What is a Component in React?
Before we delve into the specifics of Class Components and Functional Components, let’s define what a component is in the context of React.
A component in React is a reusable piece of code that represents a part of a user interface. It can be a button, a form, a list, or even a complete page. Components are the building blocks of a React application, allowing developers to break down a complex UI into smaller, manageable pieces.
Components are designed to be modular and independent, enabling them to be reused throughout an application. They encapsulate their own structure, styling, and behavior, making the codebase more maintainable and easier to understand.
Class Components
What is a Class Component?
A Class Component is a JavaScript class that extends the React.Component
base class. It is a more traditional way of defining components in React and has been widely used since the early versions of the library.
Here’s an example of a simple Class Component:
import React from 'react';
class MyComponent extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default MyComponent;
In this example, we define a class named MyComponent
that extends React.Component
. The component has a render()
method that returns the JSX to be rendered.
Characteristics of Class Components
- State: Class Components can maintain their own internal state using the
state
object. The state represents the data that can change over time and affects the component’s rendering. The initial state is defined in the constructor, and it can be updated using thesetState()
method.
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
incrementCount = () => {
this.setState((prevState) => ({
count: prevState.count + 1,
}));
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.incrementCount}>Increment</button>
</div>
);
}
}
- Lifecycle Methods: Class Components have access to lifecycle methods that allow you to hook into different stages of a component’s lifetime. Some commonly used lifecycle methods include:
componentDidMount()
: Invoked after the component is mounted to the DOM.componentDidUpdate()
: Invoked after the component’s state or props have been updated.componentWillUnmount()
: Invoked before the component is unmounted and destroyed.
class MyComponent extends React.Component {
componentDidMount() {
console.log('Component mounted');
}
componentDidUpdate(prevProps, prevState) {
console.log('Component updated');
}
componentWillUnmount() {
console.log('Component will unmount');
}
render() {
return <h1>Hello, World!</h1>;
}
}
- Event Handlers: Class Components can define event handlers as class methods. These methods can be bound to events such as clicks, form submissions, or keyboard events.
class MyComponent extends React.Component {
handleClick = () => {
console.log('Button clicked');
};
render() {
return <button onClick={this.handleClick}>Click Me</button>;
}
}
Advantages of Class Components
-
State Management: Class Components provide a built-in way to manage state using the
state
object and thesetState()
method. This makes it easier to handle dynamic data and update the component’s rendering based on state changes. -
Lifecycle Methods: Class Components have access to lifecycle methods that allow you to perform actions at specific points in a component’s lifecycle. This is useful for handling side effects, such as fetching data from an API or subscribing to events.
-
Code Organization: Class Components provide a clear and structured way to organize component logic. The class syntax allows for better code organization and separation of concerns.
Use Cases for Class Components
Class Components are suitable for the following scenarios:
-
Complex State Management: If a component needs to manage a significant amount of state or has complex state transitions, Class Components provide a more robust way to handle state management.
-
Lifecycle Methods: When a component requires specific actions to be performed at different stages of its lifecycle, such as fetching data or subscribing to events, Class Components offer the necessary lifecycle methods.
-
Reusable Logic: If a component contains reusable logic that needs to be shared across multiple components, Class Components can be a good choice. The class syntax allows for better code organization and encapsulation of component logic.
Functional Components
What is a Functional Component?
A Functional Component is a JavaScript function that takes props as an argument and returns JSX. It is a simpler and more concise way of defining components compared to Class Components.
Here’s an example of a simple Functional Component:
import React from 'react';
function MyComponent(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default MyComponent;
In this example, we define a function named MyComponent
that takes props
as an argument and returns the JSX to be rendered.
Characteristics of Functional Components
- Stateless: Traditionally, Functional Components were considered stateless, meaning they didn’t have their own internal state. They relied solely on the props passed to them to determine their rendering. However, with the introduction of React Hooks, Functional Components can now manage state using the
useState
hook.
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount((prevCount) => prevCount + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment</button>
</div>
);
}
- No Lifecycle Methods: Unlike Class Components, Functional Components do not have built-in lifecycle methods. However, with the introduction of React Hooks, Functional Components can now use the
useEffect
hook to perform side effects and mimic the behavior of lifecycle methods.
import React, { useEffect } from 'react';
function MyComponent() {
useEffect(() => {
console.log('Component mounted');
return () => {
console.log('Component will unmount');
};
}, []);
return <h1>Hello, World!</h1>;
}
- Simpler Syntax: Functional Components have a simpler and more concise syntax compared to Class Components. They are easier to read and understand, especially for developers who are familiar with JavaScript functions.
Advantages of Functional Components
-
Simplicity: Functional Components have a simpler syntax and are easier to read and understand compared to Class Components. They require less boilerplate code and are more straightforward to write.
-
Performance: Functional Components are lightweight and have a smaller footprint compared to Class Components. They don’t have the overhead of class instantiation and can result in slightly better performance.
-
Easier Testing: Functional Components are easier to test because they are pure functions that take props as input and return JSX. They don’t have internal state or lifecycle methods, making them more predictable and easier to test in isolation.
-
Code Reusability: Functional Components promote code reusability by allowing you to extract and share logic between components using custom hooks. This leads to a more modular and maintainable codebase.
Use Cases for Functional Components
Functional Components are suitable for the following scenarios:
-
Presentational Components: When a component’s primary responsibility is to render UI based on the props it receives, Functional Components are a good choice. They are lightweight and focused on presenting data.
-
Stateless Components: If a component doesn’t require internal state management or lifecycle methods, Functional Components are a simpler and more concise option.
-
Reusable UI Elements: Functional Components are ideal for creating reusable UI elements that can be easily shared across different parts of an application. They can be easily composed and combined to build more complex UI structures.
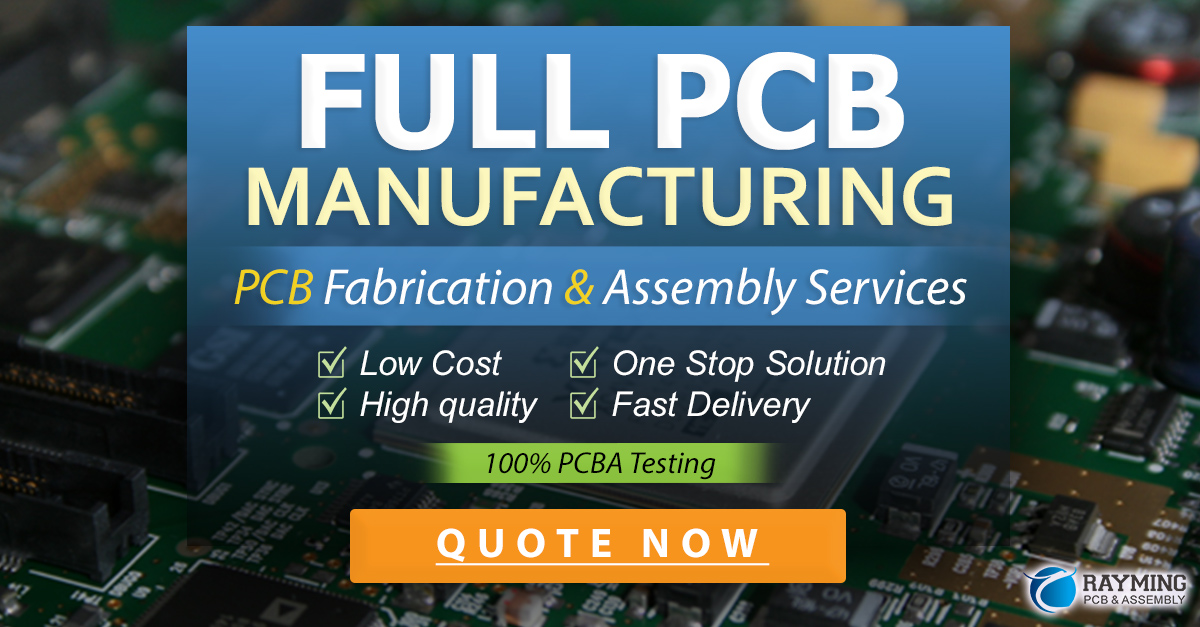
Comparison Table
Feature | Class Components | Functional Components |
---|---|---|
Syntax | Class syntax | Function syntax |
State Management | state object |
React Hooks (useState ) |
Lifecycle Methods | Available | React Hooks (useEffect ) |
Performance | Slightly slower | Slightly faster |
Code Reusability | Less intuitive | Easier with custom hooks |
Testing | More complex | Easier to test |
Frequently Asked Questions (FAQ)
-
Q: Can Class Components and Functional Components be used together in the same application?
A: Yes, absolutely! You can mix and match Class Components and Functional Components within the same React application. They can coexist and interact with each other seamlessly. -
Q: When should I choose a Class Component over a Functional Component?
A: If your component requires complex state management, lifecycle methods, or needs to leverage thethis
keyword, a Class Component might be a better choice. However, with the introduction of React Hooks, Functional Components can now handle most use cases that previously required Class Components. -
Q: Are Functional Components replacing Class Components?
A: While Functional Components have gained popularity and are now the preferred way of writing components in React, Class Components are still valid and have their place. Existing codebases may have Class Components, and there’s no need to rewrite them as Functional Components unless there’s a compelling reason to do so. -
Q: Can Functional Components have state?
A: Yes, with the introduction of React Hooks, specifically theuseState
hook, Functional Components can now manage their own state. TheuseState
hook allows you to add state to a Functional Component and update it as needed. -
Q: Are there any performance differences between Class Components and Functional Components?
A: Functional Components are generally considered slightly faster than Class Components due to their simpler structure and lack of class instantiation overhead. However, the performance difference is usually negligible in most cases, and the choice between Class Components and Functional Components should be based on the specific requirements of your component.
Conclusion
In summary, Class Components and Functional Components are two different approaches to defining components in React. Class Components are based on JavaScript classes and provide a more traditional way of managing state and lifecycle methods. On the other hand, Functional Components are simpler, more concise, and rely on React Hooks for state management and side effects.
When deciding between Class Components and Functional Components, consider factors such as the complexity of state management, the need for lifecycle methods, code reusability, and personal preference. With the introduction of React Hooks, Functional Components have become more powerful and can handle most use cases previously reserved for Class Components.
Regardless of the component type you choose, the key is to write clean, maintainable, and reusable code that follows best practices and aligns with the needs of your application. Both Class Components and Functional Components have their strengths and can be used effectively to build robust and scalable React applications.
Leave a Reply