Introduction to CAN-Bus and CANBed
CAN (Controller Area Network) is a robust vehicle bus standard designed to allow microcontrollers and devices to communicate with each other’s applications without a host computer. It is a message-based protocol, designed originally for multiplex electrical wiring within automobiles to save on copper, but can also be used in many other contexts.
CANBed is an Arduino-based development kit that simplifies the process of working with CAN-Bus. It provides an easy-to-use platform for developers to create, test, and deploy CAN-Bus applications quickly and efficiently.
Features of CANBed
Hardware Specifications
The CANBed development kit includes the following hardware components:
- Arduino-compatible microcontroller board
- CAN-Bus shield with MCP2515 CAN controller and TJA1050 CAN transceiver
- DB9 connector for CAN-Bus communication
- Screw terminals for power supply and CAN-High/CAN-Low signals
- LED indicators for power, TX, and RX
- Reset button
Component | Specification |
---|---|
Microcontroller | ATmega328P (Arduino Uno compatible) |
CAN Controller | MCP2515 with SPI interface |
CAN Transceiver | TJA1050 |
CAN Connector | DB9 male |
Power Supply | 7-12V DC input, 5V DC output (regulated) |
Dimensions | 68mm x 53mm x 25mm |
Software Libraries
CANBed comes with a set of Arduino libraries that make it easy to work with CAN-Bus:
CANBed.h
: The main library that provides high-level functions for initializing, sending, and receiving CAN messages.MCP2515.h
: A library for interfacing with the MCP2515 CAN controller.CAN.h
: A standard Arduino CAN library that provides a unified interface for working with different CAN controllers.
Getting Started with CANBed
Setting Up the Hardware
- Connect the CAN-Bus shield to the Arduino board.
- Connect the DB9 connector to your CAN-Bus network.
- Supply power to the CANBed board using the screw terminals or the DC barrel jack.
Installing the Software
- Download and install the Arduino IDE from the official website.
- Clone or download the CANBed repository from GitHub.
- Open the Arduino IDE and navigate to
Sketch > Include Library > Add .ZIP Library
. - Select the downloaded CANBed repository ZIP file.
Example Sketch: Sending CAN Messages
Here’s a simple example sketch that demonstrates how to send CAN messages using CANBed:
#include <CANBed.h>
void setup() {
CANBed.begin(500E3); // Initialize CAN-Bus at 500 kbps
}
void loop() {
CANMessage msg;
msg.id = 0x123;
msg.len = 8;
msg.data[0] = 0x01;
msg.data[1] = 0x23;
msg.data[2] = 0x45;
msg.data[3] = 0x67;
msg.data[4] = 0x89;
msg.data[5] = 0xAB;
msg.data[6] = 0xCD;
msg.data[7] = 0xEF;
CANBed.send(msg);
delay(1000);
}
This sketch initializes the CAN-Bus at a baud rate of 500 kbps, creates a CAN message with ID 0x123
and 8 bytes of data, sends the message, and repeats every 1 second.
Example Sketch: Receiving CAN Messages
Here’s another example sketch that demonstrates how to receive CAN messages using CANBed:
#include <CANBed.h>
void setup() {
CANBed.begin(500E3); // Initialize CAN-Bus at 500 kbps
Serial.begin(9600); // Initialize serial communication for debugging
}
void loop() {
CANMessage msg;
if (CANBed.receive(msg)) {
Serial.print("ID: ");
Serial.print(msg.id, HEX);
Serial.print(" Data: ");
for (int i = 0; i < msg.len; i++) {
Serial.print(msg.data[i], HEX);
Serial.print(" ");
}
Serial.println();
}
}
This sketch initializes the CAN-Bus at a baud rate of 500 kbps and the serial communication at 9600 bps. It then enters a loop where it waits for incoming CAN messages. When a message is received, it prints the message ID and data to the serial console.
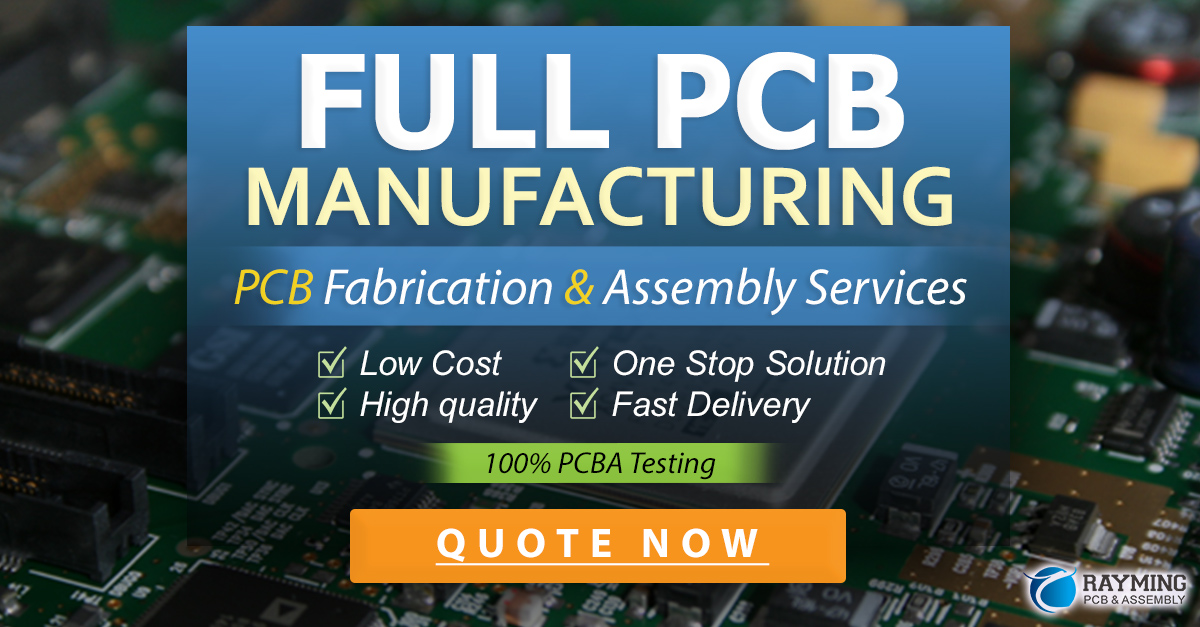
Advanced Features of CANBed
Filtering CAN Messages
CANBed allows you to filter incoming CAN messages based on their IDs. This can be useful when you only want to receive messages with specific IDs. Here’s an example of how to set up message filtering:
#include <CANBed.h>
void setup() {
CANBed.begin(500E3); // Initialize CAN-Bus at 500 kbps
CANBed.setFilter(0x123, 0x7FF); // Filter messages with ID 0x123
}
void loop() {
CANMessage msg;
if (CANBed.receive(msg)) {
// Process the received message
}
}
In this example, the setFilter
function is used to configure the CAN controller to only accept messages with ID 0x123
. The second argument (0x7FF
) is a mask that specifies which bits of the ID should be compared.
Using Extended CAN IDs
By default, CANBed uses standard 11-bit CAN IDs. However, it also supports extended 29-bit IDs. To use extended IDs, you need to modify the CANMessage
structure and set the appropriate flag. Here’s an example:
#include <CANBed.h>
void setup() {
CANBed.begin(500E3); // Initialize CAN-Bus at 500 kbps
}
void loop() {
CANMessage msg;
msg.id = 0x12345678 | CAN_EFF_FLAG; // Set extended ID
msg.len = 8;
// Set message data
CANBed.send(msg);
delay(1000);
}
In this example, the CAN_EFF_FLAG
is used to indicate that the ID is an extended 29-bit ID.
Handling CAN Bus Errors
CAN-Bus is designed to be fault-tolerant, but errors can still occur. CANBed provides functions for handling CAN bus errors. Here’s an example:
#include <CANBed.h>
void setup() {
CANBed.begin(500E3); // Initialize CAN-Bus at 500 kbps
}
void loop() {
if (CANBed.checkError()) {
Serial.println("CAN bus error detected!");
CANBed.reset(); // Reset the CAN controller
}
// Rest of the code
}
In this example, the checkError
function is used to check for CAN bus errors. If an error is detected, a message is printed to the serial console and the reset
function is called to reset the CAN controller.
FAQ
What is the maximum baud rate supported by CANBed?
CANBed supports baud rates up to 1 Mbps. However, the actual maximum baud rate may be limited by the length and characteristics of the CAN bus cable.
Can I use CANBed with other Arduino boards?
Yes, CANBed is compatible with most Arduino boards that have an SPI interface. However, you may need to modify the pin mappings in the CANBed.h
library to match your specific board.
How many CAN nodes can be connected to a single CANBed?
The maximum number of CAN nodes that can be connected to a single CANBed depends on the CAN bus topology and termination. In general, CAN bus allows up to 120 nodes per segment.
What is the maximum length of a CAN bus cable?
The maximum length of a CAN bus cable depends on the baud rate and cable characteristics. At a baud rate of 1 Mbps, the maximum cable length is typically around 40 meters. Lower baud rates allow for longer cable lengths.
Can CANBed be used in harsh environments?
CANBed is designed for prototyping and development purposes. For use in harsh environments, you may need to consider factors such as temperature range, vibration, and electromagnetic interference. Industrial-grade CAN transceivers and proper shielding may be required in such cases.
Conclusion
CANBed is a powerful and easy-to-use development kit for working with CAN-Bus on Arduino. With its comprehensive hardware and software features, CANBed simplifies the process of creating, testing, and deploying CAN-Bus applications. Whether you’re a beginner or an experienced developer, CANBed provides a solid foundation for exploring the world of CAN-Bus communication.
Leave a Reply