Introduction to Arduino Ultrasonic Sensor
The Arduino Ultrasonic Sensor, specifically the HC-SR04 model, is a popular choice among hobbyists and professionals alike for its ability to accurately measure distances using high-frequency sound waves. This sensor is widely used in various applications, such as robotics, automation, and object detection systems. In this comprehensive guide, we will delve into the working principles, specifications, and practical applications of the HC-SR04 ultrasonic sensor, as well as provide step-by-step instructions on how to integrate it with an Arduino board.
How Does the HC-SR04 Ultrasonic Sensor Work?
The HC-SR04 ultrasonic sensor operates on the principle of echo ranging, similar to the way bats or dolphins navigate their surroundings. The sensor consists of two main components: an ultrasonic transmitter and an ultrasonic receiver. Here’s a breakdown of how the sensor works:
- The ultrasonic transmitter emits a high-frequency sound wave (typically at 40 kHz) when triggered.
- The sound wave travels through the air until it encounters an object in its path.
- Upon hitting the object, the sound wave reflects back towards the sensor.
- The ultrasonic receiver detects the reflected sound wave.
- The time taken for the sound wave to travel from the transmitter to the object and back to the receiver is measured by the sensor.
- Using this time and the known speed of sound in air, the distance between the sensor and the object can be calculated.
The HC-SR04 ultrasonic sensor has a range of approximately 2 cm to 400 cm (1″ to 13 feet), with an accuracy of about 3 mm. It is important to note that the sensor’s performance can be affected by factors such as temperature, humidity, and the surface properties of the objects being detected.
HC-SR04 Ultrasonic Sensor Specifications
To effectively use the HC-SR04 ultrasonic sensor, it is essential to understand its technical specifications. The following table summarizes the key specifications of the sensor:
Parameter | Value |
---|---|
Operating Voltage | 5V DC |
Operating Current | 15 mA |
Ultrasonic Frequency | 40 kHz |
Minimum Measuring Distance | 2 cm (1″) |
Maximum Measuring Distance | 400 cm (13 feet) |
Measurement Angle | 15 degrees |
Trigger Pulse Width | 10 µs |
Dimensions | 45 mm x 20 mm x 15 mm |
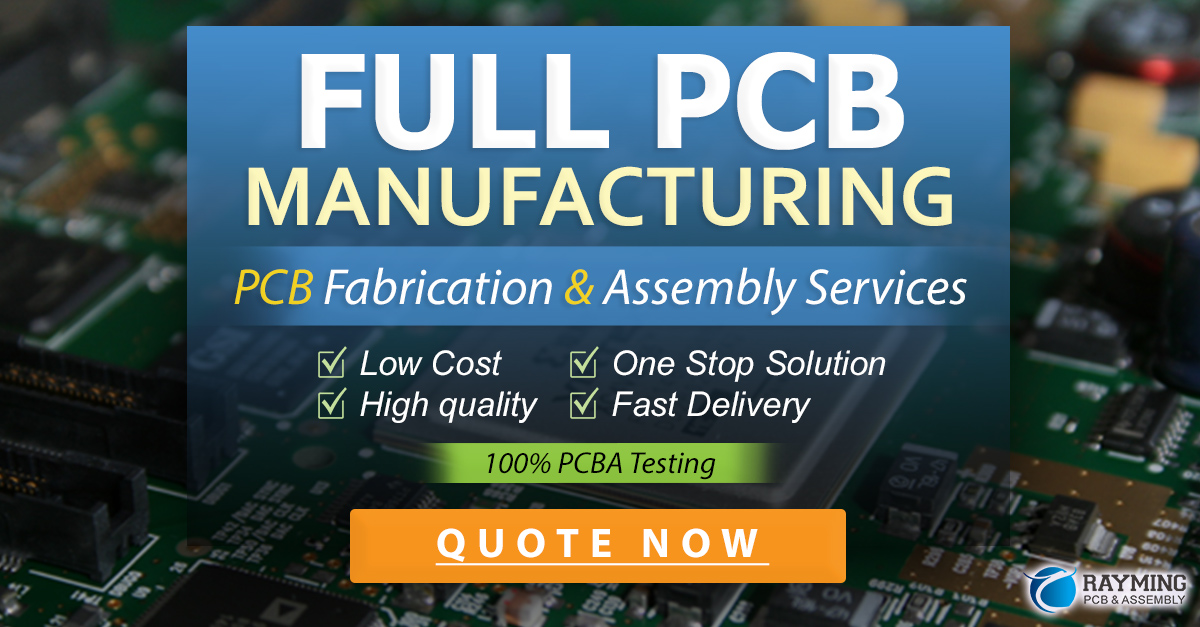
Connecting the HC-SR04 Ultrasonic Sensor to an Arduino Board
To start using the HC-SR04 ultrasonic sensor with an Arduino board, follow these steps:
- Identify the four pins on the HC-SR04 sensor: VCC (power), Trig (trigger), Echo (receive), and GND (ground).
- Connect the VCC pin to the 5V pin on the Arduino board.
- Connect the Trig pin to any digital pin on the Arduino board (e.g., pin 9).
- Connect the Echo pin to any digital pin on the Arduino board (e.g., pin 10).
- Connect the GND pin to the GND pin on the Arduino board.
Here’s a simple wiring diagram illustrating the connections:
HC-SR04 Arduino
-----------------------
VCC 5V
Trig Pin 9
Echo Pin 10
GND GND
Arduino Code for Distance Measurement Using HC-SR04
Now that the HC-SR04 sensor is connected to the Arduino board, let’s write a simple Arduino sketch to measure distances and display the results on the serial monitor.
const int trigPin = 9;
const int echoPin = 10;
void setup() {
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
Serial.begin(9600);
}
void loop() {
// Send a 10 µs trigger pulse
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Measure the duration of the echo pulse
long duration = pulseIn(echoPin, HIGH);
// Calculate the distance in centimeters
float distance = duration * 0.034 / 2;
// Print the distance on the serial monitor
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(500);
}
In this code:
- We define the
trigPin
andechoPin
variables to store the pin numbers connected to the Trig and Echo pins of the HC-SR04 sensor. - In the
setup()
function, we set thetrigPin
as an output and theechoPin
as an input. We also initialize the serial communication at a baud rate of 9600. - In the
loop()
function, we send a 10 µs trigger pulse to the HC-SR04 sensor by setting thetrigPin
HIGH for 10 µs and then LOW. - We measure the duration of the echo pulse using the
pulseIn()
function, which waits for theechoPin
to go HIGH and returns the duration in microseconds. - We calculate the distance in centimeters using the formula:
distance = duration * 0.034 / 2
, where 0.034 is the speed of sound in cm/µs divided by 2 (to account for the round trip of the sound wave). - Finally, we print the calculated distance on the serial monitor and add a small delay of 500 ms before the next measurement.
Upload this sketch to your Arduino board, open the serial monitor, and you should see the distance measurements being displayed in real-time.
Practical Applications of the HC-SR04 Ultrasonic Sensor
The HC-SR04 ultrasonic sensor finds applications in a wide range of projects and industries. Some common use cases include:
- Robotics: The sensor can be used in robotic navigation systems to detect obstacles and avoid collisions.
- Parking Sensors: HC-SR04 sensors are often used in parking assistance systems to help drivers gauge the distance between their vehicle and nearby objects.
- Level Measurement: The sensor can be employed to measure the level of liquids or solids in containers, such as water tanks or grain silos.
- Security Systems: Ultrasonic sensors can detect the presence of intruders or unauthorized personnel in restricted areas.
- Interactive Installations: Artists and designers use HC-SR04 sensors to create interactive exhibits and installations that respond to the presence and movement of people.
These are just a few examples of the many applications where the HC-SR04 ultrasonic sensor can be utilized. Its versatility, low cost, and ease of use make it a popular choice for a wide range of projects.
Tips and Tricks for Using the HC-SR04 Ultrasonic Sensor
To get the most out of your HC-SR04 ultrasonic sensor, consider the following tips and tricks:
- Mounting: Ensure that the sensor is mounted securely and oriented correctly to achieve accurate and consistent readings.
- Calibration: Perform calibration tests to determine the sensor’s accuracy and make any necessary adjustments to your code or setup.
- Temperature Compensation: The speed of sound varies with temperature, so consider implementing temperature compensation in your code for more precise measurements.
- Multiple Sensors: If your project requires monitoring distances in multiple directions, you can use multiple HC-SR04 sensors connected to different pins on your Arduino board.
- Filtering: To minimize false readings caused by noise or interference, implement filtering techniques such as averaging multiple measurements or using a median filter.
By keeping these tips in mind, you can improve the performance and reliability of your HC-SR04 ultrasonic sensor in your Arduino projects.
Frequently Asked Questions (FAQ)
-
What is the maximum range of the HC-SR04 ultrasonic sensor?
The HC-SR04 ultrasonic sensor has a maximum range of approximately 400 cm (13 feet). -
Can the HC-SR04 sensor detect objects of any material?
The sensor can detect most solid objects, but its performance may vary depending on the surface properties of the object. Soft, curved, or thin objects may be more challenging to detect accurately. -
How many HC-SR04 sensors can I connect to an Arduino board?
The number of HC-SR04 sensors you can connect depends on the number of available digital pins on your Arduino board. Each sensor requires two digital pins (one for triggering and one for receiving the echo pulse). -
Can I use the HC-SR04 sensor with other microcontrollers besides Arduino?
Yes, the HC-SR04 sensor can be used with other microcontrollers, such as Raspberry Pi or ESP32, as long as they have compatible digital pins and can provide the necessary 5V power supply. -
How do I troubleshoot if my HC-SR04 sensor is not working properly?
First, double-check your wiring connections to ensure that the sensor is connected correctly to your Arduino board. If the wiring is correct, verify that your code is properly configured with the correct pin assignments and timing parameters. You can also try testing the sensor with a simple example sketch to isolate any issues.
Conclusion
The HC-SR04 ultrasonic sensor is a powerful and versatile tool for measuring distances and detecting objects in a wide range of Arduino projects. By understanding its working principles, specifications, and practical applications, you can effectively integrate this sensor into your designs and create innovative solutions. With the step-by-step guide and tips provided in this article, you are now equipped to start exploring the possibilities of the HC-SR04 sensor and take your Arduino projects to the next level.
Leave a Reply