What is an RTC Module?
An RTC (Real-Time Clock) module is a small electronic device that keeps track of the current time and date, even when the main power source is off. It is an essential component in many projects that require accurate timekeeping, such as data logging, scheduling, and automation.
RTC modules typically use a battery backup to maintain the time and date information when the main power is disconnected. They communicate with microcontrollers or other devices using various protocols like I2C, SPI, or UART.
Common Features of RTC Modules
Feature | Description |
---|---|
Battery Backup | Maintains timekeeping when main power is off |
Accuracy | Typically within a few seconds per day |
Low Power Consumption | Ideal for battery-powered projects |
Multiple Communication Protocols | I2C, SPI, UART, etc. |
Calendar Functions | Keeps track of date, month, year, and day of the week |
Alarm Functions | Can trigger events at specific times or intervals |
Popular RTC Modules
There are several popular RTC modules available in the market, each with its own unique features and specifications. Some of the most commonly used RTC modules include:
1. DS1307
The DS1307 is a low-power, full binary-coded decimal (BCD) clock/calendar plus 56 bytes of NV SRAM. It communicates via an I2C interface and has a built-in power sense circuit that detects power failures and automatically switches to the backup supply.
2. DS3231
The DS3231 is a highly accurate I2C real-time clock with an integrated temperature-compensated crystal oscillator (TCXO) and crystal. It has a battery backup input and maintains seconds, minutes, hours, day, date, month, and year information, with leap-year compensation valid up to 2100.
3. PCF8563
The PCF8563 is a CMOS real-time clock/calendar optimized for low power consumption. It has a programmable clock output, an interrupt output, and a voltage-low detector. It communicates via an I2C interface and has a built-in oscillator.
Interfacing RTC Modules with Microcontrollers
Interfacing an RTC module with a microcontroller is relatively straightforward. Most RTC modules use the I2C communication protocol, which requires only two wires (SDA and SCL) for data transfer. Some modules may also use SPI or UART protocols.
I2C Communication
To interface an RTC module using I2C, follow these steps:
- Connect the VCC and GND pins of the RTC module to the appropriate power supply (usually 3.3V or 5V).
- Connect the SDA and SCL pins of the RTC module to the corresponding pins on the microcontroller.
- If required, connect the battery backup pin to a 3V coin cell battery.
- Use the appropriate libraries or functions in your microcontroller’s programming environment to initialize the I2C communication and set up the RTC module.
Here’s an example of how to interface a DS1307 RTC module with an Arduino using the RTClib
library:
#include <Wire.h>
#include "RTClib.h"
RTC_DS1307 rtc;
void setup() {
Serial.begin(9600);
rtc.begin();
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
void loop() {
DateTime now = rtc.now();
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(' ');
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.print(now.second(), DEC);
Serial.println();
delay(1000);
}
SPI Communication
Some RTC modules, like the DS1305, use the SPI communication protocol. To interface an SPI-based RTC module, follow these steps:
- Connect the VCC and GND pins of the RTC module to the appropriate power supply.
- Connect the MOSI, MISO, and SCK pins of the RTC module to the corresponding pins on the microcontroller.
- Connect the CS (Chip Select) pin of the RTC module to a digital pin on the microcontroller.
- If required, connect the battery backup pin to a 3V coin cell battery.
- Use the appropriate libraries or functions in your microcontroller’s programming environment to initialize the SPI communication and set up the RTC module.
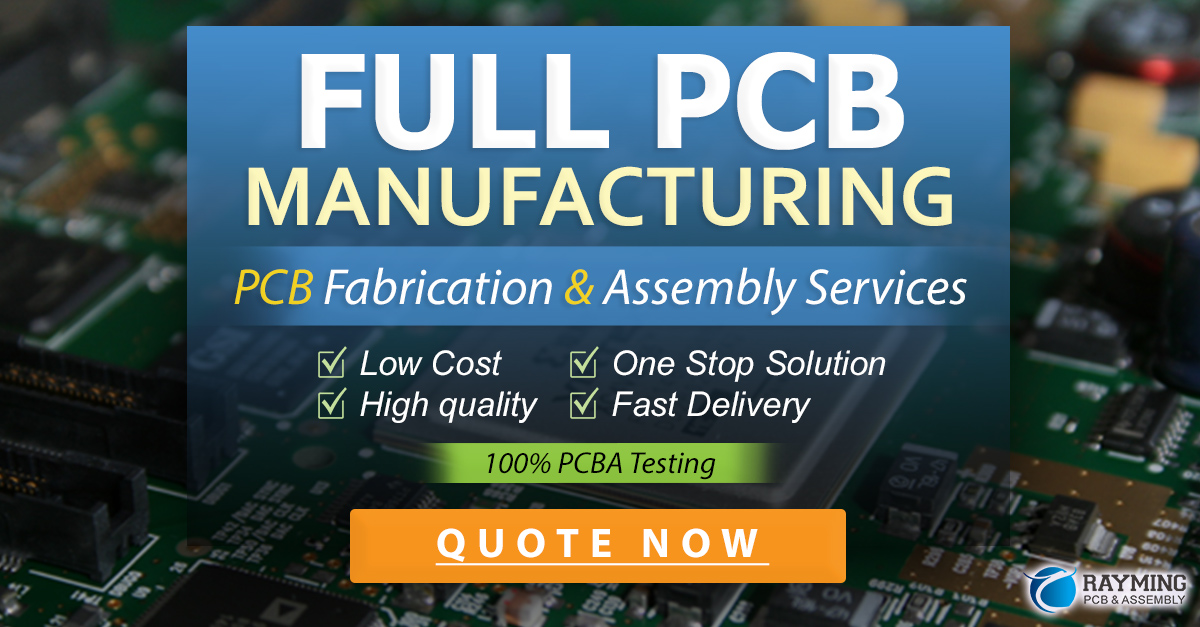
Applications of RTC Modules
RTC modules find applications in a wide range of projects, from simple clock displays to complex data logging systems. Some common applications include:
1. Clock and Calendar Displays
RTC modules can be used to create standalone clock and calendar displays or to add timekeeping functionality to existing projects. They can be combined with displays like LCDs, OLEDs, or 7-Segment Displays to show the current time and date.
2. Data Logging
In data logging applications, RTC modules are used to timestamp the collected data. This is particularly useful in projects that monitor environmental conditions, energy consumption, or other time-dependent variables.
3. Scheduling and Automation
RTC modules can be used to trigger events or actions at specific times or intervals. For example, they can be used to control irrigation systems, lighting, or other home automation tasks.
4. Low-Power Applications
Because of their low power consumption and battery backup feature, RTC modules are ideal for use in battery-powered projects. They can keep track of time even when the main power source is off, allowing the system to resume operation seamlessly when power is restored.
Troubleshooting RTC Modules
While RTC modules are generally reliable and easy to use, there may be instances where you encounter issues. Here are some common problems and their solutions:
1. Incorrect Time or Date
If your RTC module is displaying the wrong time or date, it may be due to one of the following reasons:
- The module was not initialized properly. Make sure you have set the correct time and date in your code during the initialization process.
- The battery backup is weak or not connected properly. Check the battery connection and replace the battery if necessary.
- There may be a problem with the crystal oscillator. If the module consistently loses time, it may indicate a faulty crystal or other hardware issues.
2. Communication Issues
If your microcontroller is unable to communicate with the RTC module, check the following:
- Ensure that the wiring between the microcontroller and the RTC module is correct, with the appropriate pins connected.
- Verify that you are using the correct communication protocol (I2C, SPI, or UART) and that the module is configured for the same protocol.
- Check if the module requires any pull-up resistors on the communication lines. Some modules have built-in pull-up resistors, while others may require external ones.
3. Power-related Problems
If your RTC module is not functioning properly due to power-related issues, consider the following:
- Make sure the module is receiving the correct voltage (usually 3.3V or 5V) from a stable power source.
- If using a battery backup, ensure that the battery is connected properly and has sufficient charge.
- Some modules may have a specific sequence for applying power. Consult the module’s datasheet for any required power-on procedures.
Frequently Asked Questions (FAQ)
-
Q: What is the difference between an RTC module and a regular clock?
A: An RTC module is a dedicated timekeeping device that maintains accurate time and date information even when the main power is off. It has a battery backup that allows it to continue functioning during power outages. A regular clock, on the other hand, is typically part of a larger system and does not have a backup power source. -
Q: Can I use an RTC module without a battery backup?
A: Yes, you can use an RTC module without a battery backup. However, in this case, the module will lose its time and date information whenever the main power is disconnected. The battery backup ensures that the module continues to keep track of time even during power outages. -
Q: How accurate are RTC modules?
A: The accuracy of RTC modules depends on several factors, including the quality of the crystal oscillator and the operating temperature. Most RTC modules are accurate to within a few seconds per day, with some high-end modules offering even better accuracy. -
Q: Can I use an RTC module with any microcontroller?
A: Yes, RTC modules can be used with a wide range of microcontrollers, including Arduino, Raspberry Pi, and other popular platforms. As long as your microcontroller has the appropriate communication interface (I2C, SPI, or UART) and you have the necessary libraries or functions, you can interface with an RTC module. -
Q: How long does the battery backup last in an RTC module?
A: The battery life depends on the specific module and the type of battery used. Most RTC modules use a CR2032 coin cell battery, which can last for several years under normal operating conditions. However, factors like temperature, humidity, and the frequency of power outages can affect battery life. It’s a good practice to replace the battery every few years to ensure reliable timekeeping.
Conclusion
RTC modules are versatile and essential components in many electronic projects that require accurate timekeeping. They offer a simple and reliable way to add real-time clock functionality to your projects, with features like battery backup, calendar functions, and alarm capabilities.
By following the guidelines and examples provided in this article, you should be able to easily interface an RTC module with your microcontroller and integrate it into your projects. Whether you’re building a clock display, a data logging system, or an automation solution, an RTC module can greatly enhance the functionality and reliability of your design.
Remember to choose the appropriate RTC module for your specific requirements, consider factors like accuracy, power consumption, and communication protocol. With the right module and proper implementation, you can create projects that keep precise time and operate seamlessly, even in the face of power disruptions.
Leave a Reply