Introduction to OpenMV Cam
OpenMV Cam is a powerful, low-cost, and easy-to-use smart vision camera designed for makers, hobbyists, and professionals alike. It combines the power of machine vision with the flexibility of Python programming, making it an ideal tool for a wide range of applications, from robotics and automation to security and surveillance.
Key Features of OpenMV Cam
Feature | Description |
---|---|
Powerful Processor | ARM Cortex-M7 processor running at 216 MHz |
High-Resolution Camera | Omnivision OV7725 image sensor with 640×480 resolution |
Python Programming | Supports Python scripting for easy customization |
Compact Size | Measures just 45mm x 36mm x 30mm |
Low Power Consumption | Consumes less than 140mA at 3.3V |
Getting Started with OpenMV Cam
Hardware Setup
- Connect the OpenMV Cam to your computer using a micro-USB cable.
- Install the OpenMV IDE from the official website: https://openmv.io/pages/download
- Launch the OpenMV IDE and select the appropriate port for your camera.
Writing Your First Script
- In the OpenMV IDE, create a new file and name it
hello_world.py
. - Type the following code into the file:
import sensor, image, time
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
clock = time.clock()
while(True):
clock.tick()
img = sensor.snapshot()
print(clock.fps())
- Save the file and click the “Run” button to execute the script.
Exploring OpenMV Cam’s Capabilities
Image Processing
OpenMV Cam offers a wide range of image processing functions, including:
- Color tracking
- Face detection
- QR code and barcode reading
- AprilTag detection
- Blob detection
Here’s an example of color tracking using OpenMV Cam:
import sensor, image, time
red_threshold = (30, 100, 15, 127, 15, 127) # Modify these values to suit your needs
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
while(True):
img = sensor.snapshot()
for blob in img.find_blobs([red_threshold], pixels_threshold=200, area_threshold=200, merge=True):
img.draw_rectangle(blob.rect())
img.draw_cross(blob.cx(), blob.cy())
Machine Learning
OpenMV Cam also supports machine learning, allowing you to train and deploy models directly on the camera. Some of the supported machine learning algorithms include:
- K-Nearest Neighbors (KNN)
- Support Vector Machines (SVM)
- Decision Trees
- Haar Cascades
Here’s an example of using KNN for image classification:
import sensor, image, time, os, machine_vision
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
knn = machine_vision.KNN(k=3, distance=machine_vision.METRIC_EUCLIDEAN)
# Load training data
for i in range(1, 4):
for j in range(1, 6):
img = image.Image("training_data/%s/%s.png" % (i, j))
knn.add_example(img.histogram(), i)
# Classify new images
while(True):
img = sensor.snapshot()
class_id = knn.classify(img.histogram())
print("Predicted class: %s" % class_id)
time.sleep(1000)
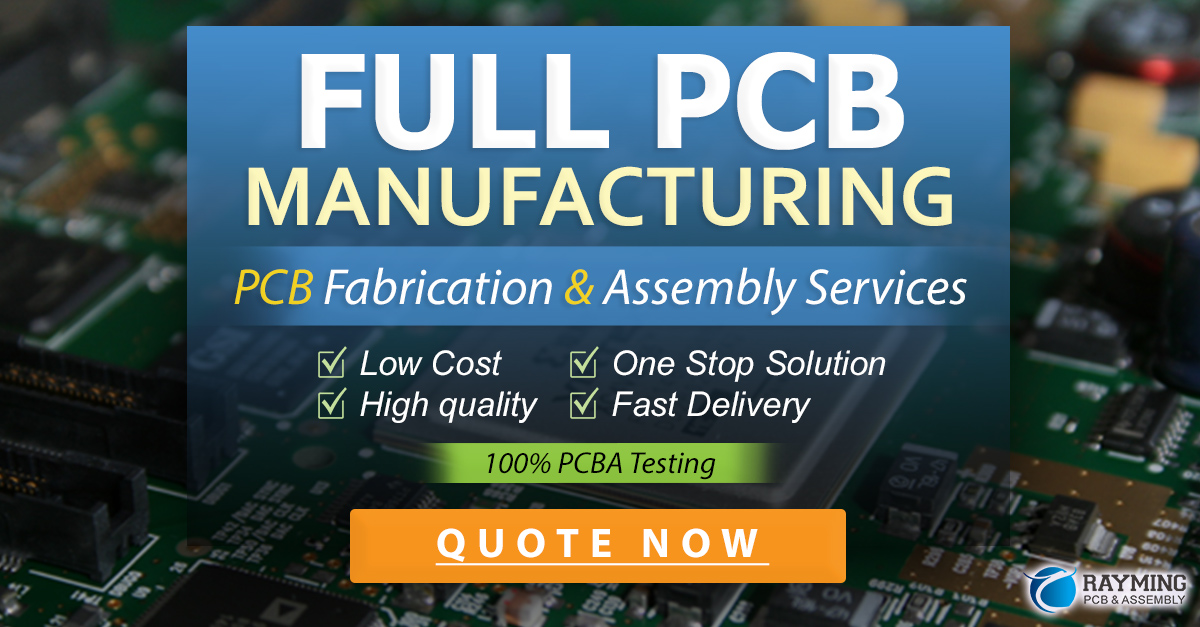
Interfacing with Other Hardware
OpenMV Cam can easily interface with other hardware, such as servos, motors, and displays, using its I/O pins. Here’s an example of controlling a servo using OpenMV Cam:
import sensor, image, time, pyb
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
servo = pyb.Servo(1)
while(True):
img = sensor.snapshot()
for blob in img.find_blobs([red_threshold], pixels_threshold=200, area_threshold=200, merge=True):
servo.angle(blob.cx() * 180 // img.width())
FAQs
-
What programming language does OpenMV Cam use?
OpenMV Cam uses Python as its primary programming language, making it easy for users to write and customize scripts. -
Can OpenMV Cam be used for real-time applications?
Yes, OpenMV Cam is capable of processing images in real-time, thanks to its powerful ARM Cortex-M7 processor and optimized image processing library. -
What is the maximum resolution supported by OpenMV Cam?
The Omnivision OV7725 image sensor used in OpenMV Cam supports a maximum resolution of 640×480 pixels (VGA). -
Can OpenMV Cam be used outdoors?
Yes, OpenMV Cam can be used outdoors, but it is recommended to use a protective case to shield the camera from dust, moisture, and other environmental factors. -
How do I update the firmware on my OpenMV Cam?
Firmware updates for OpenMV Cam can be easily installed using the OpenMV IDE. Simply connect your camera, select the appropriate firmware file, and click the “Load Firmware” button.
Conclusion
OpenMV Cam is a versatile and powerful tool for anyone interested in machine vision and embedded systems. With its combination of Python programming, high-resolution camera, and extensive image processing capabilities, OpenMV Cam opens up a world of possibilities for makers, hobbyists, and professionals alike. Whether you’re building a robot, designing a security system, or exploring the frontiers of computer vision, OpenMV Cam is an excellent choice for your next project.
Leave a Reply