Introduction to Arduino Breadboard
An Arduino breadboard is an essential tool for anyone interested in electronics and prototyping. It is a simple, yet powerful, device that allows you to quickly and easily create circuits and test out ideas. In this article, we will explore the basics of Arduino breadboards, including what they are, how they work, and why they are so useful for prototyping.
What is an Arduino Breadboard?
An Arduino breadboard is a simple, reusable prototyping board that allows you to quickly and easily create circuits without the need for soldering. It consists of a grid of holes that are connected by metal strips underneath the surface. These metal strips allow you to connect components together to create a circuit.
The Arduino breadboard is specifically designed to work with the Arduino microcontroller, which is an open-source platform for building electronics projects. The Arduino microcontroller can be easily connected to the breadboard, allowing you to create and test circuits quickly and easily.
Why Use an Arduino Breadboard?
There are many reasons why you might want to use an Arduino breadboard for your electronics projects. Some of the main benefits include:
-
Quick and Easy Prototyping: With an Arduino breadboard, you can quickly and easily create circuits without the need for soldering. This makes it easy to test out ideas and make changes on the fly.
-
Reusable: Unlike other prototyping methods, such as perfboard or stripboard, an Arduino breadboard is completely reusable. Once you’re done with a project, you can simply remove the components and start fresh with a new circuit.
-
Cost-Effective: Arduino breadboards are relatively inexpensive, making them a great option for hobbyists and students who are just getting started with electronics.
-
Compatible with Arduino: The Arduino breadboard is specifically designed to work with the Arduino microcontroller, making it easy to integrate into your projects.
How to Use an Arduino Breadboard
Using an Arduino breadboard is relatively simple, but it does require some basic knowledge of electronics and circuit design. In this section, we’ll walk through the basics of using an Arduino breadboard.
Understanding the Layout
The first step in using an Arduino breadboard is to understand the layout. A typical breadboard consists of two main sections: the power rails and the terminal strips.
The power rails are the two long rows of holes that run along the top and bottom of the breadboard. These are typically marked with a red line (+) and a blue line (-), indicating the positive and negative power supply, respectively.
The terminal strips are the main area of the breadboard where you will place your components. Each terminal strip consists of five holes that are electrically connected by a metal strip underneath the surface. The metal strips run horizontally across the breadboard, allowing you to connect components in rows.
Connecting Components
To connect components on an Arduino breadboard, you simply need to insert the leads of the component into the appropriate holes on the terminal strips. For example, if you wanted to connect an LED to a resistor, you would insert the positive lead of the LED into one hole on a terminal strip, and the negative lead of the LED into another hole on the same terminal strip. You would then insert one lead of the resistor into the same hole as the negative lead of the LED, and the other lead of the resistor into a different hole on the same terminal strip.
It’s important to note that the power rails are not connected to the terminal strips by default. If you want to supply power to your circuit, you will need to use jumper wires to connect the power rails to the appropriate holes on the terminal strips.
Using the Arduino Microcontroller
One of the main benefits of using an Arduino breadboard is that it is specifically designed to work with the Arduino microcontroller. To use the Arduino with your breadboard, you will need to connect it to your computer using a USB cable.
Once the Arduino is connected, you can use the Arduino IDE (Integrated Development Environment) to write and upload code to the microcontroller. The Arduino IDE is a free, open-source software that allows you to write code in a simplified version of C++.
To connect the Arduino to your breadboard, you will need to use jumper wires to connect the appropriate pins on the Arduino to the appropriate holes on the breadboard. The specific connections will depend on the circuit you are building, but a typical setup might look something like this:
Arduino Pin | Breadboard Connection |
---|---|
5V | Power Rail (+) |
GND | Power Rail (-) |
Digital Pin 2 | LED Positive Lead |
Digital Pin 3 | Button One Lead |
Digital Pin 4 | Button Other Lead |
Example Projects
Now that you have a basic understanding of how to use an Arduino breadboard, let’s take a look at some example projects that you can build.
Project 1: Blinking LED
One of the most basic projects you can build with an Arduino breadboard is a blinking LED. This project is a great way to get started with the Arduino and learn the basics of programming and circuit design.
To build this project, you will need:
- Arduino Uno
- Breadboard
- LED
- 220 ohm resistor
- Jumper wires
Follow these steps to build the circuit:
- Connect the 5V pin on the Arduino to the positive power rail on the breadboard.
- Connect the GND pin on the Arduino to the negative power rail on the breadboard.
- Insert the LED into the breadboard, with the positive lead (the longer one) connected to a terminal strip.
- Insert the resistor into the breadboard, with one lead connected to the same terminal strip as the negative lead of the LED, and the other lead connected to a different terminal strip.
- Use a jumper wire to connect the terminal strip with the resistor to the GND power rail.
- Use another jumper wire to connect the terminal strip with the positive lead of the LED to digital pin 13 on the Arduino.
Once you have built the circuit, you can use the Arduino IDE to write and upload the following code:
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
This code will cause the LED to blink on and off every second.
Project 2: Button-Controlled LED
Another simple project you can build with an Arduino breadboard is a button-controlled LED. This project builds on the blinking LED project by adding a button that allows you to control when the LED turns on and off.
To build this project, you will need:
- Arduino Uno
- Breadboard
- LED
- 220 ohm resistor
- Pushbutton
- 10k ohm resistor
- Jumper wires
Follow these steps to build the circuit:
- Follow steps 1-5 from the blinking LED project to set up the LED and resistor on the breadboard.
- Insert the pushbutton into the breadboard, with one lead connected to a terminal strip and the other lead connected to a different terminal strip.
- Use a jumper wire to connect the terminal strip with one lead of the pushbutton to digital pin 2 on the Arduino.
- Insert the 10k ohm resistor into the breadboard, with one lead connected to the same terminal strip as the other lead of the pushbutton, and the other lead connected to the GND power rail.
Once you have built the circuit, you can use the Arduino IDE to write and upload the following code:
const int buttonPin = 2;
const int ledPin = 13;
int buttonState = 0;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(ledPin, OUTPUT);
}
void loop() {
buttonState = digitalRead(buttonPin);
if (buttonState == HIGH) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
}
This code will cause the LED to turn on when the button is pressed, and turn off when the button is released.
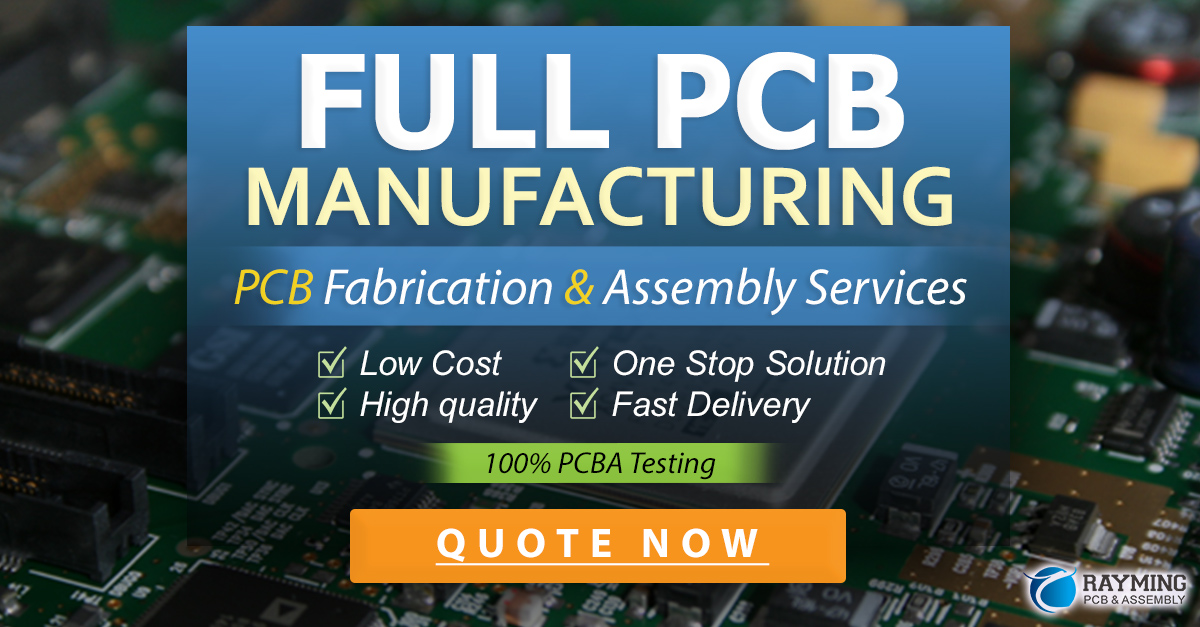
Frequently Asked Questions
What is the difference between a breadboard and a perfboard?
A breadboard is a reusable prototyping board that allows you to quickly and easily create circuits without the need for soldering. A perfboard, on the other hand, is a permanent prototyping board that requires soldering to connect components.
Can I use any type of component with an Arduino breadboard?
Yes, you can use any type of component with an Arduino breadboard, as long as it is compatible with the voltage and current requirements of the Arduino. However, some components, such as motors and relays, may require additional circuitry to work properly.
How many components can I connect to an Arduino breadboard?
The number of components you can connect to an Arduino breadboard depends on the size of the breadboard and the complexity of your circuit. A typical half-size breadboard has around 400 tie points, which should be enough for most simple projects.
Can I use an Arduino breadboard with other microcontrollers?
While the Arduino breadboard is specifically designed to work with the Arduino microcontroller, you can use it with other microcontrollers as well. However, you may need to make some modifications to your circuit and code to accommodate the differences between the microcontrollers.
How do I know if my circuit is working correctly on the breadboard?
The best way to test if your circuit is working correctly on the breadboard is to use a multimeter to measure the voltage and current at various points in the circuit. You can also use the serial monitor in the Arduino IDE to print out debugging information and check the status of your components.
Conclusion
Arduino breadboards are an essential tool for anyone interested in electronics and prototyping. They allow you to quickly and easily create circuits and test out ideas without the need for soldering. By understanding the basics of how to use an Arduino breadboard, you can start building your own projects and exploring the world of electronics.
Remember to start with simple projects, like the blinking LED and button-controlled LED, and work your way up to more complex circuits as you gain experience. With a little practice and patience, you’ll be creating your own custom electronics projects in no time!
Leave a Reply