a convenient way to prototype and test your application. It typically includes the PIC16F877A microcontroller, power supply, programming interface, and basic peripherals.
-
Programmer: A programmer is required to load your application code into the microcontroller’s flash memory. Popular programmers for the PIC16F877A include the PICkit and ICD (In-Circuit Debugger) from Microchip.
-
Integrated Development Environment (IDE): An IDE is a software tool that provides a comprehensive environment for writing, compiling, and debugging your code. Microchip offers the MPLAB X IDE, which supports the PIC16F877A and provides features like code editing, simulation, and debugging.
-
Compiler: A compiler is necessary to convert your high-level programming language (such as C or Assembly) into machine code that can be executed by the microcontroller. The MPLAB XC8 compiler is commonly used for the PIC16F877A.
-
Documentation: Refer to the PIC16F877A datasheet and application notes provided by Microchip for detailed information on the microcontroller’s features, pin configuration, and programming guidelines.
Example Application: Temperature Monitoring System
Let’s consider an example application to demonstrate the usage of the PIC16F877A microcontroller. In this project, we’ll design a temperature monitoring system that measures the ambient temperature using a temperature sensor and displays the readings on an LCD.
Hardware Components
- PIC16F877A microcontroller
- LM35 temperature sensor
- 16×2 character LCD
- 10 kΩ potentiometer for LCD contrast adjustment
- Resistors and capacitors for the power supply and pull-up resistors
Software Components
- MPLAB X IDE
- MPLAB XC8 compiler
- PIC16F877A header files and libraries
Circuit Diagram
PIC16F877A
+-----------+
| |
| RA0/AN0 |<--- LM35 Temperature Sensor
| |
| RB0 |--> LCD D0
| RB1 |--> LCD D1
| RB2 |--> LCD D2
| RB3 |--> LCD D3
| RB4 |--> LCD D4
| RB5 |--> LCD D5
| RB6 |--> LCD D6
| RB7 |--> LCD D7
| |
| RE0 |--> LCD RS
| RE1 |--> LCD R/W
| RE2 |--> LCD E
| |
+-----------+
Code Snippet
#include <xc.h>
#include <stdio.h>
#include "lcd.h"
#define LM35_PIN RA0
#define ADC_RESOLUTION 1023
#define ADC_REFERENCE_VOLTAGE 5.0
#define LM35_RESOLUTION 10.0
void main(void) {
TRISA = 0xFF; // Set PORTA as input
TRISE = 0x00; // Set PORTE as output
LCD_Init(); // Initialize the LCD
while (1) {
unsigned int adcValue = ADC_Read(LM35_PIN);
float voltage = (adcValue * ADC_REFERENCE_VOLTAGE) / ADC_RESOLUTION;
float temperature = voltage * LM35_RESOLUTION;
char tempString[16];
sprintf(tempString, "Temp: %.1f C", temperature);
LCD_Clear();
LCD_SetCursor(0, 0);
LCD_WriteString(tempString);
__delay_ms(500);
}
}
In this code snippet, we initialize the necessary pins for the LM35 temperature sensor and the LCD. The ADC_Read
function reads the analog value from the LM35 sensor connected to the RA0
pin. The analog value is then converted to voltage and subsequently to temperature using the LM35’s resolution of 10 mV/°C.
The temperature value is then displayed on the LCD using the LCD_WriteString
function. The LCD is cleared and updated every 500 milliseconds.
Frequently Asked Questions (FAQ)
-
What is the maximum operating frequency of the PIC16F877A?
The PIC16F877A can operate at a maximum frequency of 20 MHz. -
How much program memory does the PIC16F877A have?
The PIC16F877A has 14 KB of flash program memory. -
What is the ADC resolution of the PIC16F877A?
The PIC16F877A features an 8-channel, 10-bit Analog-to-Digital Converter (ADC). -
Can the PIC16F877A be programmed using the ICSP (In-Circuit Serial Programming) method?
Yes, the PIC16F877A supports ICSP, allowing you to program the microcontroller without removing it from the circuit board. -
What is the operating voltage range of the PIC16F877A?
The PIC16F877A can operate at voltages ranging from 2.0V to 5.5V.
Conclusion
The PIC16F877A microcontroller is a versatile and powerful choice for a wide range of embedded applications. Its extensive peripheral set, ease of programming, and low power consumption make it an attractive option for engineers and hobbyists alike.
When selecting a PIC16F877A for your project, consider factors such as package type, temperature range, oscillator type, memory requirements, and peripheral requirements. By carefully evaluating these aspects, you can ensure that you choose the most suitable microcontroller for your specific application.
Getting started with the PIC16F877A involves setting up a development environment, including a development board, programmer, IDE, and compiler. With the right tools and resources, you can begin developing your application and leveraging the capabilities of this powerful microcontroller.
Whether you’re working on a temperature monitoring system, a motor control application, or a data acquisition project, the PIC16F877A provides a solid foundation for building reliable and efficient embedded solutions. By understanding its features, selecting the right variant, and following best practices for development, you can unlock the full potential of this microcontroller and bring your projects to life.
Leave a Reply