What is the Arduino Serial Monitor?
The Arduino Serial Monitor is a built-in feature of the Arduino IDE (Integrated Development Environment) that allows you to communicate with your Arduino board using a serial connection. It provides a way to send and receive data between your computer and the Arduino board, making it an essential tool for debugging, testing, and monitoring your Arduino projects.
Key Features of the Arduino Serial Monitor
- Sending data from the computer to the Arduino
- Receiving data from the Arduino to the computer
- Displaying text messages and sensor readings
- Debugging and troubleshooting Arduino sketches
- Monitoring real-time data from connected sensors or modules
Setting Up the Arduino Serial Monitor
To use the Arduino Serial Monitor, you need to have the Arduino IDE installed on your computer. Follow these steps to set up the Serial Monitor:
- Connect your Arduino board to your computer using a USB cable
- Open the Arduino IDE
- Select the appropriate board and port from the “Tools” menu
- Open or create an Arduino sketch that includes serial communication
- Click on the Serial Monitor icon in the top-right corner of the IDE or go to “Tools” > “Serial Monitor”
Arduino Sketch Example for Serial Communication
Here’s a simple Arduino sketch that demonstrates serial communication:
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 baud rate
}
void loop() {
Serial.println("Hello, Arduino Serial Monitor!"); // Send a message to the Serial Monitor
delay(1000); // Wait for 1 second before sending the next message
}
In this example, the Serial.begin(9600)
function initializes the serial communication at a baud rate of 9600 bits per second. The Serial.println()
function sends the message “Hello, Arduino Serial Monitor!” to the Serial Monitor. The delay(1000)
function introduces a 1-second delay between each message.
Using the Arduino Serial Monitor
Sending Data from the Computer to the Arduino
To send data from your computer to the Arduino using the Serial Monitor, follow these steps:
- Make sure your Arduino sketch includes code to read incoming serial data, such as
Serial.read()
orSerial.readString()
- Open the Serial Monitor in the Arduino IDE
- Type your message or command in the input field at the top of the Serial Monitor
- Press the “Send” button or hit the Enter key to send the data to the Arduino
Receiving Data from the Arduino to the Computer
To receive data from the Arduino and display it in the Serial Monitor, follow these steps:
- Make sure your Arduino sketch includes code to send data over serial, such as
Serial.print()
orSerial.println()
- Open the Serial Monitor in the Arduino IDE
- The data sent by the Arduino will appear in the Serial Monitor display area
Configuring the Serial Monitor
The Serial Monitor offers several configuration options to customize its behavior:
- Baud Rate: Select the appropriate baud rate from the dropdown menu to match the baud rate used in your Arduino sketch (e.g., 9600, 115200)
- Line Ending: Choose the line ending format for the serial data (e.g., No line ending, Newline, Carriage return, Both NL & CR)
- Autoscroll: Enable or disable autoscrolling of the Serial Monitor display
- Show timestamp: Display a timestamp for each received message
Setting | Description | Options |
---|---|---|
Baud Rate | The speed of serial communication | 9600, 115200, etc. |
Line Ending | The format for line endings in serial data | No line ending, Newline, Carriage return, Both NL & CR |
Autoscroll | Automatically scroll the display as new data arrives | Enabled, Disabled |
Show timestamp | Display a timestamp for each received message | Enabled, Disabled |
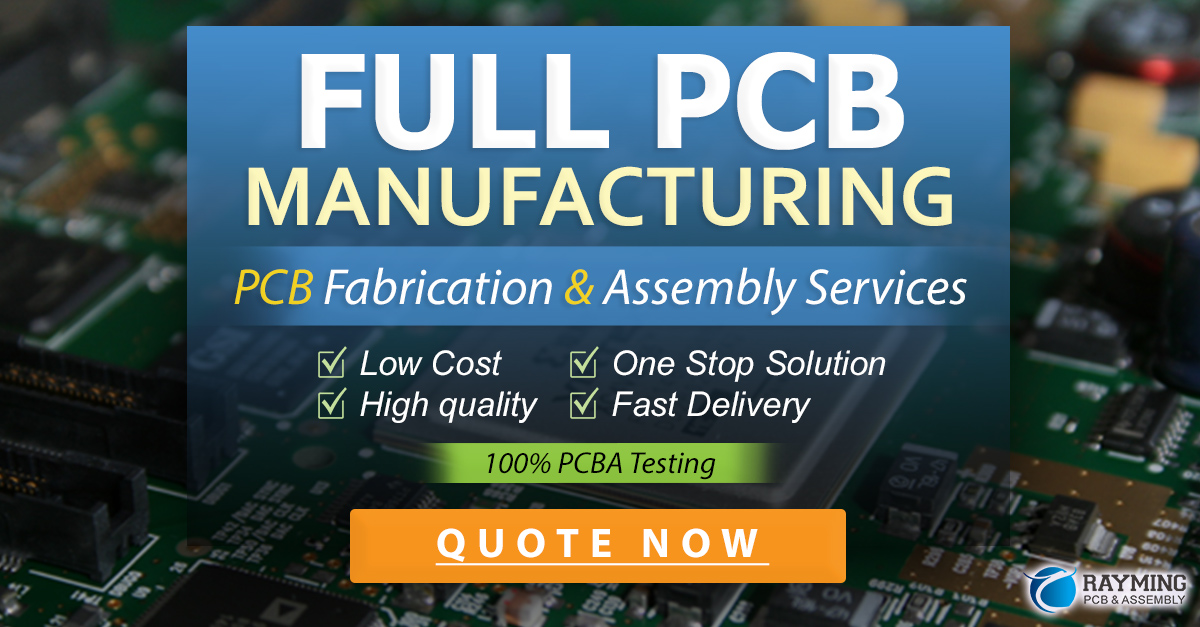
Debugging with the Arduino Serial Monitor
The Arduino Serial Monitor is a powerful tool for debugging your Arduino sketches. By strategically placing Serial.print()
or Serial.println()
statements in your code, you can track the flow of your program, check variable values, and identify issues.
Debugging Techniques
- Print variable values: Use
Serial.print()
orSerial.println()
to display the values of variables at specific points in your code - Print status messages: Use serial communication to print status messages indicating the current state of your program or the occurrence of specific events
- Print sensor readings: Display sensor readings in the Serial Monitor to verify that your sensors are working correctly and providing accurate data
- Print loop iterations: Print a message or a counter value in each iteration of a loop to ensure that the loop is running as expected
- Print function calls: Print messages when entering or exiting functions to trace the flow of your program
Example: Debugging a Temperature Sensor
Let’s consider an example where you’re using a temperature sensor (e.g., DHT11) with your Arduino, and you want to debug the sensor readings. Here’s how you can use the Serial Monitor for debugging:
#include <DHT.h>
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
delay(2000); // Wait for 2 seconds between readings
float temperature = dht.readTemperature();
if (isnan(temperature)) {
Serial.println("Failed to read from DHT sensor!");
} else {
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(" °C");
}
}
In this example, the Serial.print()
and Serial.println()
statements are used to display the temperature readings in the Serial Monitor. If the sensor fails to provide a reading, the message “Failed to read from DHT sensor!” will be printed, indicating an issue with the sensor or the connection.
Advanced Serial Monitor Techniques
Plotting Data with the Serial Plotter
In addition to the Serial Monitor, the Arduino IDE also includes a Serial Plotter tool. The Serial Plotter allows you to visualize data sent from the Arduino as graphs in real-time. To use the Serial Plotter:
- Ensure that your Arduino sketch sends numeric data over serial, separated by commas or spaces
- Open the Serial Plotter by going to “Tools” > “Serial Plotter”
- The Serial Plotter will display the received data as graphs, with each data series represented by a different color
Sending Formatted Data
To send formatted data from the Arduino to the Serial Monitor, you can use the Serial.print()
function with format specifiers. Format specifiers allow you to control the representation of different data types. Here are some commonly used format specifiers:
Format Specifier | Description | Example |
---|---|---|
%d | Integer | Serial.print(“Value: %d”, 42); |
%f | Floating-point number | Serial.print(“Temperature: %f”, 25.5); |
%s | String | Serial.print(“Message: %s”, “Hello”); |
%x | Hexadecimal integer | Serial.print(“Hex: %x”, 255); |
Sending Binary Data
In addition to text-based data, you can also send binary data over serial using the Arduino Serial Monitor. To send binary data:
- Use the
Serial.write()
function to send individual bytes or byte arrays - On the receiving end, use the
Serial.read()
function to read the incoming binary data
Example:
void setup() {
Serial.begin(9600);
}
void loop() {
byte data[] = {0x48, 0x65, 0x6C, 0x6C, 0x6F}; // "Hello" in ASCII
Serial.write(data, sizeof(data));
delay(1000);
}
Frequently Asked Questions (FAQ)
-
What is the purpose of the Arduino Serial Monitor?
The Arduino Serial Monitor is a tool that allows you to communicate with your Arduino board using a serial connection. It is used for sending and receiving data, debugging, and monitoring Arduino projects. -
How do I open the Arduino Serial Monitor?
To open the Arduino Serial Monitor, click on the Serial Monitor icon in the top-right corner of the Arduino IDE or go to “Tools” > “Serial Monitor”. -
What baud rate should I use for serial communication?
The baud rate depends on your Arduino sketch. Make sure to set the same baud rate in your sketch using theSerial.begin()
function and in the Serial Monitor’s baud rate dropdown menu. Common baud rates are 9600 and 115200. -
How can I send data from the computer to the Arduino using the Serial Monitor?
To send data from the computer to the Arduino, make sure your sketch includes code to read incoming serial data (e.g.,Serial.read()
orSerial.readString()
). Then, type your message or command in the input field at the top of the Serial Monitor and press the “Send” button or hit the Enter key. -
How can I debug my Arduino sketch using the Serial Monitor?
You can debug your Arduino sketch by addingSerial.print()
orSerial.println()
statements at strategic points in your code. These statements allow you to print variable values, status messages, sensor readings, or function calls to the Serial Monitor, helping you track the flow of your program and identify issues.
Conclusion
The Arduino Serial Monitor is an indispensable tool for any Arduino enthusiast or developer. It provides a simple and effective way to communicate with your Arduino board, send and receive data, debug sketches, and monitor real-time information from sensors and modules.
By mastering the use of the Arduino Serial Monitor, you can greatly enhance your Arduino programming experience, streamline your development process, and create more robust and reliable projects.
Remember to experiment with different Serial Monitor techniques, such as plotting data, sending formatted data, and transmitting binary data, to expand your Arduino skillset and tackle more complex projects.
Happy Arduino programming, and may the Serial Monitor be your faithful companion on your Arduino journey!
Leave a Reply