Introduction to SAMD21 Microcontroller
The SAMD21 is a powerful and versatile microcontroller that has been gaining popularity in the Arduino community. This 32-bit ARM Cortex-M0+ based microcontroller offers a wide range of features and capabilities that make it an excellent choice for a variety of projects, from simple IoT devices to complex embedded systems.
Key Features of SAMD21 Microcontroller
- 32-bit ARM Cortex-M0+ core
- Up to 256KB of Flash memory and 32KB of SRAM
- Operating voltage range from 1.62V to 3.63V
- Up to 48MHz clock speed
- Multiple serial communication interfaces (UART, I2C, SPI)
- 12-bit Analog-to-Digital Converter (ADC)
- 10-bit Digital-to-Analog Converter (DAC)
- Pulse Width Modulation (PWM) support
- Real-Time Counter (RTC) with clock/calendar function
- Watchdog Timer (WDT) for system reliability
- Peripheral Touch Controller (PTC) for capacitive touch sensing
These features make the SAMD21 a highly capable microcontroller that can handle a wide range of tasks and applications.
Comparison with other Arduino-compatible Microcontrollers
The SAMD21 is a significant step up from the more traditional Arduino boards, such as the Arduino Uno or Nano, which are based on the 8-bit ATmega328P microcontroller. The following table compares some key specifications of the SAMD21 with the ATmega328P:
Feature | SAMD21 | ATmega328P |
---|---|---|
Architecture | 32-bit ARM Cortex-M0+ | 8-bit AVR |
Clock Speed | Up to 48MHz | 16MHz |
Flash Memory | Up to 256KB | 32KB |
SRAM | Up to 32KB | 2KB |
ADC Resolution | 12-bit | 10-bit |
DAC Resolution | 10-bit | Not available |
Operating Voltage | 1.62V to 3.63V | 1.8V to 5.5V |
As evident from the table, the SAMD21 offers significant improvements in terms of processing power, memory, and peripheral capabilities compared to the ATmega328P.
Advantages of SAMD21 over ATmega328P
- Higher processing speed: The SAMD21’s 48MHz clock speed allows for faster execution of complex algorithms and tasks.
- Larger memory: With up to 256KB of Flash and 32KB of SRAM, the SAMD21 can store and manipulate larger amounts of data.
- Enhanced peripherals: The SAMD21 features higher resolution ADC and DAC, as well as additional peripherals like the PTC for capacitive touch sensing.
- Lower power consumption: The SAMD21’s lower operating voltage range and advanced power management features enable more efficient power usage.
Getting Started with SAMD21 Development
To start developing with the SAMD21 microcontroller, you’ll need the following:
- A development board with the SAMD21 microcontroller (e.g., Arduino MKR Zero, Adafruit Feather M0, or Seeed Studio XIAO)
- A computer with the Arduino IDE installed
- A USB cable to connect the development board to your computer
Setting up the Arduino IDE for SAMD21
- Open the Arduino IDE and navigate to “Tools” > “Board” > “Boards Manager”
- Search for “SAMD” in the Boards Manager and install the “Arduino SAMD Boards (32-bits ARM Cortex-M0+)” package
- Select your SAMD21 development board from the “Tools” > “Board” menu
- Choose the appropriate port from the “Tools” > “Port” menu
Your First SAMD21 Sketch: Blinking an LED
To test your setup, let’s create a simple sketch that blinks an LED connected to the SAMD21 board:
void setup() {
pinMode(LED_BUILTIN, OUTPUT);
}
void loop() {
digitalWrite(LED_BUILTIN, HIGH);
delay(1000);
digitalWrite(LED_BUILTIN, LOW);
delay(1000);
}
This sketch sets the built-in LED pin as an output and alternately turns it on and off with a 1-second delay. Upload the sketch to your SAMD21 board and observe the blinking LED.
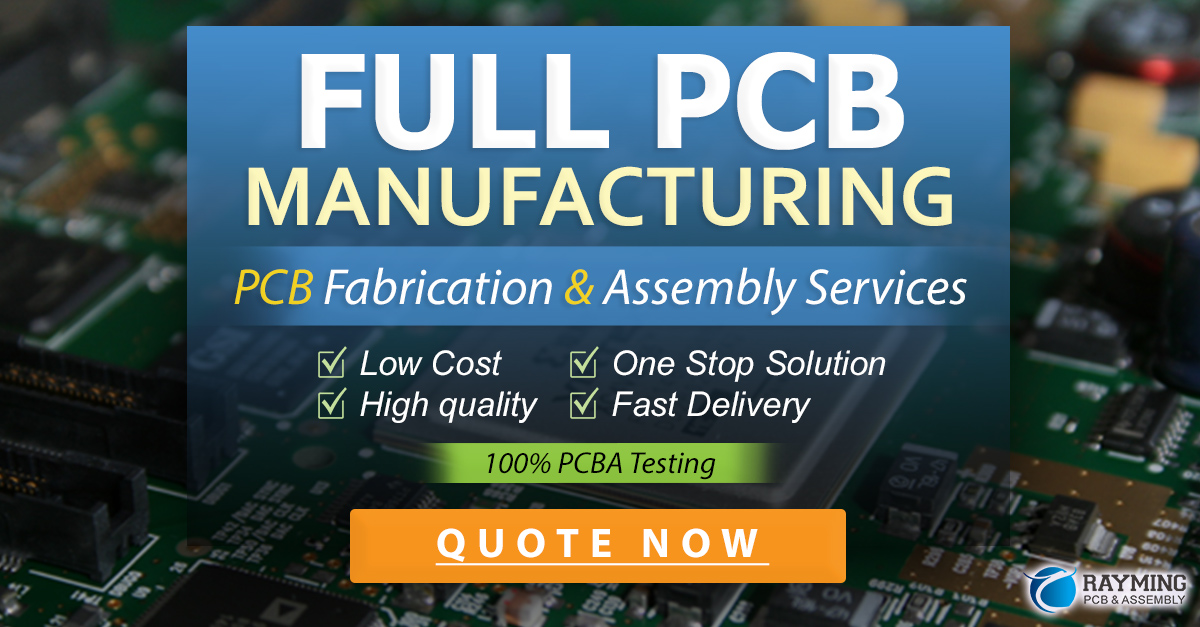
Exploring SAMD21 Peripherals
The SAMD21 microcontroller offers a wide range of peripherals that enable various functionalities. Let’s explore some of the most commonly used peripherals and their applications.
Analog-to-Digital Converter (ADC)
The SAMD21’s 12-bit ADC allows for precise measurement of analog signals, such as sensor readings or voltage levels. To use the ADC, follow these steps:
- Connect the analog signal to one of the SAMD21’s analog input pins (A0-A5)
- Use the
analogRead()
function to read the analog value - Convert the analog value to the corresponding voltage or physical quantity
Example sketch for reading an analog sensor:
const int sensorPin = A0;
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(sensorPin);
float voltage = sensorValue * (3.3 / 4095.0);
Serial.print("Sensor Value: ");
Serial.print(sensorValue);
Serial.print(", Voltage: ");
Serial.println(voltage);
delay(1000);
}
This sketch reads the analog value from the sensor connected to pin A0, converts it to voltage (assuming a 3.3V reference voltage), and prints the values to the serial monitor.
Pulse Width Modulation (PWM)
PWM is a technique used to generate analog-like signals using digital pins. The SAMD21 supports PWM on multiple pins, allowing for control of LED brightness, motor speed, or servo position. To use PWM:
- Connect the device to one of the SAMD21’s PWM-capable pins
- Use the
analogWrite()
function to set the PWM duty cycle - Adjust the duty cycle to control the connected device
Example sketch for controlling LED brightness:
const int ledPin = 3;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
for (int brightness = 0; brightness <= 255; brightness++) {
analogWrite(ledPin, brightness);
delay(10);
}
for (int brightness = 255; brightness >= 0; brightness--) {
analogWrite(ledPin, brightness);
delay(10);
}
}
This sketch gradually increases and decreases the brightness of an LED connected to PWM pin 3 using the analogWrite()
function.
Inter-Integrated Circuit (I2C) Communication
I2C is a popular serial communication protocol used for connecting various devices, such as sensors, displays, or memory modules, to the microcontroller. The SAMD21 has built-in I2C support, making it easy to communicate with I2C devices. To use I2C:
- Connect the I2C device to the SAMD21’s SDA and SCL pins
- Use the Wire library to initialize the I2C communication
- Communicate with the device using the Wire library’s functions
Example sketch for reading data from an I2C temperature sensor:
#include <Wire.h>
const int sensorAddress = 0x48;
void setup() {
Wire.begin();
Serial.begin(9600);
}
void loop() {
Wire.beginTransmission(sensorAddress);
Wire.write(0x00);
Wire.endTransmission();
Wire.requestFrom(sensorAddress, 2);
float temperature = (Wire.read() << 8 | Wire.read()) / 256.0;
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(" °C");
delay(1000);
}
This sketch communicates with an I2C temperature sensor at address 0x48, reads the temperature data, and prints the value to the serial monitor.
Advanced SAMD21 Features and Applications
The SAMD21 microcontroller offers several advanced features that enable more complex applications and projects. Let’s explore a few of these features and their potential applications.
Real-Time Counter (RTC) and Low-Power Operation
The SAMD21 features a built-in RTC that allows for accurate timekeeping and clock/calendar functionality. The RTC can be used in conjunction with the microcontroller’s low-power modes to create energy-efficient applications, such as battery-powered sensors or wearable devices.
To use the RTC and low-power modes:
- Configure the RTC using the RTCZero library
- Set up the desired low-power mode (Idle, Standby, or Backup)
- Use interrupts or the RTC alarm to wake the microcontroller from low-power mode
Example sketch for using the RTC and low-power mode:
#include <RTCZero.h>
RTCZero rtc;
void setup() {
rtc.begin();
rtc.setTime(0, 0, 0);
rtc.setDate(1, 1, 2021);
rtc.attachInterrupt(alarmMatch);
rtc.setAlarmTime(0, 0, 10);
rtc.enableAlarm(rtc.MATCH_SS);
}
void loop() {
// Enter idle mode
SCB->SCR &= ~SCB_SCR_SLEEPDEEP_Msk;
__DSB();
__WFI();
}
void alarmMatch() {
// Do something when the alarm triggers
// For example, read a sensor and send data over a wireless connection
}
This sketch sets up the RTC, configures an alarm to trigger every 10 seconds, and enters idle mode. When the alarm triggers, the alarmMatch()
function is called, where you can perform tasks such as reading sensors or sending data.
Peripheral Touch Controller (PTC) and Capacitive Touch Sensing
The SAMD21’s PTC enables capacitive touch sensing, allowing for the creation of touch-based user interfaces or proximity sensors. To use the PTC:
- Configure the PTC using the Arduino_PTC library
- Define the touch sensor pins and thresholds
- Read the touch sensor states and perform actions based on the touch events
Example sketch for creating a capacitive touch button:
#include <Arduino_PTC.h>
const int touchPin = A0;
const int ledPin = 3;
const int touchThreshold = 500;
void setup() {
PTC.begin();
PTC.attachInterrupt(touchPin, touchInterrupt);
pinMode(ledPin, OUTPUT);
}
void loop() {
// The touch interrupt will handle the touch events
}
void touchInterrupt() {
int touchValue = PTC.read(touchPin);
if (touchValue > touchThreshold) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
}
This sketch sets up a capacitive touch sensor on pin A0 and a LED on pin 3. When a touch is detected (i.e., the touch value exceeds the threshold), the LED is turned on. When the touch is released, the LED turns off.
Frequently Asked Questions (FAQ)
-
Can I use the SAMD21 with the regular Arduino IDE?
Yes, you can use the SAMD21 with the Arduino IDE by installing the “Arduino SAMD Boards (32-bits ARM Cortex-M0+)” package through the Boards Manager. -
Is the SAMD21 compatible with existing Arduino libraries?
Most Arduino libraries should work with the SAMD21, but some may require modifications or adaptations due to the differences in architecture and peripherals. -
How does the SAMD21’s performance compare to other Arduino-compatible boards?
The SAMD21 offers significantly better performance than 8-bit Arduino boards like the Uno or Nano, with a faster clock speed, larger memory, and more advanced peripherals. -
What are some popular development boards that feature the SAMD21 microcontroller?
Some popular SAMD21-based development boards include the Arduino MKR Zero, Adafruit Feather M0, and Seeed Studio XIAO. -
Can I use the SAMD21 for battery-powered projects?
Yes, the SAMD21’s low-power modes and RTC make it well-suited for battery-powered projects, as it can enter sleep modes to conserve energy and wake up periodically to perform tasks.
Conclusion
The SAMD21 microcontroller is a powerful and versatile choice for Arduino enthusiasts looking to step up their projects’ capabilities. With its 32-bit ARM Cortex-M0+ core, extensive memory, and advanced peripherals, the SAMD21 enables the creation of more complex and feature-rich applications.
By exploring the SAMD21’s ADC, PWM, I2C, RTC, and PTC features, you can create a wide range of projects, from sensor-based systems to low-power wearables and touch-based interfaces. The Arduino IDE and the vast ecosystem of libraries and resources make it easy to get started with SAMD21 development.
As you continue to work with the SAMD21, you’ll discover even more possibilities and applications for this powerful microcontroller. With its performance, flexibility, and growing community support, the SAMD21 is an excellent choice for taking your Arduino projects to the next level.
Leave a Reply