Introduction to the Wio Terminal
The Wio Terminal is an innovative, feature-packed development board designed for creating IoT projects with ease. Built around the powerful ATSAMD51 microcontroller, this all-in-one Arduino-compatible device offers an impressive array of hardware features, making it an excellent choice for both beginners and experienced makers alike.
Key Features of the Wio Terminal
-
ATSAMD51 Microcontroller: The heart of the Wio Terminal is the ATSAMD51 microcontroller, which provides ample processing power for demanding IoT applications.
-
2.4″ LCD Display: The built-in 2.4″ LCD display allows you to create intuitive user interfaces and visualize data from your projects.
-
Wi-Fi and Bluetooth Connectivity: With integrated Wi-Fi and Bluetooth capabilities, the Wio Terminal enables seamless wireless communication between devices and the cloud.
-
Onboard Sensors: The Wio Terminal comes equipped with an accelerometer, gyroscope, and light sensor, enabling you to capture various environmental data without additional hardware.
-
Expandable with 40-Pin GPIO: The 40-pin GPIO header allows you to connect a wide range of sensors, actuators, and other peripherals to extend the functionality of your projects.
Getting Started with the Wio Terminal
Setting Up the Development Environment
To start developing with the Wio Terminal, you’ll need to set up the Arduino IDE and install the necessary libraries. Follow these steps:
-
Download and install the latest version of the Arduino IDE from the official website: https://www.arduino.cc/en/software
-
Open the Arduino IDE and navigate to File > Preferences.
-
In the “Additional Boards Manager URLs” field, paste the following URL: https://files.seeedstudio.com/arduino/package_seeeduino_boards_index.json
-
Click “OK” to save the preferences.
-
Go to Tools > Board > Boards Manager, search for “Seeed SAMD Boards,” and install the latest version.
-
Select “Seeed Wio Terminal” from the Tools > Board menu.
Uploading Your First Sketch
With the development environment set up, you can now upload your first sketch to the Wio Terminal:
-
Connect the Wio Terminal to your computer using a USB cable.
-
Open the Arduino IDE and select File > Examples > Basic > Blink.
-
Click the “Upload” button to compile and upload the sketch to the Wio Terminal.
-
The onboard LED should start blinking, indicating a successful upload.
Exploring the Wio Terminal’s Capabilities
Creating a Simple IoT Project with the Wio Terminal
Let’s create a simple IoT project that demonstrates the Wio Terminal’s capabilities. In this example, we’ll build a temperature and humidity monitoring system that sends data to the cloud.
Hardware Requirements
- Wio Terminal
- DHT11 or DHT22 temperature and humidity sensor
- Breadboard
- Jumper wires
Software Requirements
- Arduino IDE
- Seeed SAMD Boards library
- DHT library
- PubSubClient library (for MQTT communication)
Step-by-Step Guide
-
Connect the DHT sensor to the Wio Terminal using the breadboard and jumper wires. Connect the sensor’s VCC pin to 3.3V, GND to GND, and the data pin to D0 on the Wio Terminal.
-
Install the required libraries in the Arduino IDE:
- Go to Sketch > Include Library > Manage Libraries.
- Search for “DHT” and install the “DHT sensor library” by Adafruit.
-
Search for “PubSubClient” and install the “PubSubClient” library by Nick O’Leary.
-
Open a new sketch in the Arduino IDE and copy the following code:
#include <DHT.h>
#include <rpcWiFi.h>
#include <PubSubClient.h>
#define DHTPIN D0
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
WiFiClient wioClient;
PubSubClient client(wioClient);
const char* ssid = "your_wifi_ssid";
const char* password = "your_wifi_password";
const char* mqttServer = "your_mqtt_server_address";
const int mqttPort = 1883;
void setup() {
Serial.begin(115200);
dht.begin();
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
client.setServer(mqttServer, mqttPort);
}
void loop() {
if (!client.connected()) {
reconnect();
}
client.loop();
float temperature = dht.readTemperature();
float humidity = dht.readHumidity();
if (isnan(temperature) || isnan(humidity)) {
Serial.println("Failed to read from DHT sensor!");
return;
}
String temperatureStr = String(temperature);
String humidityStr = String(humidity);
client.publish("wio/temperature", temperatureStr.c_str());
client.publish("wio/humidity", humidityStr.c_str());
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print("°C, Humidity: ");
Serial.print(humidity);
Serial.println("%");
delay(5000);
}
void reconnect() {
while (!client.connected()) {
Serial.print("Attempting MQTT connection...");
if (client.connect("WioTerminalClient")) {
Serial.println("connected");
} else {
Serial.print("failed, rc=");
Serial.print(client.state());
Serial.println(" retrying in 5 seconds");
delay(5000);
}
}
}
-
Replace
your_wifi_ssid
,your_wifi_password
, andyour_mqtt_server_address
with your actual Wi-Fi and MQTT server details. -
Upload the sketch to the Wio Terminal.
-
Open the Serial Monitor to view the temperature and humidity readings.
-
Set up an MQTT client (e.g., MQTT.fx or MQTTBox) to subscribe to the
wio/temperature
andwio/humidity
topics to receive the data sent by the Wio Terminal.
This simple project showcases the Wio Terminal’s ability to collect sensor data, connect to Wi-Fi, and send data to the cloud using MQTT.
Visualizing Data with the Wio Terminal’s LCD Display
One of the standout features of the Wio Terminal is its built-in 2.4″ LCD display, which allows you to create intuitive user interfaces and visualize data from your projects. Let’s extend the previous example to display the temperature and humidity readings on the LCD.
- Install the Seeed_Arduino_LCD library in the Arduino IDE:
- Go to Sketch > Include Library > Manage Libraries.
-
Search for “Seeed_Arduino_LCD” and install the library.
-
Modify the code from the previous example to include the following lines:
#include <Seeed_Arduino_LCD.h>
TFT_eSPI tft;
void setup() {
// ...
tft.begin();
tft.setRotation(3);
tft.fillScreen(TFT_BLACK);
tft.setTextColor(TFT_WHITE);
tft.setTextSize(2);
// ...
}
void loop() {
// ...
tft.fillScreen(TFT_BLACK);
tft.setCursor(0, 0);
tft.print("Temperature: ");
tft.print(temperature);
tft.println(" C");
tft.print("Humidity: ");
tft.print(humidity);
tft.println("%");
// ...
}
- Upload the modified sketch to the Wio Terminal.
The LCD display will now show the current temperature and humidity readings, updating every 5 seconds.
Expanding Functionality with the 40-Pin GPIO
The Wio Terminal’s 40-pin GPIO header allows you to connect a wide range of sensors, actuators, and other peripherals to extend the functionality of your projects. Some popular examples include:
- PIR motion sensors
- Ultrasonic distance sensors
- Relay modules for controlling high-power devices
- RFID readers for access control systems
- Servo motors for robotics applications
To use these peripherals, simply connect them to the appropriate pins on the GPIO header and install the necessary libraries in the Arduino IDE.
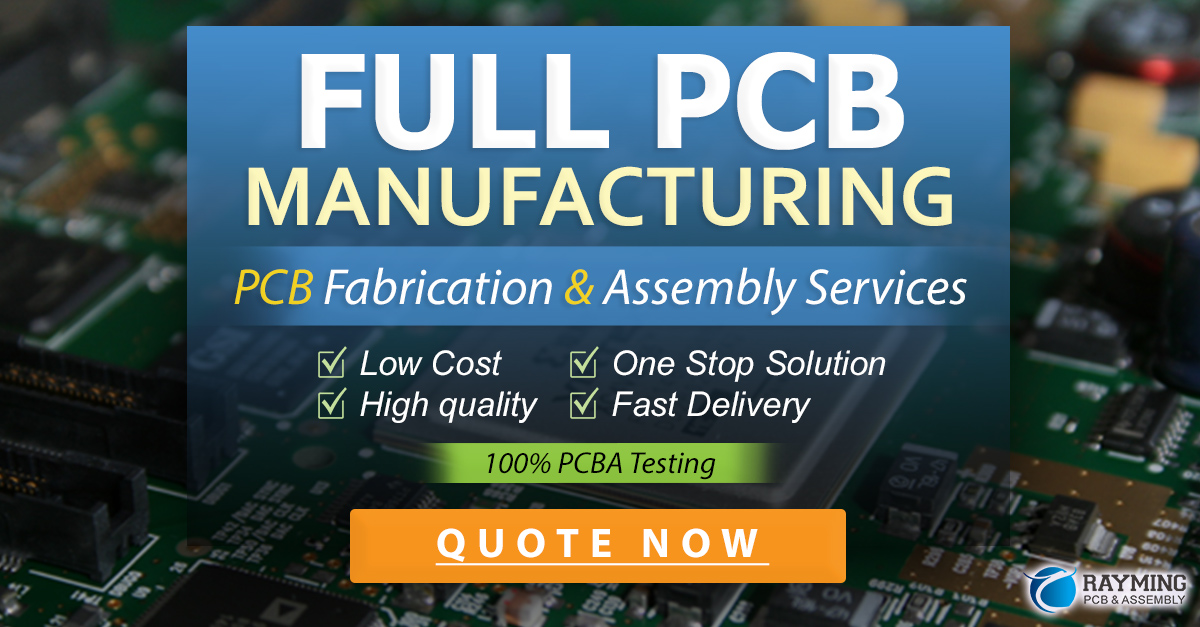
Wio Terminal and the IoT Ecosystem
The Wio Terminal is an excellent choice for IoT projects, thanks to its built-in Wi-Fi and Bluetooth capabilities. It seamlessly integrates with popular IoT platforms and protocols, such as:
- MQTT (Message Queuing Telemetry Transport)
- CoAP (Constrained Application Protocol)
- AWS IoT
- Microsoft Azure IoT Hub
- Google Cloud IoT Core
By leveraging these platforms and protocols, you can create powerful IoT applications that collect, process, and analyze data from multiple devices, enabling real-time monitoring, control, and automation.
Troubleshooting Common Issues
1. Uploading Sketches
If you encounter problems uploading sketches to the Wio Terminal, try the following:
- Ensure that you have selected the correct board (Seeed Wio Terminal) and port in the Arduino IDE.
- Press and hold the boot button on the Wio Terminal while uploading the sketch.
- Check that the USB cable is securely connected to both the Wio Terminal and your computer.
2. Wi-Fi Connectivity
If your Wio Terminal is unable to connect to Wi-Fi, consider these troubleshooting steps:
- Double-check that you have entered the correct Wi-Fi SSID and password in your sketch.
- Ensure that your Wi-Fi network is within range and functioning properly.
- Verify that the Wio Terminal’s antenna is securely connected.
3. Sensor Readings
If you’re not getting the expected readings from sensors connected to the Wio Terminal, try the following:
- Check that the sensor is properly connected to the correct pins on the Wio Terminal.
- Ensure that you have installed the necessary libraries for the sensor in the Arduino IDE.
- Verify that the sensor is functioning correctly by testing it with a multimeter or a known working setup.
FAQ
-
Q: Can I use the Wio Terminal with programming languages other than Arduino?
A: Yes, the Wio Terminal is compatible with other programming languages and platforms, such as MicroPython and CircuitPython. -
Q: How do I update the firmware on the Wio Terminal?
A: You can update the firmware using the Seeed_ArduinoCore_Wio repository on GitHub. Follow the instructions provided in the repository’s README file. -
Q: Is the Wio Terminal suitable for battery-powered projects?
A: Yes, the Wio Terminal can be powered by a 3.7V lithium battery connected to the onboard JST connector. It also has built-in charging circuitry for easy battery management. -
Q: Can I connect multiple Wio Terminals together?
A: Yes, you can connect multiple Wio Terminals using various communication protocols, such as I2C, SPI, or UART. You can also use Wi-Fi or Bluetooth for wireless communication between devices. -
Q: Where can I find more resources and project ideas for the Wio Terminal?
A: You can find a wealth of resources, tutorials, and project ideas on the Seeed Studio Wiki, the official Arduino website, and various online maker communities, such as Hackster.io and Instructables.
Conclusion
The Wio Terminal is a powerful, all-in-one Arduino-compatible development board that offers an impressive array of features for creating IoT projects. With its built-in sensors, wireless connectivity, and expandable GPIO, the Wio Terminal is an excellent choice for both beginners and experienced makers alike.
By following the guides and examples provided in this article, you can quickly get started with the Wio Terminal and begin exploring its capabilities. Whether you’re interested in environmental monitoring, home automation, or robotics, the Wio Terminal provides a solid foundation for bringing your IoT ideas to life.
As you continue to develop your skills and knowledge, be sure to take advantage of the many resources available online, including tutorials, forums, and maker communities. With the Wio Terminal and the support of the Arduino and IoT ecosystem, the possibilities for creating innovative and impactful projects are endless.
Leave a Reply