Introduction to Attiny84
The Attiny84 is an 8-bit AVR microcontroller that comes in a 14-pin package. It offers a good balance between size, performance, and power efficiency, making it suitable for a wide range of embedded applications. With its rich set of peripherals and low power modes, the Attiny84 is a go-to choice for projects that require a compact yet capable microcontroller.
Key Features of Attiny84
- 8KB of In-System Programmable Flash
- 512 bytes of EEPROM
- 512 bytes of SRAM
- 14 GPIO pins
- 8-bit Timer/Counter with Two PWM Channels
- 16-bit Timer/Counter with Two PWM Channels
- USI (Universal Serial Interface) for SPI and I2C Communication
- 10-bit ADC with 8 Channels
- Watchdog Timer
- Internal Oscillator
Attiny84 Pin Configuration
Understanding the pin configuration of the Attiny84 is crucial for designing circuits and interfacing with external components. Let’s take a closer look at the pinout and the functions of each pin.
Attiny84 Pinout
Pin | Function | Description |
---|---|---|
1 | VCC | Supply Voltage |
2 | PB0 (PCINT8/XTAL1) | GPIO / External Clock Input |
3 | PB1 (PCINT9/XTAL2) | GPIO / External Clock Output |
4 | PB3 (PCINT11/RESET) | GPIO / Reset Input |
5 | PB2 (PCINT10/INT0) | GPIO / External Interrupt 0 |
6 | PA7 (PCINT7/ADC7) | GPIO / ADC Input 7 |
7 | PA6 (PCINT6/ADC6) | GPIO / ADC Input 6 |
8 | PA5 (PCINT5/ADC5) | GPIO / ADC Input 5 |
9 | PA4 (PCINT4/ADC4) | GPIO / ADC Input 4 |
10 | PA3 (PCINT3/ADC3) | GPIO / ADC Input 3 |
11 | PA2 (PCINT2/ADC2) | GPIO / ADC Input 2 |
12 | PA1 (PCINT1/ADC1) | GPIO / ADC Input 1 |
13 | PA0 (PCINT0/ADC0) | GPIO / ADC Input 0 |
14 | GND | Ground |
Power Supply
The Attiny84 operates on a supply voltage range of 1.8V to 5.5V. The VCC pin (Pin 1) is used to provide power to the microcontroller, while the GND pin (Pin 14) serves as the ground reference.
GPIO Pins
The Attiny84 has 12 GPIO (General Purpose Input/Output) pins, which can be configured as either inputs or outputs. These pins are divided into two ports: Port A (PA0 to PA7) and Port B (PB0 to PB3). Each GPIO pin has multiple functions, such as:
- Digital input/output
- Analog-to-Digital Converter (ADC) input
- Pin Change Interrupt (PCINT)
- Timer/Counter functions
- USI (Universal Serial Interface) for SPI and I2C communication
Reset and Clock Pins
- Pin 4 (PB3) serves as the Reset input. Pulling this pin low will reset the microcontroller.
- Pin 2 (PB0) and Pin 3 (PB1) can be used as external clock input and output, respectively, if an external crystal or resonator is connected.
Programming the Attiny84
To start programming the Attiny84, you’ll need the following:
- An AVR programmer (e.g., AVR ISP, AVRISP mkII, USBasp)
- A development board or breadboard for prototyping
- The Attiny84 microcontroller
- A computer with the Arduino IDE or Atmel Studio installed
Setting Up the Development Environment
- If using the Arduino IDE:
- Install the Arduino IDE on your computer
- Add support for the Attiny84 by installing the “ATTinyCore” library through the Board Manager
-
Select the appropriate board (Attiny84) and programmer from the Tools menu
-
If using Atmel Studio:
- Install Atmel Studio on your computer
- Create a new project and select the Attiny84 as the target device
Connecting the Programmer
- Connect the AVR programmer to your computer using a USB cable
- Connect the programmer to the Attiny84 following the ISP (In-System Programming) pinout:
Programmer Pin | Attiny84 Pin |
---|---|
MISO | PA6 (Pin 7) |
MOSI | PA5 (Pin 8) |
SCK | PA4 (Pin 9) |
RESET | PB3 (Pin 4) |
VCC | VCC (Pin 1) |
GND | GND (Pin 14) |
- Ensure that the Attiny84 is powered either through the programmer or an external power supply
Writing and Uploading Code
- Open the Arduino IDE or Atmel Studio
- Write your code in the editor
- Compile the code to check for any errors
- Connect the programmer to the Attiny84 as described earlier
- Upload the code to the Attiny84 using the programmer
- Once the upload is complete, the Attiny84 will start executing the code
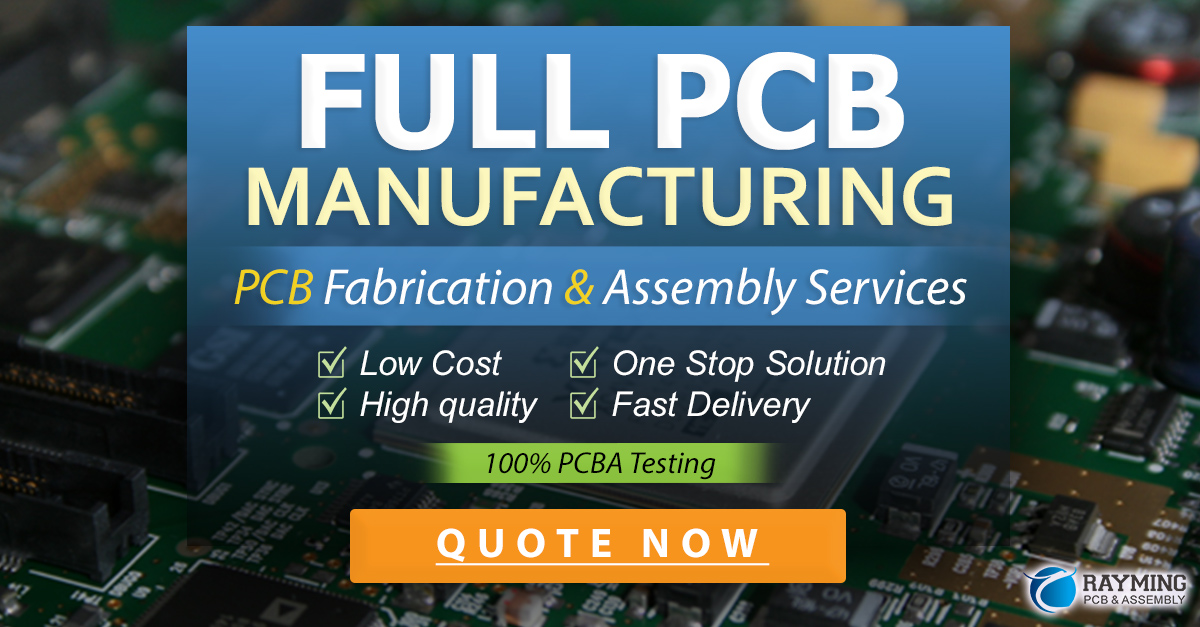
Example Code
Here’s a simple example code that blinks an LED connected to Pin 7 (PA6) of the Attiny84:
#include <avr/io.h>
#include <util/delay.h>
#define LED_PIN PA6
int main() {
// Set LED_PIN as output
DDRA |= (1 << LED_PIN);
while (1) {
// Turn on the LED
PORTA |= (1 << LED_PIN);
_delay_ms(1000);
// Turn off the LED
PORTA &= ~(1 << LED_PIN);
_delay_ms(1000);
}
return 0;
}
This code sets Pin 7 (PA6) as an output and repeatedly turns the LED on and off with a delay of 1 second.
Frequently Asked Questions (FAQ)
-
What is the difference between the Attiny84 and other AVR microcontrollers?
The Attiny84 is a compact, low-power microcontroller with a reduced set of features compared to larger AVR microcontrollers like the ATmega328P. It has fewer GPIO pins, smaller memory, and a limited set of peripherals, making it suitable for smaller, power-efficient projects. -
Can I program the Attiny84 using the Arduino IDE?
Yes, you can program the Attiny84 using the Arduino IDE by installing the “ATTinyCore” library, which adds support for various Attiny microcontrollers, including the Attiny84. This allows you to use the familiar Arduino programming environment and libraries. -
What is the maximum clock speed of the Attiny84?
The Attiny84 can operate at a maximum clock speed of 20MHz when powered with 5V. However, at lower voltages, the maximum clock speed is reduced. For example, at 3.3V, the maximum clock speed is 10MHz. -
How many analog input channels does the Attiny84 have?
The Attiny84 has 8 analog input channels (ADC0 to ADC7) that can be used to measure analog voltages. These channels are multiplexed with the GPIO pins PA0 to PA7. -
Can I use the Attiny84 for low-power applications?
Yes, the Attiny84 is well-suited for low-power applications. It offers various sleep modes and can operate at low voltages, which helps reduce power consumption. Additionally, the microcontroller has power-saving features like the Watchdog Timer and the ability to disable unused peripherals.
Conclusion
The Attiny84 is a versatile and compact microcontroller that offers a good balance of features and power efficiency. By understanding its pin configuration and programming steps, you can harness the power of the Attiny84 for your embedded projects. Whether you’re a beginner or an experienced developer, the Attiny84 provides a solid foundation for learning and exploration in the world of microcontrollers.
With its 8KB of flash memory, 512 bytes of EEPROM, and 512 bytes of SRAM, the Attiny84 is capable of handling a wide range of applications. Its 12 GPIO pins, 8-bit and 16-bit timers, USI interface, and ADC make it a flexible choice for projects involving sensors, actuators, and communication protocols.
By following the programming steps outlined in this article and referring to the Attiny84 Datasheet for detailed information, you can start developing your own projects using this powerful microcontroller. Whether you’re building a simple LED blinker or a complex sensor-based system, the Attiny84 provides a reliable and efficient platform for bringing your ideas to life.
So, grab your Attiny84, connect it to a programmer, and start exploring the exciting world of embedded development. With its extensive capabilities and user-friendly programming options, the Attiny84 is an excellent choice for both beginners and experienced developers alike.
Leave a Reply