What is a CAN Controller?
A CAN (Controller Area Network) controller is a device that manages the communication between nodes on a CAN bus. It handles the transmission and reception of CAN frames, ensuring reliable and efficient data exchange. The CAN controller takes care of low-level tasks such as bit timing, error detection, and message filtering, allowing the host microcontroller to focus on higher-level application tasks.
Key Features of the MCP2515
The MCP2515 offers several key features that make it a popular choice for CAN communication:
-
CAN 2.0B Support: The MCP2515 is fully compliant with the CAN 2.0B specification, supporting both standard (11-bit) and extended (29-bit) message identifiers.
-
High-Speed CAN: It supports CAN bit rates up to 1 Mbps, enabling high-speed communication in demanding applications.
-
Receive Buffers: The MCP2515 provides two receive buffers, each capable of storing a complete CAN frame. This allows efficient handling of incoming messages without overloading the microcontroller.
-
Transmit Buffers: It also features three transmit buffers, enabling the microcontroller to queue up multiple messages for transmission, improving overall system performance.
-
Message Filtering: The MCP2515 supports both mask and filter-based message filtering, allowing the microcontroller to selectively receive messages based on their identifiers. This helps reduce the processing overhead and ensures that only relevant messages are passed to the application.
-
Interrupt Capability: The device generates interrupts for various events, such as message reception, transmission completion, and error conditions. This enables efficient event-driven programming and reduces the need for polling.
-
Low Power Mode: The MCP2515 offers a low power mode that reduces current consumption when the device is not actively communicating on the CAN bus.
MCP2515 Pin Configuration
The MCP2515 is available in various package options, including DIP, SOIC, and QFN. Here’s a table summarizing the pin configuration for the 18-pin SOIC package:
Pin | Name | Description |
---|---|---|
1 | TXCAN | Transmit CAN Output |
2 | RXCAN | Receive CAN Input |
3 | CLKOUT | Clock Output |
4 | TX0RTS | Transmit Buffer 0 Request to Send |
5 | TX1RTS | Transmit Buffer 1 Request to Send |
6 | TX2RTS | Transmit Buffer 2 Request to Send |
7 | OSC2 | Oscillator Input/Output |
8 | OSC1 | Oscillator Input/Output |
9 | VSS | Ground |
10 | RX1BF | Receive Buffer 1 Full Flag |
11 | RX0BF | Receive Buffer 0 Full Flag |
12 | INT | Interrupt Output |
13 | SCK | SPI Clock Input |
14 | SI | SPI Data Input |
15 | SO | SPI Data Output |
16 | CS | SPI Chip Select Input |
17 | RESET | Reset Input |
18 | VDD | Power Supply |
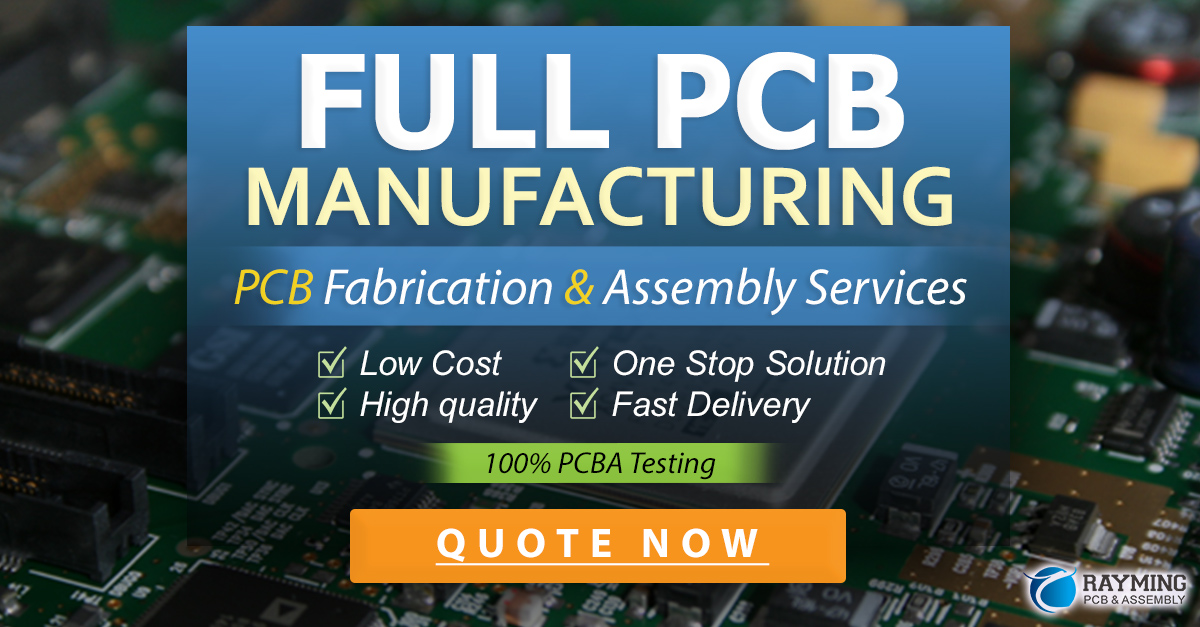
Interfacing with the MCP2515
The MCP2515 communicates with the host microcontroller using the SPI (Serial Peripheral Interface) protocol. SPI is a synchronous, full-duplex communication protocol that requires four signals: MOSI (Master Out Slave In), MISO (Master In Slave Out), SCK (Serial Clock), and CS (Chip Select).
To interface with the MCP2515, follow these steps:
- Connect the MCP2515’s SPI pins (SI, SO, SCK, and CS) to the corresponding SPI pins on your microcontroller.
- Connect the MCP2515’s TXCAN and RXCAN pins to the CAN transceiver, which handles the physical layer of the CAN communication.
- Provide a suitable clock source for the MCP2515, either by connecting an external crystal oscillator or using the microcontroller’s clock output.
- Configure the MCP2515’s operating mode, bit rate, and other settings by writing to its configuration registers via SPI.
- Implement the necessary code on the microcontroller to send and receive CAN messages using the MCP2515’s transmit and receive buffers.
Configuring the MCP2515
The MCP2515 has several configuration registers that control its behavior and operation. Here are some of the key registers:
- CNF1, CNF2, CNF3: These registers set the bit timing parameters for the CAN bus, such as the baud rate prescaler, synchronization jump width, and sample point.
- CANCTRL: This register controls the operating mode of the MCP2515 (normal, sleep, loopback, etc.) and enables/disables features like one-shot mode and CLKOUT.
- CANSTAT: This register provides status information about the MCP2515, such as the current operating mode and error flags.
- TXBnCTRL: These registers control the transmission of messages from the three transmit buffers (n = 0, 1, 2).
- RXBnCTRL: These registers control the reception of messages into the two receive buffers (n = 0, 1) and configure the message filtering.
- RXFnSIDH, RXFnSIDL, RXFnEID8, RXFnEID0: These registers define the filter values for the receive buffers, allowing selective reception of messages based on their identifiers.
- RXMnSIDH, RXMnSIDL, RXMnEID8, RXMnEID0: These registers define the mask values for the receive buffers, determining which bits of the identifier are compared against the filter values.
To configure the MCP2515, you need to write the appropriate values to these registers using SPI commands. The specific values depend on your application requirements, such as the desired CAN bit rate, message filtering criteria, and operating mode.
Sending and Receiving CAN Messages
Once the MCP2515 is properly configured, you can start sending and receiving CAN messages. The process involves the following steps:
- Sending Messages:
- Load the message ID, data length code (DLC), and data bytes into one of the transmit buffers (TXB0, TXB1, or TXB2).
- Set the appropriate bits in the TXBnCTRL register to mark the buffer as ready for transmission.
-
The MCP2515 will automatically transmit the message when the CAN bus is available.
-
Receiving Messages:
- The MCP2515 continuously monitors the CAN bus for incoming messages that match the configured filters.
- When a matching message is received, it is stored in one of the receive buffers (RXB0 or RXB1).
- The corresponding RXnIF flag in the CANINTF register is set to indicate the presence of a new message.
- The microcontroller can then read the message ID, DLC, and data bytes from the receive buffer using SPI commands.
Here’s an example of how to send a CAN message using the MCP2515:
// Load message into TXB0
spi_write(MCP2515_LOAD_TX_BUFFER | 0x00, message_id >> 3);
spi_write(MCP2515_LOAD_TX_BUFFER | 0x01, (message_id << 5) | (message_length & 0x0F));
for (int i = 0; i < message_length; i++) {
spi_write(MCP2515_LOAD_TX_BUFFER | (0x02 + i), message_data[i]);
}
// Request transmission
spi_write(MCP2515_RTS | 0x01, 0);
And here’s an example of how to receive a CAN message:
// Check if a message is available in RXB0
uint8_t status = spi_read(MCP2515_READ_STATUS);
if (status & 0x01) {
// Read message from RXB0
uint8_t message_id_high = spi_read(MCP2515_READ_RX_BUFFER | 0x00);
uint8_t message_id_low = spi_read(MCP2515_READ_RX_BUFFER | 0x01);
uint8_t message_length = message_id_low & 0x0F;
for (int i = 0; i < message_length; i++) {
message_data[i] = spi_read(MCP2515_READ_RX_BUFFER | (0x02 + i));
}
// Clear the receive flag
spi_write(MCP2515_CANINTF, 0x01);
}
Note that these are simplified examples, and the actual implementation may vary depending on your specific microcontroller and application requirements.
Error Handling and Debugging
The MCP2515 provides several mechanisms for error handling and debugging:
-
Error Flags: The EFLG register contains various error flags that indicate the occurrence of errors, such as bit errors, stuff errors, and form errors. Monitoring these flags can help identify and troubleshoot communication issues.
-
Error Counters: The MCP2515 maintains two error counters, TEC (Transmit Error Counter) and REC (Receive Error Counter), which keep track of the number of errors detected during transmission and reception, respectively. These counters can be read from the TEC and REC registers.
-
Interrupt Flags: The CANINTF register contains interrupt flags for various events, such as message reception, transmission completion, and error conditions. By enabling the corresponding interrupt sources in the CANINTE register and monitoring the CANINTF flags, you can implement event-driven error handling and debugging.
-
Loopback Mode: The MCP2515 supports a loopback mode that allows internal testing of the CAN controller without affecting the actual CAN bus. By setting the appropriate bits in the CANCTRL register, you can enable loopback mode and send messages internally for debugging purposes.
When encountering errors or communication issues, consider the following debugging steps:
- Check the wiring and connections between the MCP2515, CAN transceiver, and microcontroller.
- Verify the correct configuration of the MCP2515’s registers, including the bit timing, operating mode, and message filtering settings.
- Monitor the error flags and counters to identify the type and frequency of errors occurring.
- Use the interrupt flags to detect and handle specific events, such as message reception and transmission errors.
- Utilize the loopback mode to isolate and test the MCP2515’s functionality independently of the CAN bus.
By properly handling errors and utilizing the debugging features provided by the MCP2515, you can ensure robust and reliable CAN communication in your application.
Frequently Asked Questions (FAQ)
-
What is the maximum CAN bit rate supported by the MCP2515?
The MCP2515 supports CAN bit rates up to 1 Mbps. -
How many transmit and receive buffers does the MCP2515 have?
The MCP2515 has three transmit buffers (TXB0, TXB1, TXB2) and two receive buffers (RXB0, RXB1). -
Can the MCP2515 filter incoming messages based on their identifiers?
Yes, the MCP2515 supports both mask and filter-based message filtering, allowing selective reception of messages based on their identifiers. -
What is the purpose of the CLKOUT pin on the MCP2515?
The CLKOUT pin provides a programmable clock output that can be used for synchronization or as a reference clock for other devices in the system. -
How do I set the CAN bit rate on the MCP2515?
The CAN bit rate is determined by the values programmed in the CNF1, CNF2, and CNF3 registers. You need to calculate and set the appropriate values based on your desired bit rate and the frequency of the MCP2515’s clock source.
Conclusion
The MCP2515 is a powerful and versatile CAN controller that simplifies the implementation of CAN communication in a wide range of applications. By understanding its features, pin configuration, and interfacing requirements, you can effectively integrate the MCP2515 into your projects and achieve reliable and efficient CAN communication.
Throughout this article, we explored the key aspects of the MCP2515, including its configuration registers, sending and receiving messages, error handling, and debugging techniques. By following the guidelines and examples provided, you can confidently utilize the MCP2515 to establish robust CAN networks and enhance the functionality of your embedded systems.
Remember to carefully consider your application requirements, such as the desired CAN bit rate, message filtering criteria, and error handling strategies, when configuring and working with the MCP2515. Additionally, refer to the MCP2515 datasheet and application notes for more detailed information and specific implementation guidelines.
With its extensive feature set and industry-standard compliance, the MCP2515 is a reliable choice for CAN communication in automotive, industrial, and other demanding environments. By leveraging its capabilities and following best practices, you can develop high-performance and dependable CAN-based systems.
Leave a Reply