Introduction to TM1637 Display Module
The TM1637 is a versatile and easy-to-use display module that allows you to create visually appealing projects with minimal effort. This four-digit, seven-segment LED display is commonly used in Arduino and other microcontroller projects to display numeric values, time, or custom patterns. In this comprehensive tutorial, we will cover everything you need to know to get started with the TM1637 display module.
What is the TM1637 Display Module?
The TM1637 is a microcontroller-driven, four-digit, seven-segment LED display module. It features a built-in controller that handles the multiplexing and driving of the LED segments, making it simple to interface with various microcontrollers like Arduino, Raspberry Pi, or ESP32.
Key Features of the TM1637 Display Module
- Four-digit, seven-segment LED display
- Built-in controller for easy interfacing
- Adjustable display brightness
- Low power consumption
- Simple two-wire communication protocol
- Compatible with various microcontrollers
Hardware Overview and Pinout
TM1637 Display Module Pinout
The TM1637 display module has four pins:
Pin | Name | Description |
---|---|---|
1 | CLK | Clock signal for synchronous communication |
2 | DIO | Bidirectional data input/output |
3 | VCC | Power supply (3.3V to 5V) |
4 | GND | Ground |
Connecting the TM1637 to an Arduino
To connect the TM1637 display module to an Arduino, follow these steps:
- Connect the VCC pin to the 5V or 3.3V pin on the Arduino, depending on your module’s specifications.
- Connect the GND pin to one of the GND pins on the Arduino.
- Choose two digital pins on the Arduino for the CLK and DIO signals, and connect them accordingly.
Example connection:
TM1637 Pin | Arduino Pin |
---|---|
CLK | D6 |
DIO | D7 |
VCC | 5V |
GND | GND |
Software Setup and Library Installation
Installing the TM1637 Library
To use the TM1637 display module with Arduino, you need to install the appropriate library. One of the most popular and well-maintained libraries is the “TM1637” library by Avishay Orpaz.
To install the library:
- Open the Arduino IDE.
- Navigate to Sketch > Include Library > Manage Libraries.
- Search for “TM1637” in the search bar.
- Locate the “TM1637” library by Avishay Orpaz and click on “Install.”
Including the Library in Your Sketch
Once the library is installed, you can include it in your Arduino sketch by adding the following line at the beginning of your code:
#include <TM1637Display.h>
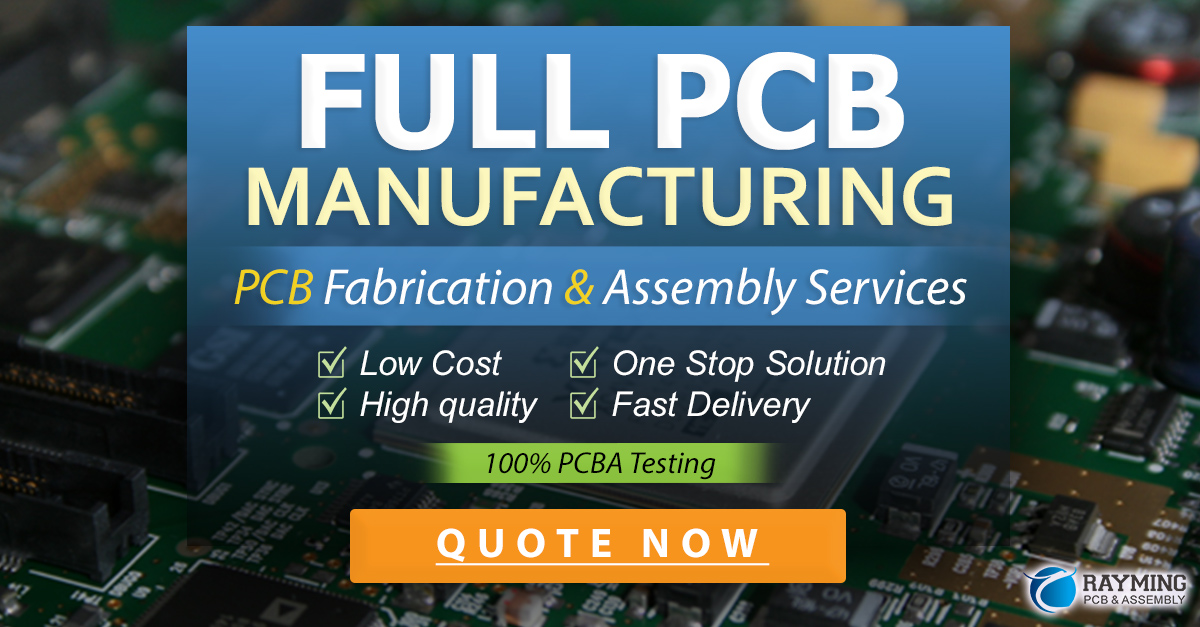
Basic Usage and Examples
Creating a TM1637 Display Object
To create a TM1637 display object, you need to specify the CLK and DIO pins you connected to the Arduino. Use the following code to create the object:
#define CLK 6
#define DIO 7
TM1637Display display(CLK, DIO);
Initializing the Display
In the setup()
function of your Arduino sketch, initialize the display by calling the setBrightness()
method. You can set the brightness level between 0 (lowest) and 7 (highest).
void setup() {
display.setBrightness(7);
}
Displaying Numbers
To display a number on the TM1637 display, use the showNumberDec()
method. This method takes two arguments: the number to be displayed and a boolean value indicating whether to show leading zeros.
void loop() {
display.showNumberDec(1234, false);
delay(1000);
display.showNumberDec(5678, true);
delay(1000);
}
Displaying Custom Segments
You can also display custom segments on the TM1637 display using the setSegments()
method. This method takes an array of four bytes, each representing the state of the segments for a single digit.
uint8_t custom[] = {
SEG_A | SEG_B | SEG_C | SEG_D | SEG_E | SEG_F, // Digit 1
SEG_A | SEG_B | SEG_F | SEG_G, // Digit 2
SEG_A | SEG_C | SEG_D | SEG_G, // Digit 3
SEG_B | SEG_C | SEG_D | SEG_E | SEG_G // Digit 4
};
void loop() {
display.setSegments(custom);
delay(1000);
}
Advanced Techniques and Projects
Displaying Time
One common application of the TM1637 display is to show the current time. You can use the Arduino’s built-in millis()
function to keep track of time and update the display accordingly.
unsigned long previousMillis = 0;
const long interval = 1000;
void loop() {
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval) {
previousMillis = currentMillis;
int hours = (currentMillis / 3600000) % 24;
int minutes = (currentMillis / 60000) % 60;
display.showNumberDecEx(hours * 100 + minutes, 0b01000000, true);
}
}
Creating a Countdown Timer
You can also use the TM1637 display to create a countdown timer. Set the initial time and update the display as the timer counts down.
const int startMinutes = 5;
int remainingSeconds = startMinutes * 60;
void loop() {
int minutes = remainingSeconds / 60;
int seconds = remainingSeconds % 60;
display.showNumberDecEx(minutes * 100 + seconds, 0b01000000, true);
if (remainingSeconds > 0) {
remainingSeconds--;
}
delay(1000);
}
Troubleshooting and FAQ
1. Q: My TM1637 display is not showing anything. What could be the problem?
A: Check your wiring connections and ensure that the CLK and DIO pins are connected correctly. Also, verify that you have set the appropriate brightness level using the setBrightness()
method.
2. Q: Can I use the TM1637 display with a 3.3V microcontroller?
A: Yes, most TM1637 display modules are compatible with both 3.3V and 5V logic levels. However, always check your specific module’s specifications to ensure compatibility.
3. Q: How can I display floating-point numbers on the TM1637 display?
A: The TM1637 library does not have built-in support for displaying floating-point numbers. However, you can convert the floating-point number to an integer and display it using the showNumberDec()
method.
4. Q: Is it possible to control multiple TM1637 displays with a single Arduino?
A: Yes, you can control multiple TM1637 displays by creating separate objects for each display and using different CLK and DIO pins for each one.
5. Q: Can I change the brightness of the TM1637 display dynamically?
A: Yes, you can call the setBrightness()
method anytime in your code to change the display brightness dynamically.
Conclusion
The TM1637 display module is a fantastic choice for adding a simple and intuitive display to your Arduino projects. With its built-in controller and easy-to-use library, you can quickly create visually appealing projects that display numbers, time, or custom patterns. By following this comprehensive tutorial, you should now have a solid understanding of how to set up and use the TM1637 display module in your own projects. Happy tinkering!
Leave a Reply