Introduction to PID Temperature Controllers
A PID temperature controller is a critical component in many industrial processes where precise temperature control is essential. PID stands for Proportional-Integral-Derivative, which are the three terms used in the control algorithm. Temperature controllers using PID allow you to accurately regulate heating or cooling to maintain a target temperature setpoint.
PID controllers are used in a wide variety of applications including:
- Chemical processing
- Food and beverage manufacturing
- Plastics injection molding and extrusion
- Environmental chambers
- Laboratory and scientific equipment
- HVAC systems
The ability of PID controllers to minimize temperature deviations and respond quickly to disturbances makes them a popular choice for temperature-sensitive processes. In this article, we’ll dive into the fundamentals of PID control and walk through the steps of creating your own PID temperature controller.
How PID Temperature Control Works
Before we get into building a PID controller, it’s important to understand some key concepts behind how they function. The block diagram below shows the basic elements of a feedback control loop using a PID controller:
graph LR
A[Temperature Setpoint] --> B[Error]
C[PID Controller] --> D[Control Signal]
B --> C
D --> E[Process]
E --> F[Temperature Sensor]
F --> B
The key elements are:
- Temperature Setpoint: The desired temperature you want to maintain.
- Error: The difference between the setpoint and the actual measured temperature.
- PID Controller: Takes the error as an input and computes a control signal.
- Control Signal: The output from the PID controller used to drive a heating or cooling element.
- Process: The physical system being controlled, where heat transfer takes place.
- Temperature Sensor: Measures the actual temperature of the process and feeds it back to calculate the error.
The PID controller looks at the current value of the error, the integral of the error over time, and the current derivative of the error to determine its output. The “P” term provides an output proportional to the error. The “I” term sums error over time to correct steady-state errors. The “D” term looks at the current rate of change of the error to anticipate future errors.
The equation for the PID algorithm is:
u(t) = Kp * e(t) + Ki * ∫e(t)dt + Kd * de(t)/dt
Where:
– u(t) is the control signal
– e(t) is the error
– Kp is the proportional gain constant
– Ki is the integral gain constant
– Kd is the derivative gain constant
By tuning the values of Kp, Ki, and Kd, you can optimize the performance of the temperature control system to achieve the desired response in terms of rise time, overshoot, settling time, and steady-state error.
Hardware Components Needed
To build a PID temperature controller, you’ll need the following hardware components:
-
Microcontroller: Acts as the brain of the controller, running the PID algorithm. Popular options include Arduino, Raspberry Pi, or PIC microcontrollers.
-
Temperature Sensor: Measures the actual temperature of the system. Common types are thermocouples, RTDs (resistance temperature detectors), and thermistors.
-
Heating/Cooling Element: Provides the means to add or remove heat from the system. This could be a resistive heater, Peltier device, compressor, etc.
-
Solid State Relay (SSR): Allows the microcontroller to switch the high-power heating/cooling element.
-
Power Supply: Provides appropriate voltage and current for the microcontroller, sensor, and SSR.
-
LCD Display (optional): Useful for displaying the current temperature, setpoint, and PID parameters.
-
Buttons or Keypad (optional): Allows user input for changing setpoint and tuning parameters.
Here’s a table summarizing the key specs to look for when selecting these components:
Component | Key Specifications |
---|---|
Microcontroller | – Number of analog and digital I/O pins – PWM outputs – Sufficient program memory and RAM – Serial communication (UART, I2C, SPI) |
Temperature Sensor | – Suitable temperature range – Accuracy and precision – Response time – Output type (voltage, resistance, current) |
Heating/Cooling Element | – Power output – Voltage and current ratings – Efficiency – Form factor and size |
Solid State Relay | – Rated load voltage and current – Control voltage (compatible with microcontroller) – Switching time |
Power Supply | – Output voltage and current ratings – Voltage regulation – Protection features (short circuit, overload) |
LCD Display | – Display size and resolution – Communication interface (parallel, I2C, SPI) – Backlight type |
Buttons or Keypad | – Number of buttons – Debounce handling – Tactile feedback |
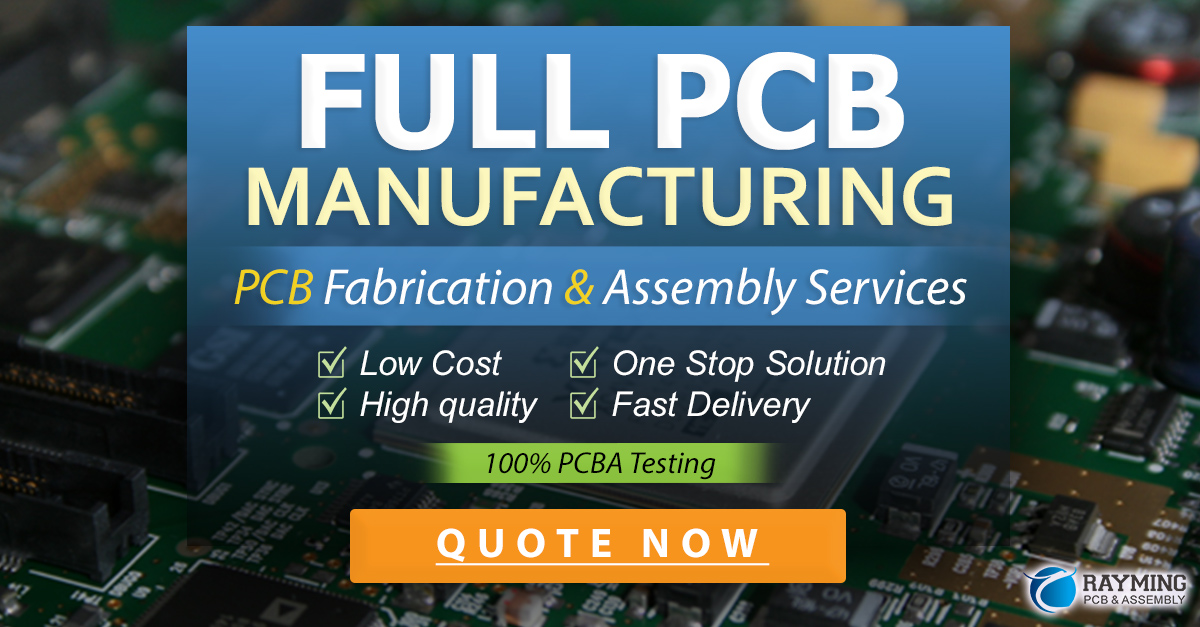
Wiring Diagram
Here’s a simplified wiring diagram showing how to connect the components for a PID temperature controller using an Arduino Uno:
Arduino Uno
-----------------
+5V <-- |VCC GND|--> GND
| |
Buttons ->|D2-D4 D13 |--> SSR Control
| |
Thermocouple ->|A0 D7|--> LCD RS
| D6|--> LCD Enable
| LCD D5|--> LCD D4
| ------ D4|--> LCD D5
| | | D3|--> LCD D6
| ------ D2|--> LCD D7
| |
GND <--|GND GND|--> GND
-----------------
PID Control Algorithm Implementation
Now let’s look at how to implement the PID algorithm in code. We’ll use the Arduino IDE and the C++ programming language for this example.
First, we need to include the necessary libraries and define some constants:
#include <LiquidCrystal.h>
#include <max6675.h>
// Pin definitions
#define THERMOCOUPLE_CS 10
#define THERMOCOUPLE_SO 9
#define THERMOCOUPLE_SCK 8
#define SSR_PIN 13
#define LCD_RS 7
#define LCD_EN 6
#define LCD_D4 5
#define LCD_D5 4
#define LCD_D6 3
#define LCD_D7 2
// PID constants
#define KP 10.0
#define KI 0.1
#define KD 1.0
// Other constants
#define SETPOINT 100.0 // Target temperature
#define SAMPLE_TIME 500 // PID update interval in ms
// Global variables
double input, output, setpoint;
double error, integral, derivative, last_error;
unsigned long last_time;
LiquidCrystal lcd(LCD_RS, LCD_EN, LCD_D4, LCD_D5, LCD_D6, LCD_D7);
MAX6675 thermocouple(THERMOCOUPLE_SCK, THERMOCOUPLE_CS, THERMOCOUPLE_SO);
Next, let’s initialize the PID variables and set the SSR control pin as an output in the setup()
function:
void setup() {
// Initialize LCD
lcd.begin(16, 2);
lcd.print("PID Temperature");
lcd.setCursor(0,1);
lcd.print("Controller");
// Initialize PID variables
setpoint = SETPOINT;
last_time = millis();
error = 0.0;
integral = 0.0;
derivative = 0.0;
last_error = 0.0;
// Set SSR control pin as output
pinMode(SSR_PIN, OUTPUT);
}
The main loop()
function will read the temperature sensor, calculate the PID output, and update the SSR and LCD:
void loop() {
// Read temperature input
input = thermocouple.readCelsius();
// Calculate PID output
unsigned long current_time = millis();
double dt = (double)(current_time - last_time) / 1000.0;
error = setpoint - input;
integral += error * dt;
derivative = (error - last_error) / dt;
output = KP * error + KI * integral + KD * derivative;
last_error = error;
last_time = current_time;
// Limit output to 0-100% duty cycle
output = constrain(output, 0, 100);
// Update SSR
digitalWrite(SSR_PIN, output > 50 ? HIGH : LOW);
// Update LCD
lcd.clear();
lcd.print("Temp: ");
lcd.print(input);
lcd.print(" C");
lcd.setCursor(0,1);
lcd.print("Output: ");
lcd.print(output);
lcd.print("%");
delay(SAMPLE_TIME);
}
The constrain()
function limits the PID output to a 0-100% range. This gets mapped to a simple on/off control signal for the SSR. For more advanced control, you could use PWM output to vary the SSR duty cycle.
PID Tuning
Once you have the basic PID temperature controller up and running, the next crucial step is tuning the PID constants Kp, Ki, and Kd to achieve optimal performance. Tuning is often an iterative process that requires some trial and error.
There are several methods for PID tuning, including:
-
Manual Tuning: Adjust Kp, Ki, and Kd based on observing the system response.
- Start with only Kp and increase until oscillations occur.
- Then increase Ki to eliminate steady-state error.
- Finally, increase Kd to dampen oscillations and improve response time.
-
Ziegler-Nichols Method: A classic PID tuning approach.
- Set Ki and Kd to zero.
- Increase Kp until sustained oscillations occur. Record this “ultimate gain” Ku and oscillation period Tu.
- Calculate PID constants based on Ku and Tu using the Ziegler-Nichols table.
-
Software-based Tuning: Use tuning software or PID auto-tuning libraries that can automatically determine optimal PID constants.
The table below shows typical effects of increasing each of the PID constants:
Parameter | Rise Time | Overshoot | Settling Time | Steady-State Error |
---|---|---|---|---|
Kp | Decrease | Increase | Small Change | Decrease |
Ki | Decrease | Increase | Increase | Eliminate |
Kd | Small Change | Decrease | Decrease | No Effect |
It’s important to note that PID tuning is highly dependent on the specific system dynamics, so these effects may vary. Always start with small increments when adjusting constants and monitor the system response closely.
Enhancements and Additional Features
There are many ways you can enhance your PID temperature controller for improved functionality and user experience. Some ideas include:
-
Autotuning: Implement a PID autotuning algorithm to automatically determine the optimal PID constants for your system.
-
Setpoint Ramping: Add the ability to gradually ramp the setpoint up or down to avoid sudden changes in temperature.
-
Alarms: Set up high and low temperature alarms to alert the user if the temperature exceeds set thresholds.
-
Data Logging: Log temperature data over time to an SD card or send it to a computer for analysis and visualization.
-
Remote Monitoring: Add wireless connectivity (e.g., Wi-Fi or Bluetooth) to monitor and control the temperature remotely from a smartphone or web interface.
-
Multiple Zones: Expand the controller to handle multiple temperature zones with independent PID control loops.
-
Adaptive Control: Implement adaptive PID control techniques that can automatically adjust PID constants based on changes in system behavior.
Troubleshooting Tips
If you encounter issues while building or operating your PID temperature controller, here are some troubleshooting tips:
-
Double-check wiring: Ensure all components are connected correctly and securely.
-
Verify sensor accuracy: Check that the temperature sensor is providing accurate readings. Use a reference thermometer to compare.
-
Monitor PID output: Watch the PID output to see if it’s behaving as expected. If it’s rapidly oscillating or hitting 0% or 100% limits, the PID constants may need adjusting.
-
Tune PID constants: If the temperature is oscillating or not reaching the setpoint, try adjusting the PID constants using the methods described earlier.
-
Check for noise: Electrical noise can interfere with sensor readings and PID calculations. Use shielded wires, ground properly, and add filtering capacitors if needed.
-
Verify code: Double-check your code for errors and ensure the PID algorithm is implemented correctly.
-
Adjust sample time: The PID sample time affects how frequently the controller updates. If it’s too short, the controller may become unstable. If too long, it may respond too slowly.
FAQ
-
What’s the difference between a PID controller and an on/off controller?
An on/off controller simply turns the heating/cooling element on or off when the temperature crosses the setpoint. This can lead to large temperature oscillations. A PID controller adjusts the power to the heating/cooling element proportionally based on the size and duration of the temperature error, resulting in much tighter temperature control.
-
Can I use a PID controller for both heating and cooling?
Yes, a PID controller can be used for both heating and cooling applications. You’ll need to have separate heating and cooling elements (e.g., a heater and a compressor) and control them with separate PID outputs. The PID output will be positive for heating and negative for cooling.
-
How do I choose the right PID constants?
The optimal PID constants (Kp, Ki, Kd) depend on the specific system dynamics, including the heat transfer characteristics, thermal mass, and disturbances. Start with a small Kp value and increment until oscillations occur. Then add Ki to eliminate steady-state error and Kd to improve response time. Fine-tune the constants through experimentation or use PID tuning methods like Ziegler-Nichols.
-
What if my system has a large time delay?
Systems with large time delays (e.g., a large oven or a process with slow-responding sensors) can be challenging for PID control. The derivative term (Kd) can amplify noise in these systems. One approach is to use a PI controller (omit the derivative term) and use a larger integral term (Ki) to compensate for the delay. Advanced techniques like Smith Predictors can also be used for time delay compensation.
-
Can I use PID control for non-temperature applications?
Yes, PID controllers are widely used for controlling various process variables beyond temperature, such as pressure, flow rate, speed, position, and more. The same principles of feedback control and PID tuning apply. You’ll need to select appropriate sensors and actuators for your specific application.
Conclusion
PID temperature controllers offer a powerful and flexible way to precisely regulate temperature in a wide range of applications. By understanding the basics of PID control and following the steps outlined in this article, you can build your own PID temperature controller using readily available hardware components and simple coding.
Remember that PID tuning is a critical step in achieving optimal performance. Start with the basic PID algorithm and then explore more advanced features like autotuning, adaptive control, and remote monitoring to take your temperature control to the next level.
With a well-designed PID temperature controller, you can ensure consistent, reliable temperature control for your projects and experiments. Happy building!
Leave a Reply