Introduction to the MCP41010 Digital Potentiometer
The MCP41010 is a single-channel digital potentiometer (digipot) that provides 256 taps/positions and is controlled via the SPI interface. It acts as a digitally-controlled variable resistor. The resistance between the wiper terminal and the two end terminals can be adjusted by sending digital commands from a microcontroller like an Arduino.
Some key features of the MCP41010 include:
- 256 taps/wiper positions
- Single 2.7V to 5.5V supply
- Resistances available: 5kΩ, 10kΩ, 50kΩ, 100kΩ
- 14-pin TSSOP or 14-pin DIP package
- SPI interface (mode 0,0)
- 3-wire interface: SI (Serial In), SCK (Serial Clock), CS̄ (Chip Select)
- Maximum wiper current: 1 mA
- Power-on reset to midscale position
- Nonvolatile memory to store last wiper position
The MCP41010 is useful in applications requiring digital control of analog circuits, such as:
- Volume/gain controls
- Calibration/trimming circuits
- Programmable filters and oscillators
- Programmable voltage/current sources
- Sensor calibration
- LCD contrast adjustment
Pinout and Schematic for Connecting MCP41010 to Arduino
To use the MCP41010 with an Arduino (or any microcontroller), you need to connect the SPI pins as follows:
MCP41010 Pin | Arduino Pin | Function |
---|---|---|
1 (CS̄) | 10 | SPI Chip Select |
2 (SCK) | 13 | SPI Serial Clock |
3 (SI) | 11 | SPI Serial Data Input |
7 (VDD) | 5V | Power |
14 (VSS) | GND | Ground |
The potentiometer terminals are:
- Pin 5 (PA0): Potentiometer End Terminal A
- Pin 6 (PW0): Potentiometer Wiper Terminal
- Pin 13 (PB0): Potentiometer End Terminal B
Here is a schematic showing the basic connections:
Installing the MCP41xxx Arduino Library
To make interfacing the MCP41010 with Arduino easier, you can use the MCP41xxx library written by Connor Nishijima. This abstracts away the low-level SPI commands.
To install the library:
- In the Arduino IDE, go to Sketch > Include Library > Manage Libraries
- Search for “MCP41xxx”
- Click on the entry for “MCP41xxx by Connor Nishijima”
- Click “Install”
Once installed, you can include the library in your sketch with:
#include <MCP41xxx.h>
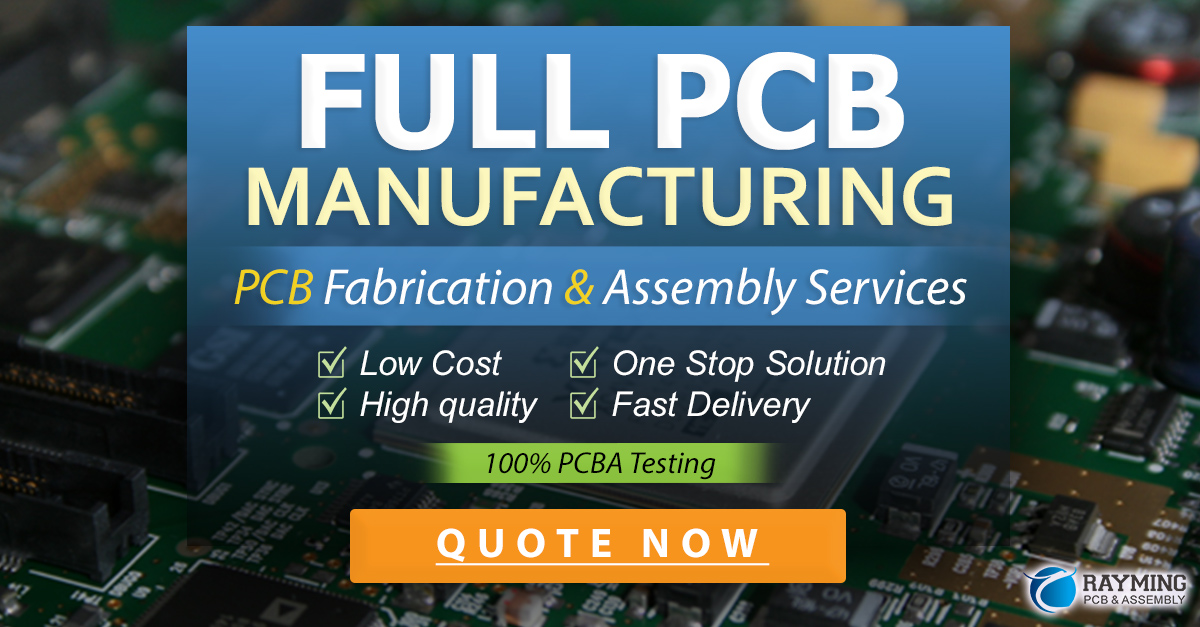
Basic Usage Example: Controlling the Wiper Position
Here’s a simple example sketch that demonstrates how to set the wiper position of the MCP41010 using the MCP41xxx library:
#include <MCP41xxx.h>
// Create MCP41010 object with CS pin 10 and 10K total resistance
MCP41xxx mcp(10, 10000, MCP41xxx::P10K);
void setup() {
mcp.begin();
}
void loop() {
// Ramp up wiper position from 0 to max
for (int i = 0; i <= 255; i++) {
mcp.setValue(i);
delay(10);
}
// Ramp down wiper position from max to 0
for (int i = 255; i >= 0; i--) {
mcp.setValue(i);
delay(10);
}
}
Key points:
- The
MCP41xxx
constructor takes 3 arguments: - The Arduino pin number connected to the CS̄ pin of the MCP41010 (10 in this case)
- The total end-to-end resistance (10,000 ohms or 10K)
- The potentiometer model (
MCP41xxx::P10K
for the 10K version) - Call
begin()
insetup()
to initialize SPI - The
setValue()
function sets the wiper position, from 0 to 255 - The wiper value is stored in nonvolatile memory, so it will retain its position even after a power cycle
Reading the Wiper Position as a Voltage
In this example, we will read the voltage on the MCP41010’s wiper pin using one of the Arduino’s analog inputs:
#include <MCP41xxx.h>
const int POT_PIN = A0;
MCP41xxx mcp(10, 10000, MCP41xxx::P10K);
void setup() {
mcp.begin();
Serial.begin(9600);
}
void loop() {
// Set a random wiper position
int wiperValue = random(0, 256);
mcp.setValue(wiperValue);
// Read the voltage on the wiper pin
int wiperVoltage = analogRead(POT_PIN);
// Convert to voltage
float voltage = wiperVoltage * (5.0 / 1023.0);
Serial.print("Wiper value: ");
Serial.print(wiperValue);
Serial.print(", Voltage: ");
Serial.println(voltage);
delay(1000);
}
In this sketch:
- We connect the MCP41010’s wiper pin to analog input A0 on the Arduino
- Each loop, we set the wiper to a random position between 0-255 using
setValue()
- We read the voltage on A0 using
analogRead()
, which returns a value from 0-1023 corresponding to 0-5V - We convert this 0-1023 value to an actual voltage using the formula:
voltage = wiperVoltage * (5.0 / 1023.0)
- Finally, we print out both the wiper value and measured voltage over serial
Generating a Variable Voltage with the MCP41010
Building on the previous example, we can use the MCP41010 as a programmable voltage source or digital-to-analog converter (DAC).
To generate a variable voltage:
- Connect one end terminal (PA0) to ground
- Connect the other end terminal (PB0) to the Arduino’s 5V output or another reference voltage
- Connect the wiper (PW0) to the load
By changing the wiper position digitally, you can generate a variable voltage between 0V and the reference voltage. The voltage will be proportional to the wiper position.
For example, if using a 5V reference and a wiper value of 127 (midpoint), the output voltage would be approximately:
Vout = (127 / 255) * 5V = 2.49V
And here is a sketch to generate a 0-5V triangle wave:
#include <MCP41xxx.h>
MCP41xxx mcp(10, 10000, MCP41xxx::P10K);
void setup() {
mcp.begin();
}
void loop() {
// Ramp up voltage from 0 to 5V
for (int i = 0; i <= 255; i++) {
mcp.setValue(i);
delay(10);
}
// Ramp down voltage from 5V to 0V
for (int i = 255; i >= 0; i--) {
mcp.setValue(i);
delay(10);
}
}
FAQ
What is the resolution of the MCP41010?
The MCP41010 has 256 taps or wiper positions, providing 8-bit resolution. This means it can divide the end-to-end resistance into 256 steps.
How much current can the MCP41010 handle?
The maximum current that can flow through the MCP41010’s wiper terminal is 1mA. For higher current applications, you may need to use the digipot to control an external buffer circuit.
Is the MCP41010 volatile?
No, the MCP41010 has nonvolatile memory that stores the last wiper position. It will retain this position even after power is removed and restored.
Can I use multiple MCP41010s with one Arduino?
Yes, you can connect multiple MCP41010s to the same SPI bus (MOSI, MISO, SCK lines). Just make sure each one has its own unique CS̄ (chip select) line connected to a different Arduino pin.
What is the difference between the MCP41010 and MCP41050 or MCP41100?
The difference is the total end-to-end resistance:
- MCP41010: 10 kΩ
- MCP41050: 50 kΩ
- MCP41100: 100 kΩ
Otherwise, they have identical functionality. Choose the resistance that best suits your application.
Conclusion
The MCP41010 is a versatile digital potentiometer that is easy to control from an Arduino or other microcontroller using SPI. With its 256 positions, nonvolatile memory, and simple digital interface, it is useful in a wide variety of applications needing digitally-controlled resistance.
We’ve covered the basics of wiring it up and using it from Arduino sketches. The MCP41xxx library provides a user-friendly abstraction layer, while still allowing low-level SPI access if needed.
Whether generating variable voltages, trimming sensors, or making a digital volume control, the MCP41010 is a handy component to have in your toolkit when you need precise, programmable resistance under digital control.
Leave a Reply