Introduction to Arduino Byte
Arduino is an open-source electronics platform that has revolutionized the world of hardware programming. It provides an easy-to-use environment for creating interactive projects and prototypes. One of the fundamental data types in Arduino programming is the byte type. In this comprehensive guide, we will dive deep into the Arduino byte type, exploring its characteristics, usage, and practical applications.
What is a Byte in Arduino?
In Arduino, a byte is an 8-bit unsigned data type. It can store integer values ranging from 0 to 255. The byte type is commonly used when working with raw binary data, such as reading from or writing to sensors, communicating with other devices, or storing small amounts of data efficiently.
Data Type | Size (bits) | Range |
---|---|---|
byte | 8 | 0 to 255 |
Declaring and Initializing Byte Variables
To use a byte variable in your Arduino sketch, you need to declare it and optionally initialize it with a value. Here’s the syntax for declaring and initializing a byte variable:
byte variableName; // Declaration
byte variableName = value; // Declaration and initialization
For example:
byte ledPin = 13;
byte sensorValue;
Byte Arithmetic and Operations
Arduino supports various arithmetic and bitwise operations on byte variables. Here are some commonly used operations:
Operation | Description |
---|---|
+ |
Addition |
- |
Subtraction |
* |
Multiplication |
/ |
Division |
% |
Modulo (remainder) |
++ |
Increment by 1 |
-- |
Decrement by 1 |
& |
Bitwise AND |
| |
Bitwise OR |
^ |
Bitwise XOR |
~ |
Bitwise NOT |
<< |
Left shift |
>> |
Right shift |
Here’s an example that demonstrates some arithmetic operations on byte variables:
byte a = 100;
byte b = 50;
byte sum = a + b; // Addition
byte diff = a - b; // Subtraction
byte product = a * 2; // Multiplication
byte quotient = a / 3; // Division
byte remainder = a % 3; // Modulo
Byte Overflow and Underflow
When performing arithmetic operations on byte variables, it’s important to be aware of overflow and underflow conditions. If the result of an operation exceeds the maximum value of 255 or goes below 0, the value will wrap around.
For example:
byte maxValue = 255;
byte overflowResult = maxValue + 1; // overflowResult will be 0
In the above code, adding 1 to the maximum value of 255 causes an overflow, and the result wraps around to 0.
Byte I/O Operations
Reading and Writing Bytes
Arduino provides functions to read and write byte values from/to various sources, such as digital and analog pins, serial communication, and external devices.
Digital I/O
To read or write a byte value from/to a digital pin, you can use the digitalRead()
and digitalWrite()
functions.
byte ledPin = 13;
byte buttonPin = 2;
void setup() {
pinMode(ledPin, OUTPUT);
pinMode(buttonPin, INPUT_PULLUP);
}
void loop() {
byte buttonState = digitalRead(buttonPin);
digitalWrite(ledPin, buttonState);
}
In this example, the state of a button connected to digital pin 2 is read using digitalRead()
, and the value is written to an LED connected to digital pin 13 using digitalWrite()
.
Analog I/O
To read an analog value as a byte, you can use the analogRead()
function. The function returns a 10-bit value (0-1023), which can be scaled down to a byte range (0-255) using the map()
function.
byte sensorPin = A0;
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(sensorPin);
byte mappedValue = map(sensorValue, 0, 1023, 0, 255);
Serial.println(mappedValue);
delay(100);
}
In this example, an analog sensor connected to analog pin A0 is read using analogRead()
. The 10-bit value is then mapped to a byte range using map()
, and the resulting byte value is printed to the serial monitor.
Serial Communication
Arduino boards can communicate with other devices using serial communication protocols such as UART, I2C, and SPI. Byte values can be sent and received over these communication channels.
UART (Serial)
The Arduino IDE provides a built-in serial monitor that allows you to send and receive data over the USB connection. You can use the Serial
object to read and write byte values.
void setup() {
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
byte receivedByte = Serial.read();
Serial.print("Received: ");
Serial.println(receivedByte);
}
}
In this example, when a byte is received over the serial connection, it is read using Serial.read()
and printed back to the serial monitor.
I2C
I2C (Inter-Integrated Circuit) is a communication protocol commonly used to communicate with sensors and other devices. Arduino boards can act as an I2C master and communicate with I2C slave devices.
#include <Wire.h>
#define SLAVE_ADDRESS 0x68
void setup() {
Wire.begin();
}
void loop() {
Wire.beginTransmission(SLAVE_ADDRESS);
Wire.write(0x42);
byte receivedByte = Wire.read();
Wire.endTransmission();
Serial.print("Received: ");
Serial.println(receivedByte);
delay(1000);
}
In this example, the Arduino board communicates with an I2C slave device at address 0x68. It sends a byte value of 0x42 using Wire.write()
and then reads a byte value from the slave using Wire.read()
. The received byte is printed to the serial monitor.
SPI
SPI (Serial Peripheral Interface) is another communication protocol used for high-speed data transfer between the Arduino and peripheral devices.
#include <SPI.h>
#define SLAVE_SELECT_PIN 10
void setup() {
SPI.begin();
pinMode(SLAVE_SELECT_PIN, OUTPUT);
digitalWrite(SLAVE_SELECT_PIN, HIGH);
}
void loop() {
byte data = 0x55;
digitalWrite(SLAVE_SELECT_PIN, LOW);
byte receivedByte = SPI.transfer(data);
digitalWrite(SLAVE_SELECT_PIN, HIGH);
Serial.print("Received: ");
Serial.println(receivedByte, HEX);
delay(1000);
}
In this example, the Arduino board communicates with an SPI slave device. It sends a byte value of 0x55 using SPI.transfer()
and receives a byte value from the slave in return. The received byte is printed to the serial monitor in hexadecimal format.
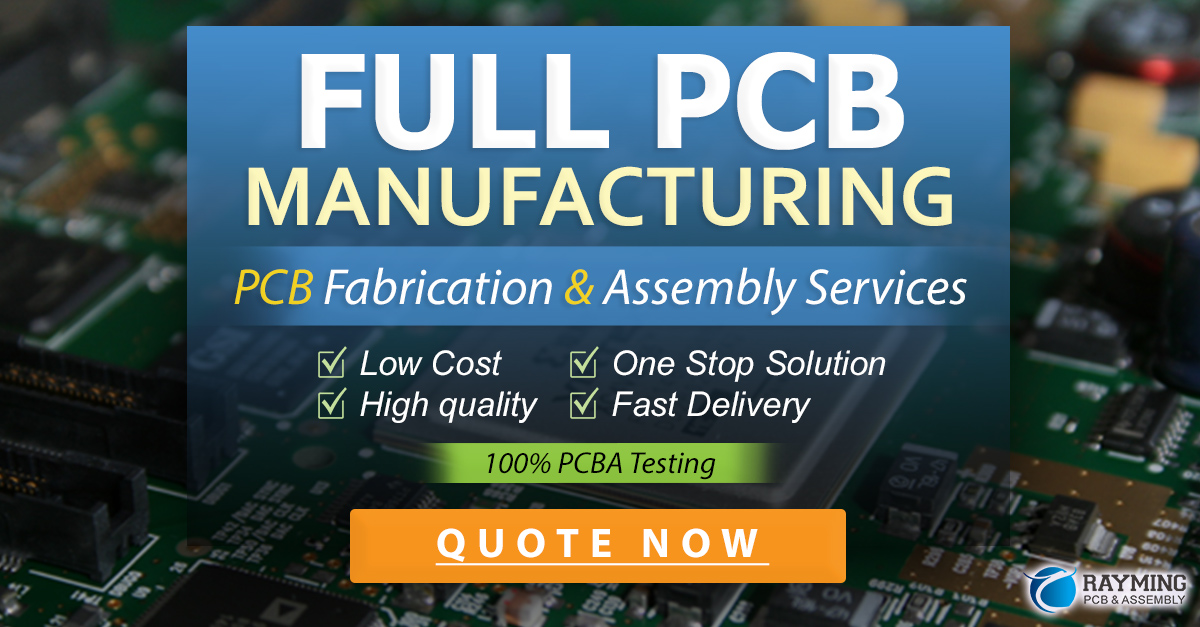
Byte Arrays and Buffers
Creating and Accessing Byte Arrays
Byte arrays are used to store multiple byte values in a contiguous block of memory. You can create a byte array by specifying the size of the array during declaration.
byte myArray[5]; // Declares an array of 5 bytes
To access individual elements of a byte array, you can use the array indexing operator []
. The index starts from 0 for the first element.
myArray[0] = 10; // Assigns value 10 to the first element
myArray[1] = 20; // Assigns value 20 to the second element
byte value = myArray[0]; // Retrieves the value of the first element
Byte Buffers for Data Storage
Byte arrays can be used as buffers to store and manipulate data efficiently. Buffers are commonly used when working with sensor data, communication protocols, or file storage.
Here’s an example of using a byte buffer to store sensor data:
#define BUFFER_SIZE 10
byte sensorBuffer[BUFFER_SIZE];
int bufferIndex = 0;
void setup() {
Serial.begin(9600);
}
void loop() {
if (bufferIndex < BUFFER_SIZE) {
sensorBuffer[bufferIndex] = analogRead(A0) >> 2; // Store 8-bit sensor value
bufferIndex++;
} else {
for (int i = 0; i < BUFFER_SIZE; i++) {
Serial.print(sensorBuffer[i]);
Serial.print(" ");
}
Serial.println();
bufferIndex = 0;
}
delay(100);
}
In this example, a byte buffer sensorBuffer
of size 10 is used to store sensor readings. The analogRead()
function is used to read a 10-bit value from analog pin A0, which is then shifted right by 2 bits to fit into a byte (8 bits). The sensor values are stored in the buffer until it is full. Once the buffer is full, its contents are printed to the serial monitor, and the buffer index is reset to start storing values from the beginning again.
Practical Applications of Byte Type
Sensor Data Processing
Byte variables are commonly used when working with sensor data. Many sensors communicate their readings as byte values, and Arduino needs to interpret and process these values accordingly.
Here’s an example of reading temperature and humidity data from a DHT11 sensor using byte variables:
#include <DHT.h>
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
byte temperature = dht.readTemperature();
byte humidity = dht.readHumidity();
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print(" °C, Humidity: ");
Serial.print(humidity);
Serial.println("%");
delay(2000);
}
In this example, the DHT library is used to read temperature and humidity values from a DHT11 sensor. The readTemperature()
and readHumidity()
functions return the values as byte variables, which are then printed to the serial monitor.
Bit Manipulation and Flags
Byte variables are often used for bit manipulation and storing flags. Each bit in a byte can represent a specific condition or state, allowing you to efficiently store and manipulate multiple boolean values within a single byte.
Here’s an example of using a byte variable for storing flags:
byte flags = 0;
#define FLAG_BUTTON_PRESSED 0x01
#define FLAG_SENSOR_ACTIVE 0x02
#define FLAG_ALARM_TRIGGERED 0x04
void setup() {
Serial.begin(9600);
}
void loop() {
// Set flags based on conditions
if (digitalRead(2) == HIGH) {
flags |= FLAG_BUTTON_PRESSED;
}
if (analogRead(A0) > 500) {
flags |= FLAG_SENSOR_ACTIVE;
}
if (flags & (FLAG_BUTTON_PRESSED | FLAG_SENSOR_ACTIVE)) {
flags |= FLAG_ALARM_TRIGGERED;
}
// Check and act on flags
if (flags & FLAG_ALARM_TRIGGERED) {
Serial.println("Alarm triggered!");
}
// Clear flags
flags = 0;
delay(1000);
}
In this example, a byte variable flags
is used to store multiple flags. Each flag is assigned a bit position using bitmasks defined with the #define
directive. The flags are set based on specific conditions using bitwise OR operations. The state of the flags is checked using bitwise AND operations, and appropriate actions are taken based on the flag states. Finally, the flags are cleared by assigning 0 to the flags
variable.
Data Compression and Storage Optimization
When working with limited memory resources, using byte variables can help optimize data storage and reduce memory usage. By storing data as bytes instead of larger data types like integers or floating-point numbers, you can save memory space.
Here’s an example of compressing sensor data using byte variables:
byte compressedData[2];
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue1 = analogRead(A0);
int sensorValue2 = analogRead(A1);
compressedData[0] = sensorValue1 >> 2; // Store upper 8 bits of sensorValue1
compressedData[1] = (sensorValue1 & 0x03) << 6; // Store lower 2 bits of sensorValue1
compressedData[1] |= sensorValue2 >> 4; // Store upper 6 bits of sensorValue2
Serial.print("Compressed Data: ");
Serial.print(compressedData[0]);
Serial.print(", ");
Serial.println(compressedData[1]);
// Decompress the data
int decompressedValue1 = (compressedData[0] << 2) | (compressedData[1] >> 6);
int decompressedValue2 = (compressedData[1] & 0x3F) << 4;
Serial.print("Decompressed Values: ");
Serial.print(decompressedValue1);
Serial.print(", ");
Serial.println(decompressedValue2);
delay(1000);
}
In this example, two 10-bit sensor values are compressed into two bytes. The upper 8 bits of the first sensor value are stored in the first byte, while the lower 2 bits are stored in the upper 2 bits of the second byte. The upper 6 bits of the second sensor value are stored in the remaining bits of the second byte. The compressed data is then printed to the serial monitor. To decompress the data, bitwise operations are used to extract the original sensor values from the compressed bytes.
Frequently Asked Questions (FAQ)
-
What is the range of values that can be stored in a byte variable?
A byte variable can store unsigned integer values ranging from 0 to 255. -
How many bits are in a byte?
A byte consists of 8 bits. -
Can a byte variable store negative numbers?
No, a byte variable is an unsigned data type and can only store non-negative values. If you need to store negative numbers, you can use thechar
data type, which is signed and has a range of -128 to 127. -
What happens if I assign a value greater than 255 to a byte variable?
If you assign a value greater than 255 to a byte variable, the value will overflow and wrap around to a smaller value within the range of 0 to 255. For example, assigning 256 to a byte variable will result in a value of 0. -
Can I use a byte variable to store decimal values?
No, a byte variable can only store integer values. If you need to store decimal values, you can use thefloat
ordouble
data types, which provide floating-point precision.
Conclusion
In this comprehensive guide, we explored the Arduino byte type in detail. We covered the basics of bytes, their declaration and initialization, arithmetic operations, overflow and underflow conditions, byte I/O operations, serial communication, byte arrays and buffers, and practical applications of byte variables.
Understanding the byte type is crucial when working with Arduino, especially when dealing with sensor data, communication protocols, bit manipulation, and memory optimization. By effectively utilizing byte variables, you can create efficient and optimized Arduino programs.
Remember to consider the range and limitations of byte variables and choose the appropriate data type based on your specific requirements. With the knowledge gained from this guide, you are now equipped to tackle byte-related challenges and harness the power of bytes in your Arduino projects.
Happy coding!
Leave a Reply