Introduction to CircuitPython
CircuitPython is a programming language designed to simplify experimenting and learning to code on low-cost microcontroller boards. It’s a great way to get started with programming microcontrollers, especially for those new to embedded systems. CircuitPython is based on Python, which is widely used in many applications such as web development, data analysis, artificial intelligence, and scientific computing.
What is CircuitPython?
CircuitPython is a variant of Python that has been modified to run on microcontrollers. It’s an open-source project maintained by Adafruit Industries, a company known for its innovative electronic products and educational resources. CircuitPython was created to make it easier for people to start programming microcontrollers without needing to learn complex languages like C or C++.
Advantages of CircuitPython
CircuitPython offers several advantages over traditional embedded programming languages:
-
Easy to learn: If you’re familiar with Python, you’ll find CircuitPython very easy to pick up. Even if you’re new to programming, CircuitPython’s syntax is straightforward and readable.
-
Rapid prototyping: With CircuitPython, you can quickly write and test code without the need for complex toolchains or compilers. Just connect your board to a computer, edit your code, and run it.
-
Large ecosystem: CircuitPython has a growing library of modules and drivers that make it easy to interface with a wide range of sensors, displays, and other peripherals.
-
Cross-platform: CircuitPython runs on Windows, macOS, Linux, and even in web browsers. You can use your favorite text editor to write code and upload it to your board using a simple file copy.
Getting Started with CircuitPython
Supported Boards
CircuitPython supports a wide range of microcontroller boards, including:
Board Family | Examples |
---|---|
Adafruit Feather | Feather M0 Express, Feather nRF52840 Express |
Adafruit ItsyBitsy | ItsyBitsy M0 Express, ItsyBitsy M4 Express |
Adafruit Trinket | Trinket M0 |
Adafruit Circuit Playground | Circuit Playground Express, Circuit Playground Bluefruit |
Adafruit Gemma | Gemma M0 |
Adafruit Metro | Metro M0 Express, Metro M4 Express |
Many other manufacturers also offer CircuitPython-compatible boards, such as SparkFun, Seeed Studio, and Particle.
Installing CircuitPython
To get started with CircuitPython:
-
Download the latest version of CircuitPython for your board from the CircuitPython downloads page.
-
Connect your board to your computer using a USB cable.
-
Double-click the reset button on your board. This will put it into bootloader mode.
-
Drag and drop the CircuitPython .UF2 file onto the BOOT drive that appears.
Your board will reset and a new drive called CIRCUITPY will appear. This is where you’ll store your CircuitPython code.
Your First CircuitPython Program
Let’s write a simple CircuitPython program that blinks the built-in LED on your board:
-
Open your favorite text editor and create a new file called
main.py
on the CIRCUITPY drive. -
Copy and paste the following code into
main.py
:
import board
import digitalio
import time
led = digitalio.DigitalInOut(board.LED)
led.direction = digitalio.Direction.OUTPUT
while True:
led.value = True
time.sleep(0.5)
led.value = False
time.sleep(0.5)
- Save the file. Your board will automatically reset and start running the code.
You should see the built-in LED blinking on and off every half second. Congratulations, you’ve just written your first CircuitPython program!
CircuitPython Libraries
One of the strengths of CircuitPython is its extensive library of modules and drivers that make it easy to interface with various sensors, displays, and other peripherals.
Built-in Libraries
CircuitPython includes several built-in libraries that provide essential functionality:
board
: Provides access to the board’s pins and other hardware features.digitalio
: Allows control of digital input/output pins.analogio
: Enables reading from analog input pins and writing to analog output pins.time
: Provides functions for working with time, such as delays and timers.math
: Includes mathematical functions and constants.random
: Generates random numbers.storage
: Allows access to the board’s file system.
External Libraries
In addition to the built-in libraries, CircuitPython has a growing ecosystem of external libraries contributed by the community. These libraries provide support for a wide range of sensors, displays, and other peripherals.
Some popular external libraries include:
adafruit_bme280
: Supports the BME280 temperature, humidity, and pressure sensor.adafruit_ssd1306
: Allows control of SSD1306-based OLED displays.adafruit_gps
: Provides support for GPS modules.adafruit_motorkit
: Enables control of DC motors and stepper motors.adafruit_lis3dh
: Supports the LIS3DH accelerometer.
You can find a full list of CircuitPython libraries on the CircuitPython Libraries page.
Installing External Libraries
To use an external library in your CircuitPython project:
-
Download the library from the CircuitPython Libraries page or the library’s GitHub repository.
-
Unzip the downloaded file.
-
Copy the library folder into the
lib
folder on your CIRCUITPY drive. -
Import the library in your
main.py
file and start using it.
For example, to use the adafruit_bme280
library:
-
Download the library from the adafruit_bme280 GitHub repository.
-
Unzip the downloaded file.
-
Copy the
adafruit_bme280
folder into thelib
folder on your CIRCUITPY drive. -
In your
main.py
file, import the library and use it:
import board
import adafruit_bme280
i2c = board.I2C()
bme280 = adafruit_bme280.Adafruit_BME280_I2C(i2c)
print("Temperature: {:.2f} C".format(bme280.temperature))
print("Humidity: {:.2f} %".format(bme280.humidity))
print("Pressure: {:.2f} hPa".format(bme280.pressure))
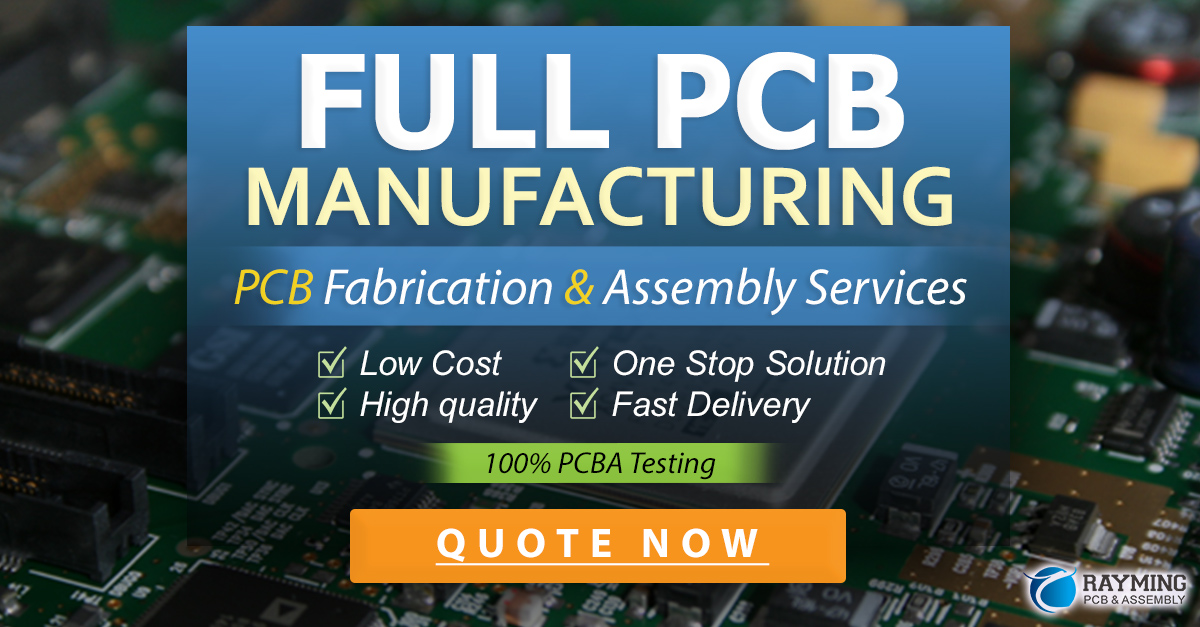
CircuitPython Projects
Now that you know the basics of CircuitPython, let’s explore some projects you can build with it.
Temperature and Humidity Display
In this project, we’ll use a BME280 sensor and an SSD1306 OLED display to create a temperature and humidity display.
Hardware
- Adafruit Feather M0 Express
- BME280 sensor
- SSD1306 OLED display
- Breadboard
- Jumper wires
Software
-
Install the
adafruit_bme280
andadafruit_ssd1306
libraries as described in the “Installing External Libraries” section. -
Create a new file called
main.py
on your CIRCUITPY drive and copy the following code:
import time
import board
import adafruit_bme280
import adafruit_ssd1306
i2c = board.I2C()
bme280 = adafruit_bme280.Adafruit_BME280_I2C(i2c)
display = adafruit_ssd1306.SSD1306_I2C(128, 32, i2c)
while True:
display.fill(0)
display.text("Temp: {:.2f} C".format(bme280.temperature), 0, 0, 1)
display.text("Hum: {:.2f} %".format(bme280.humidity), 0, 10, 1)
display.show()
time.sleep(1)
-
Connect the BME280 sensor and the SSD1306 display to your Feather M0 Express according to the library documentation.
-
Save the file and your project should start running, displaying the current temperature and humidity on the OLED display.
LED Pulse Animation
This project creates a pulsing LED animation using the board’s built-in LED and PWM (Pulse Width Modulation).
Hardware
- Adafruit Circuit Playground Express
Software
- Create a new file called
main.py
on your CIRCUITPY drive and copy the following code:
import time
import board
import pwmio
led = pwmio.PWMOut(board.D13)
while True:
for i in range(100):
led.duty_cycle = int(i / 100 * 65535)
time.sleep(0.01)
for i in range(100, -1, -1):
led.duty_cycle = int(i / 100 * 65535)
time.sleep(0.01)
- Save the file and your project should start running, creating a pulsing animation on the built-in LED.
Troubleshooting and FAQ
My board isn’t showing up as a CIRCUITPY drive. What should I do?
Make sure your board is supported by CircuitPython and that you’ve installed the correct version of CircuitPython for your board. Double-check that you’ve put the board into bootloader mode by double-clicking the reset button.
I’m getting an error message when I try to import a library. How can I fix this?
Ensure that you’ve installed the library correctly by copying it into the lib
folder on your CIRCUITPY drive. Check that the library is compatible with your version of CircuitPython.
Can I use CircuitPython with Arduino boards?
Some Arduino boards, like the Arduino Zero and the Arduino MKR series, are compatible with CircuitPython. However, most Arduino boards use a different microcontroller architecture and are not supported by CircuitPython.
How do I update CircuitPython on my board?
To update CircuitPython, download the latest version from the CircuitPython downloads page and follow the same installation process as when you first installed CircuitPython.
Where can I find more CircuitPython projects and resources?
The Adafruit Learning System offers a wide range of CircuitPython projects, tutorials, and guides. The CircuitPython community forum is also a great place to ask questions and share projects.
Conclusion
CircuitPython is a powerful and easy-to-use platform for programming microcontrollers. Its beginner-friendly syntax, extensive libraries, and supportive community make it an excellent choice for anyone interested in learning embedded systems or working on microcontroller projects.
In this article, we’ve covered the basics of CircuitPython, from installation and setup to libraries and projects. By following the examples and exploring the resources provided, you’ll be well on your way to creating your own amazing CircuitPython projects.
So why wait? Grab a CircuitPython-compatible board, start coding, and unleash your creativity!
Leave a Reply